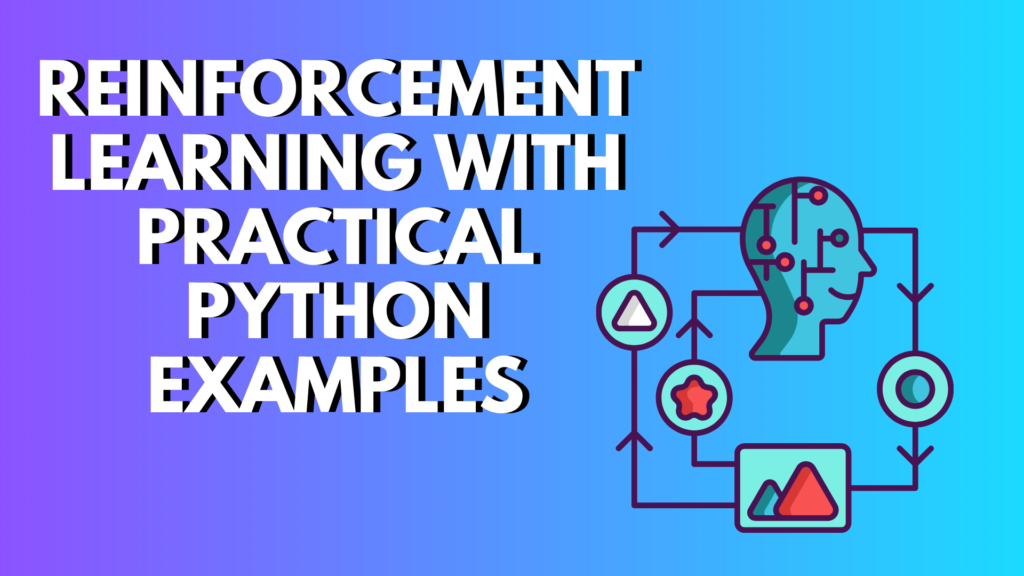
Introduction
Reinforcement Learning (RL) is an exciting field in artificial intelligence that allows machines to learn optimal decision-making strategies by interacting with their environment. Unlike supervised learning, RL does not require labeled data; instead, it relies on rewards and punishments to guide learning. This approach has been used in game-playing AI (like AlphaGo), robotics, and even finance.
Can you do reinforcement learning in Python? Yes! Python is one of the best programming languages for RL, with libraries like TensorFlow, PyTorch, and OpenAI Gym making implementation easy.
In this article, we will break down reinforcement learning concepts, explore key algorithms, and implement a simple RL model in Python using OpenAI’s Gym and Q-learning.
Reinforcement learning with practical python examples github
Free reinforcement learning with practical python examples
Reinforcement learning Python sklearn
Reinforcement learning Python code github
Reinforcement learning example
Reinforcement learning Python library
Reinforcement learning Python book
Reinforcement learning Python PyTorch
What is Reinforcement Learning?
Reinforcement Learning is a type of machine learning where an agent learns by interacting with an environment. The goal is to take actions that maximize cumulative rewards over time.
Key Concepts in RL
- Agent: The learner or decision-maker (e.g., a robot, game AI, or trading algorithm).
- Environment: The world in which the agent operates.
- State (S): A representation of the current situation of the agent.
- Action (A): The set of possible moves the agent can take.
- Reward (R): A feedback signal that tells the agent how good or bad an action is.
- Policy (π): A strategy that the agent follows to determine actions.
- Value Function (V): Estimates how good a given state is for achieving maximum reward.
- Q-Value (Q): Measures the value of taking a particular action in a given state.
Which principle is essential to reinforcement learning? The most essential principle in RL is the reward hypothesis, which states that an agent’s objective is to maximize cumulative rewards over time.
Is Reinforcement Learning AI or ML?
Reinforcement learning is a subset of machine learning (ML) and a key component of artificial intelligence (AI). It falls under the broader AI umbrella, focusing on learning through interactions.
Types of Reinforcement Learning
1. Model-Free vs. Model-Based RL
- Model-Free RL: The agent learns without knowledge of the environment’s dynamics (e.g., Q-learning, Deep Q Networks).
- Model-Based RL: The agent builds a model of the environment and uses it to plan (e.g., AlphaZero).
2. On-Policy vs. Off-Policy Learning
- On-Policy: The agent learns from actions generated by its current policy (e.g., SARSA).
- Off-Policy: The agent learns from past experiences or actions generated by another policy (e.g., Q-learning).
Implementing Q-Learning in Python
We will now implement a simple Q-learning algorithm to train an agent to navigate the FrozenLake environment from OpenAI Gym.
Step 1: Install Dependencies
pip install numpy gym
Step 2: Import Libraries
import gym
import numpy as np
import random
Step 3: Initialize the Environment and Q-Table
env = gym.make("FrozenLake-v1", is_slippery=False)
state_size = env.observation_space.n
action_size = env.action_space.n
q_table = np.zeros((state_size, action_size))
Step 4: Define Hyperparameters
learning_rate = 0.1
discount_factor = 0.99
epsilon = 1.0
epsilon_decay = 0.995
epsilon_min = 0.01
episodes = 1000
Step 5: Implement Q-Learning Algorithm
for episode in range(episodes):
state = env.reset()[0]
done = False
while not done:
if random.uniform(0, 1) < epsilon:
action = env.action_space.sample() # Explore
else:
action = np.argmax(q_table[state, :]) # Exploit best action
next_state, reward, done, _, _ = env.step(action)
q_table[state, action] = q_table[state, action] + learning_rate * \
(reward + discount_factor * np.max(q_table[next_state, :]) - q_table[state, action])
state = next_state
epsilon = max(epsilon * epsilon_decay, epsilon_min) # Decay epsilon
Step 6: Test the Trained Agent
total_rewards = []
for episode in range(100):
state = env.reset()[0]
done = False
total_reward = 0
while not done:
action = np.argmax(q_table[state, :])
next_state, reward, done, _, _ = env.step(action)
total_reward += reward
state = next_state
total_rewards.append(total_reward)
print("Average Reward:", np.mean(total_rewards))
Conclusion
Reinforcement learning is a powerful technique that enables machines to make intelligent decisions through trial and error. In this guide, we explored the fundamental concepts of RL and implemented a basic Q-learning algorithm in Python.
If you’re interested in diving deeper, consider exploring Deep Q Networks (DQN), Policy Gradient Methods, or Multi-Agent Reinforcement Learning.
What is a practical example of reinforcement learning?
Can you do reinforcement learning in Python?
What is a real-time example of reinforcement learning?
What is an example of a game with reinforcement learning?
Frequently Asked Questions (FAQ)
Is ChatGPT reinforcement learning? ChatGPT is trained using a combination of supervised learning and reinforcement learning from human feedback (RLHF) to fine-tune responses.
Is TensorFlow good for reinforcement learning? Yes, TensorFlow is widely used in reinforcement learning, especially for implementing deep RL models like Deep Q Networks (DQN).
Is PyTorch or TensorFlow better for reinforcement learning? Both are excellent, but PyTorch is often preferred for flexibility and ease of debugging, while TensorFlow is favored for production scalability.
How do I train my own AI in Python? You can train your AI using Python libraries like TensorFlow, PyTorch, and OpenAI Gym by implementing RL algorithms like Q-learning or Policy Gradient Methods.
What is the best algorithm for reinforcement learning? The best algorithm depends on the task. Q-learning, Deep Q Networks (DQN), and Proximal Policy Optimization (PPO) are popular choices.
Can PyTorch do reinforcement learning? Yes, PyTorch is a powerful framework for reinforcement learning and is widely used in deep RL research and development.
Call to Action
Did you find this guide helpful? Share it with others and start experimenting with reinforcement learning today! Explore more advanced RL techniques and real-world applications to take your skills to the next level.
0 Comments