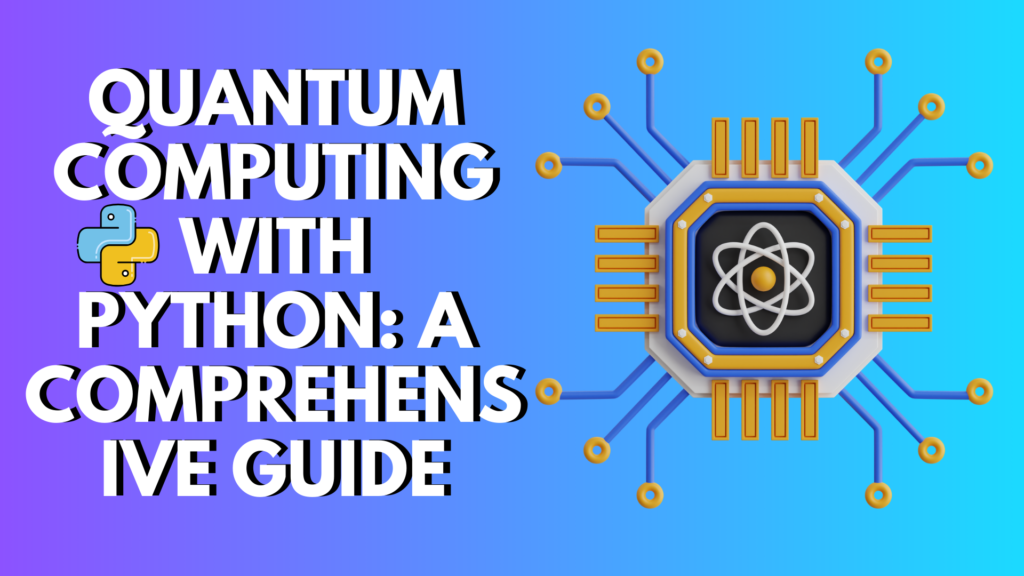
Introduction
Quantum computing is one of the most revolutionary advancements in technology, promising to transform industries by solving complex problems exponentially faster than classical computers. But how can you get started with quantum computing using Python?
In this guide, we’ll explore how quantum computing works, why it matters, and how you can use Python to write and execute quantum algorithms. Whether you’re a beginner or an experienced programmer, this post will provide actionable insights to help you navigate the quantum world.
What is Quantum Computing?
Quantum computing leverages the principles of quantum mechanics—such as superposition and entanglement—to perform computations in a way that classical computers cannot. Unlike traditional bits (0s and 1s), quantum bits (qubits) can exist in multiple states simultaneously, enabling faster processing and problem-solving.
Key Concepts:
- Qubits: The fundamental unit of quantum information, analogous to classical bits but with unique properties.
- Superposition: A qubit can exist in multiple states at once.
- Entanglement: Qubits can be interconnected, affecting each other regardless of distance.
- Quantum Gates: Operations that manipulate qubits, similar to logic gates in classical computing.
Why is Quantum Computing Important?
Quantum computing is set to revolutionize various fields, including:
- Cryptography: Breaking traditional encryption methods and enabling quantum-safe security.
- Artificial Intelligence & Machine Learning: Enhancing optimization and data analysis capabilities.
- Drug Discovery: Simulating molecular interactions for faster drug development.
- Financial Modeling: Improving risk analysis and trading strategies.
- Climate Modeling: Simulating complex environmental changes with high precision.
Getting Started with Quantum Computing in Python
Python has become the go-to language for quantum computing, thanks to its simplicity and the availability of powerful libraries. Here are some key tools:
1. Qiskit (IBM Quantum)
Qiskit is an open-source framework developed by IBM for quantum computing.
Installation:
pip install qiskit
Example: Creating a Quantum Circuit
from qiskit import QuantumCircuit, Aer, transpile, assemble, execute
# Create a quantum circuit with 2 qubits
qc = QuantumCircuit(2)
qc.h(0) # Apply Hadamard gate to first qubit
qc.cx(0, 1) # Apply CNOT gate (entanglement)
qc.measure_all()
# Simulate the quantum circuit
simulator = Aer.get_backend('aer_simulator')
compiled_circuit = transpile(qc, simulator)
qobj = assemble(compiled_circuit)
result = simulator.run(qobj).result()
print(result.get_counts())
2. Cirq (Google Quantum)
Cirq is a Python library developed by Google for designing and simulating quantum circuits.
Installation:
pip install cirq
Example: Creating a Bell State
import cirq
q0, q1 = cirq.LineQubit.range(2)
circuit = cirq.Circuit(
cirq.H(q0),
cirq.CNOT(q0, q1),
cirq.measure(q0, q1)
)
simulator = cirq.Simulator()
result = simulator.run(circuit, repetitions=10)
print(result)
3. PennyLane (Quantum Machine Learning)
PennyLane bridges quantum computing and machine learning.
Installation:
pip install pennylane
Example: Variational Quantum Circuit
import pennylane as qml
from pennylane import numpy as np
n_qubits = 2
dev = qml.device("default.qubit", wires=n_qubits)
@qml.qnode(dev)
def circuit(params):
qml.RX(params[0], wires=0)
qml.RY(params[1], wires=1)
return qml.expval(qml.PauliZ(0))
params = np.array([0.1, 0.5], requires_grad=True)
print(circuit(params))
How Quantum Computing Will Change the Future
1. Quantum Computing in AI
Quantum algorithms like Quantum Neural Networks (QNNs) could revolutionize machine learning by dramatically reducing training times and improving model accuracy.
2. Impact on Cryptography
Quantum computers pose a threat to current encryption systems (e.g., RSA, AES). This has led to the development of Post-Quantum Cryptography (PQC) to create secure encryption methods resistant to quantum attacks.
3. Advancements in Drug Discovery
Quantum computing allows researchers to model molecular structures with unprecedented accuracy, speeding up drug development.
4. Financial Markets and Optimization
Quantum algorithms can solve complex optimization problems in finance, logistics, and risk assessment more efficiently than classical systems.
Challenges and Limitations of Quantum Computing
Despite its potential, quantum computing still faces challenges:
- Hardware Limitations: Qubits are fragile and require extreme cooling to function properly.
- Error Rates: Quantum operations are prone to errors due to noise and decoherence.
- Scalability Issues: Current quantum systems have a limited number of qubits, restricting their computational power.
- High Costs: Quantum computing hardware and cloud access remain expensive.
FAQ
Can Python be used for quantum computing?
Yes! Python is widely used in quantum computing thanks to libraries like Qiskit, Cirq, and PennyLane, which provide tools to write and run quantum algorithms.
Which programming language is best for quantum computing?
Python is currently the most popular language for quantum computing, but other languages like C++ and Julia are also used in quantum research.
Will quantum computing replace AI?
No, quantum computing will enhance AI rather than replace it. Quantum machine learning could lead to faster training and better optimization in AI models.
What is the next big tech after AI?
Quantum computing is considered one of the most transformative technologies following AI, along with advancements in biotechnology and brain-computer interfaces.
Is quantum programming the future?
Yes, as quantum hardware improves, quantum programming will play a crucial role in solving problems beyond classical computing capabilities.
What is the biggest problem with quantum computing?
The biggest challenge is error correction due to qubit instability and noise, making it difficult to achieve reliable computations.
How long until quantum computers?
Experts estimate that practical quantum computers could become mainstream within 10-20 years, but significant advancements are expected sooner.
Who is the leader in quantum computing?
IBM, Google, Microsoft, and startups like Rigetti and IonQ are leading the quantum computing race.
Conclusion
Quantum computing with Python is more accessible than ever, thanks to libraries like Qiskit, Cirq, and PennyLane. Whether you’re interested in cryptography, AI, or scientific research, Python provides powerful tools to explore this emerging field.
🔹 Ready to dive deeper? Explore official documentation and try running quantum circuits on IBM’s cloud-based quantum computers! 🚀
0 Comments