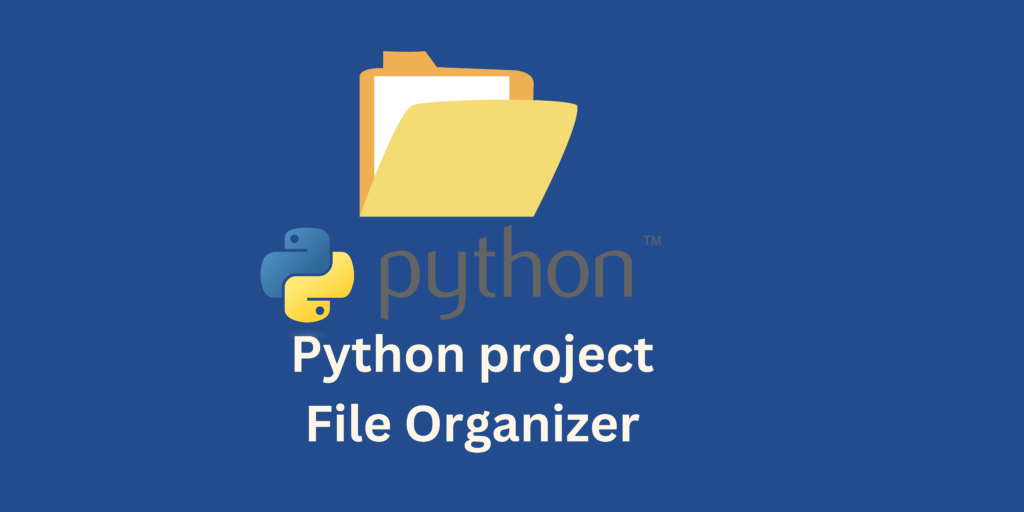
A Python project called the File Organizer project automatically arranges files in a directory according to the type of file they are.
Files are moved to different folders based on their file extensions after the program scans the specified directory. All image files, for instance, would be transferred to a folder called images, and all text files, to a folder called documents.
Steps for python project
Importing Modules
First, we import the required modules – os
, shutil
, and sys
.
import os
import shutil
import sys
Defining Functions
Next, we define two functions – create_dir
and move_file
.
def create_dir(directory):
if not os.path.exists(directory):
os.makedirs(directory)
def move_file(file_path, destination_directory):
shutil.move(file_path, destination_directory)
The create_dir
function checks if a directory exists and creates it if it doesn’t. The move_file
function moves a file to a specified destination directory.
File Organizer
In the main function of the program, we first define the directory to organize and create the necessary subdirectories using the create_dir
function.
Here, we define the directory to organize and create subdirectories for different file types.
def main():
directory = "C:/Users/user/Desktop/files"
create_dir(directory + "/images")
create_dir(directory + "/documents")
create_dir(directory + "/music")
create_dir(directory + "/videos")
create_dir(directory + "/archives")
Next, we iterate over all files in the directory using the os.scandir
method and move them to the appropriate subdirectory based on their file extension.
def main():
directory = "C:/Users/user/Desktop/files"
# create subdirectories here
for file in os.scandir(directory):
if file.is_file():
file_path = file.path
file_extension = os.path.splitext(file_path)[1]
if file_extension in (".png", ".jpg", ".jpeg", ".gif"):
move_file(file_path, directory + "/images")
elif file_extension in (".txt", ".docx", ".pdf"):
move_file(file_path, directory + "/documents")
elif file_extension in (".mp3", ".wav", ".ogg"):
move_file(file_path, directory + "/music")
elif file_extension in (".mp4", ".avi", ".mkv"):
move_file(file_path, directory + "/videos")
elif file_extension in (".zip", ".rar", ".tar"):
move_file(file_path, directory + "/archives")
Here, we use the os.path.splitext
method to get the file extension of each file and move it to the appropriate subdirectory based on the extension.
Run the File Organizer
- Copy the entire code into a new file and save it with a
.py
extension (e.g.,file_organizer.py
). - Modify the
directory
variable in themain()
function to the directory you want to organize. - Open a terminal or command prompt and navigate to the directory where the Python file is saved.
- Run the Python file by typing
python file_organizer.py
and pressing enter.
Conclusion of python project
In conclusion, the File Organizer project in Python demonstrates how to automatically organize files in a directory based on their file type.
This project can be further improved by adding support for additional file types, implementing error handling, and adding a GUI for a more user-friendly experience.
0 Comments