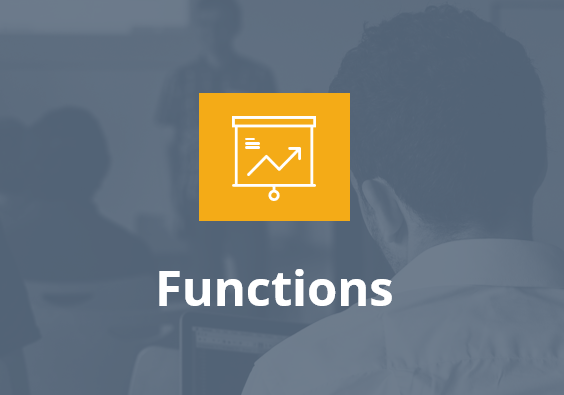
functions course, this is the simplest python functions course ever.
This course will teach you the fundamentals of Python functions as well as how to write them. By the conclusion of the training, you would be capable of developing simple functions to computerize tasks and improve the efficiency of your code.
What is a function?
A function is a block of code which only runs when it is called.
You can pass data, known as parameters, into a function.
A function can return data as a result.
Creating a Function
In Python a function is defined using the def keyword:
def function_name():
print("Hello from a function")
Calling a Function
To call a function, use the function name followed by parenthesis:
def my_function():
print("Hello from a function")
my_function()
Arguments
Information can be passed into functions as arguments.
Arguments are specified after the function name, inside the parentheses.
You can add as many arguments as you want, just separate them with a comma.
Parameters or Arguments
The following example has a function with one argument (fname). When the function is called, we pass along a first name, which is used inside the function to print the full name:
def my_function(fname):
print(fname + " is my name")
my_function(“farah")
my_function("Tobias")
Number of Arguments
By default, a function must be called with the correct number of arguments.
Meaning that if your function expects 2 arguments, you have to call the function with 2 arguments, not more, and not less.
#This function expects 2 arguments, and gets 2 arguments:
def my_function(fname, lname):
print(fname + " " + lname)
my_function(“dorra", “kin")
Arbitrary Arguments, *args
If you do not know how many arguments that will be passed into your function, add a * before the parameter name in the function definition.
This way the function will receive a tuple of arguments
That means we have 1 keyword argument but different values.
Arbitrary Keyword Arguments, **kwargs
If you do not know how many keyword arguments that will be passed into your function, add two asterisk: ** before the parameter name in the function definition.
That means we have lots of keywords to add without knowing its number and not values.
Default Parameter Value
def my_function(country = "Norway"):
print("I am from " + country)
my_function("Sweden")
my_function("India")
my_function()
my_function("Brazil")
If we do not assign any value to country it will return us Norway, but if we assign it will change
Passing a List as an Argument
You can send any data types of argument to a function (string, number, list, dictionary etc.), and it will be treated as the same data type inside the function.
def my_function(food): for x in food:
print(x)
fruits = ["apple", "banana", "cherry"]
my_function(fruits)
0 Comments