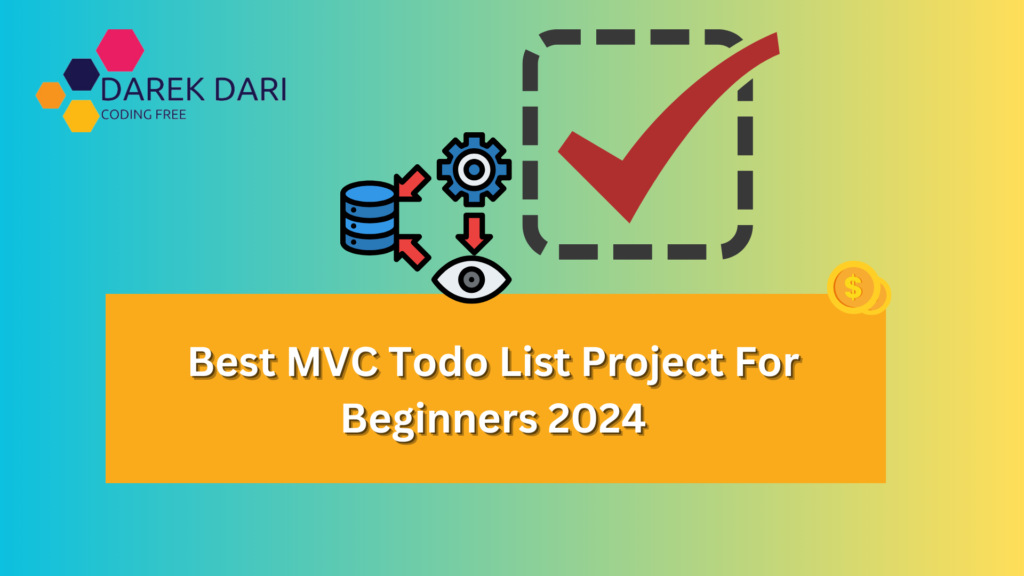
Table of Contents
Introduction
Creating a simple Todo List application is an excellent opportunity to gain hands-on experience with the MVC (Model-View-Controller) pattern.
In this tutorial, we will utilize Python for the backend, Flask as our web framework, and SQLite for the database. By the end of this guide, you will have a fully functional Todo List application and a solid grasp of how MVC operates.
What is MVC?
Now, let’s take a moment to understand what MVC is before we dive into coding. MVC is a design pattern that divides an application into three key components:
Component | Role | Example |
---|---|---|
Model | Manages the data and business logic | Think of it as a filing cabinet where you store, retrieve, and manage your task files. |
View | Renders the user interface | It’s the display window of your shop, showing the list of tasks and providing forms to add or delete tasks. |
Controller | Handles user input and updates the model | The shopkeeper who takes your requests (add/delete tasks), processes them, and updates the display window. |
By separating these concerns, MVC enhances the modularity and maintainability of your code.
todo project
js mvc
mvc js
todomvc
Why Use MVC?
MVC enhances the organization and manageability of your code. Here’s how it works:
Modularity: Each component (Model, View, Controller) has a specific role, making it easier to manage and scale the application. Think of it like organizing a big event. Instead of one person handling everything, you have one person for invitations (Model), another for setting up the venue (View), and a coordinator to ensure everything runs smoothly (Controller).
js framework mvc
js mvc framework
mvc framework for javascript
mvc framework in javascript
Reusability: Components can be reused. For instance, you can change how tasks are displayed (the view) without impacting how they are stored (the model). It’s similar to revamping your shop window without altering the contents of the storage room.
Maintainability: With this organized structure, it becomes simpler to locate and fix bugs, add new features, or update existing ones. It’s akin to having a well-organized toolbox – you always know where to find what you need.
architecture mvc
mvc architecture
modèle mvc
Setting Up Your Project
Let’s go through setting up the project directory step-by-step, with a bit more detail and explanation.
Setting Up Your Project Directory
To begin, let’s establish a dedicated space on your computer where all the files for our Todo List application will reside.
Consider this as creating your own workshop, where you’ll construct and arrange all the components of your project.
Step 1: Open Your Terminal
Depending on your operating system, you’ll need to open a terminal or command prompt. Here’s how you can do it on different systems:
- Windows: You can use Command Prompt or PowerShell. To open it, you can search for “cmd” or “PowerShell” in the start menu and click on it.
- MacOS: You can use the Terminal app. You can find it by searching for “Terminal” in Spotlight or navigating to Applications > Utilities > Terminal.
- Linux: You can use the terminal. Open it from your application menu or use the shortcut Ctrl+Alt+T.
Step 2: Create a New Directory
After opening your terminal, the first step is to create a new directory for your project. This directory will serve as a folder to store all the files associated with your Todo List app.
To do this, simply type the following command in your terminal and hit Enter:
mkdir todo_app
Here’s what this command does:
mkdir
: This stands for “make directory.” It’s a command that tells your system you want to create a new folder.todo_app
: This is the name of the new directory you’re creating. You can name it whatever you like, buttodo_app
is a good descriptive name for this project.
mvc design pattern
design patterns mvc
Step 3: Navigate to Your New Directory
After setting up a new directory, make sure to enter it in order to begin adding files and developing your application.
Simply input the command below in your terminal and hit Enter:
cd todo_app
This is the purpose of the following command:
- cd: It is short for “change directory,” indicating to your system that you wish to navigate to a new folder.
- todo_app: This is the specific directory you intend to access. Make sure it matches the name you specified when creating it with the mkdir command.
Putting It All Together
So, to summarize, you open your terminal and run these two commands one after the other:
mkdir todo_app
cd todo_app
After executing the first command, a new folder named todo_app will be created.
Once you run the second command, you will be directed into that folder. This means that any subsequent commands you execute will be performed within this project directory.
Now that you are inside your project directory, you are all set to begin creating files and developing your Todo List application.
It’s similar to entering a brand new workshop, fully prepared to craft and arrange your project components!
Absolutely! Let’s dive into creating our app.py
file, which is like the main entry point of our Flask application. Think of it as the front door to our workshop where everything starts.
mvc framework javascript
mvc framework js
todo mvc
react todo list tutorial
Creating app.py
In this file, we’ll set up and configure Flask to get our application running smoothly.
Importing Flask:
First, we need to bring in Flask, which is a powerful web framework for Python.
from flask import Flask
This line simply imports Flask so we can use it to build our web application.
Creating the Flask Application:
Next, we create an instance of the Flask class. This instance will be our web application. We often name it app
.
app = Flask(__name__)
Here, Flask(__name__)
initializes a new Flask web application. The __name__
variable holds the name of the current module, and Flask uses it to know where to look for resources like templates and static files.
Setting Up the Main Entry Point:
Now, we define the main entry point of our application. This part of the code runs when we execute app.py
directly.
if __name__ == "__main__":
app.run(debug=True)
This block of code checks if the script is being run directly (not imported as a module). If it is, app.run(debug=True)
starts the Flask development server with debugging enabled. Debug mode is helpful during development because it provides detailed error messages and auto-reloads the server when you make code changes.
model view controller example
model-view controller example
model-view-controller example
mvc architecture example
What Happens When You Run This Code?
When you run app.py in your terminal:
- Flask will initialize and start a web server.
- The server will be listening for incoming requests on the default port (5000).
- If you visit http://127.0.0.1:5000 in your browser without defining any routes, you will see a 404 Not Found error.
This happens because Flask hasn’t been instructed on how to handle requests yet. Don’t worry, we’ll be adding routes soon to manage different paths and actions.
By setting up app.py, you’ve established the groundwork for your Flask application. It’s like unlocking the front door to your workshop – now we’re all set to begin building!
Next, we’ll create models.py to handle our data, followed by views.py to control how things are displayed, and controllers.py to manage user interactions and inputs.
react todo list example
simple todo list react
to do app react
to do react
Creating models.py
Let’s discuss setting up models.py
, where we handle our data model using SQLite. This file is like the storage room of our workshop where we manage all the tasks for our Todo List application.
In this file, we’ll use SQLite, a lightweight relational database management system, to manage our tasks.
Setting Up SQLite:
To start, we import sqlite3
, a Python library that provides a SQL interface for SQLite databases.
import sqlite3
Initializing the Database:
The init_db()
function sets up our SQLite database. It connects to todo.db
(or creates it if it doesn’t exist), and creates a table named todos
if it hasn’t been created already. This table will store our tasks.
def init_db():
conn = sqlite3.connect('todo.db')
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS todos (
id INTEGER PRIMARY KEY,
task TEXT NOT NULL
)
''')
conn.commit()
conn.close()
Adding a Task:
The add_task(task)
function allows us to add a new task to our todos
table. It connects to the database, inserts the task into the table, and commits the changes.
def add_task(task):
conn = sqlite3.connect('todo.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO todos (task) VALUES (?)', (task,))
conn.commit()
conn.close()
Retrieving Tasks:
The get_tasks()
function retrieves all tasks from the todos
table. It connects to the database, executes a SELECT
query to fetch all rows, fetches the results, and closes the connection.
def get_tasks():
conn = sqlite3.connect('todo.db')
cursor = conn.cursor()
cursor.execute('SELECT * FROM todos')
tasks = cursor.fetchall()
conn.close()
return tasks
mvc model
mvc model view
mvc model-view controller
mvc structure
mvc pattern
what is a mvc
Deleting a Task:
The function delete_task(task_id) removes a task from the todos table using its unique id. It establishes a connection to the database, runs a DELETE query for the given task_id, saves the modifications, and then closes the connection.
def delete_task(task_id):
conn = sqlite3.connect('todo.db')
cursor = conn.cursor()
cursor.execute('DELETE FROM todos WHERE id = ?', (task_id,))
conn.commit()
conn.close()
What Does This Code Do?
When the init_db() function is executed, it will generate a todo.db file (if it doesn’t already exist) and establish a todos table within it for task storage.
By utilizing add_task() to insert new tasks, get_tasks() to retrieve all tasks, and delete_task() to eliminate a task based on its ID, we can efficiently manage our tasks using a database.
what is model view controller
what is mvc
model view
mvc meaning
model-view controller diagram
mvc model example
This configuration ensures that our Todo List application can effectively store, retrieve, and delete tasks. In our workshop comparison, models.py serves as the hub for managing and organizing all tools and materials related to tasks.
Moving forward, we will proceed with the creation of views.py to determine how tasks are presented to users, followed by controllers.py to oversee user interactions and inputs.
These combined files will form the fundamental structure of our Todo List application.
to-do app react
todo react app
react todo app tutorial
todolist in react
Creating views.py
The views.py
file is all about how our application presents information to users. It handles rendering the HTML templates, which means displaying the tasks in a user-friendly format. Think of it as the display window of our workshop, where everything is neatly arranged for everyone to see.
Setting Up the Views:
First, we need to import a few essentials. We import render_template
from Flask to render HTML files, and we also need our Flask app instance and our get_tasks
function from models.py
.
from flask import render_template
from app import app
from models import get_tasks
Defining a Route:
A route is a URL pattern that the application recognizes. When users visit a specific URL, the route tells Flask which function to execute. In our case, we want to display our list of tasks when the user visits the home page.
@app.route("/")
def index():
tasks = get_tasks()
return render_template("index.html", tasks=tasks)
Here’s what’s happening in this function:
@app.route("/")
: This decorator tells Flask to execute theindex()
function when the user visits the root URL (/
).def index():
: This is the function that will run when the user visits the root URL.tasks = get_tasks()
: This line fetches all the tasks from the database using theget_tasks()
function we defined inmodels.py
.return render_template("index.html", tasks=tasks)
: This line renders theindex.html
template and passes the list of tasks to it. Thetasks=tasks
part makes the tasks available in the template.
mvc examples
mvc model view controller example
mvc pattern example
model view controller example
model-view controller example
model-view-controller example
Creating the HTML Template index.html
Next, we need to create the HTML template that will display our tasks. This file should be placed in a folder named templates
within your project directory. The templates
folder is where Flask looks for HTML templates by default.
Example of index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Todo List</title>
</head>
<body>
<h1>My Todo List</h1>
<ul>
{% for task in tasks %}
<li>{{ task[1] }}</li>
{% endfor %}
</ul>
<form action="/add" method="post">
<input type="text" name="task" placeholder="New task">
<input type="submit" value="Add">
</form>
</body>
</html>
Here’s a breakdown of what’s happening in this HTML file:
{% for task in tasks %}
: This is a Jinja2 template loop that iterates over the list of tasks passed from the view. Jinja2 is Flask’s template engine.<li>{{ task[1] }}</li>
: This line displays each task within a list item (<li>
).task[1]
refers to the task description (the second item in each task tuple).- The form: This form allows users to add a new task. When submitted, it sends a POST request to the
/add
route, which we’ll define later.
What Happens When You Run This Code?
When you navigate to the root URL (http://127.0.0.1:5000/
), the index()
function runs, fetching the list of tasks from the database. It then renders the index.html
template, passing the tasks to it. The template displays the tasks in an unordered list and includes a form to add new tasks.
With views.py
in place, we have a neat display window showing our tasks. Next, we’ll create controllers.py
to handle user interactions, such as adding and deleting tasks. This completes the core functionality of our Todo List application.
mvc architecture example
mvc architecture with example
mvc framework example
model view controller explained
Creating views.py
The views.py
file is all about how our application presents information to users. It handles rendering the HTML templates, which means displaying the tasks in a user-friendly format. Think of it as the display window of our workshop, where everything is neatly arranged for everyone to see.
Setting Up the Views:
First, we need to import a few essentials. We import render_template
from Flask to render HTML files, and we also need our Flask app instance and our get_tasks
function from models.py
.
from flask import render_template
from app import app
from models import get_tasks
Defining a Route:
A route serves as a URL pattern that the application can identify. When visitors go to a particular URL, the route guides Flask on which function to run. For our situation, we aim to showcase our task list when users land on the homepage.
@app.route("/")
def index():
tasks = get_tasks()
return render_template("index.html", tasks=tasks)
Here’s what’s happening in this function:
@app.route("/")
: This decorator tells Flask to execute theindex()
function when the user visits the root URL (/
).def index():
: This is the function that will run when the user visits the root URL.tasks = get_tasks()
: This line fetches all the tasks from the database using theget_tasks()
function we defined inmodels.py
.return render_template("index.html", tasks=tasks)
: This line renders theindex.html
template and passes the list of tasks to it. Thetasks=tasks
part makes the tasks available in the template.
reactjs todo app tutorial
todo app react example
Creating the HTML Template index.html
After that, it’s important to generate the HTML template for showcasing our tasks. You should save this file in a directory called templates in your project folder. Flask automatically searches for HTML templates in the templates folder.
Then, we have to produce the HTML template to showcase our tasks. Save this file in a folder named templates within your project directory. Flask will search for HTML templates in the templates folder by default.
Example of index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Todo List</title>
</head>
<body>
<h1>My Todo List</h1>
<ul>
{% for task in tasks %}
<li>{{ task[1] }}</li>
{% endfor %}
</ul>
<form action="/add" method="post">
<input type="text" name="task" placeholder="New task">
<input type="submit" value="Add">
</form>
</body>
</html>
Here is an overview of the current situation in this HTML file:
- {% for task in tasks %}: This loop is a Jinja2 template that goes through the tasks list provided by the view. Jinja2 is the template engine used by Flask.
- {{ task[1] }}: This particular line showcases each task in a list item (). task[1] represents the task description (the second item in each task tuple).
- The form: This form enables users to include a new task. Once submitted, it sends a POST request to the /add route, which we will elaborate on later.
What Happens When You Run This Code?
Upon visiting the root URL (http://127.0.0.1:5000/), the index() function is executed, retrieving tasks from the database.
These tasks are then passed to the index.html template for rendering. The template showcases the tasks in an unordered list and provides a form for adding new tasks.
With views.py set up, we now have a clean interface displaying our tasks. Moving forward, we will develop controllers.py to manage user interactions like adding and removing tasks.
This finalizes the essential features of our Todo List application.
model view controller
model-view controller
model-view-controller
Creating HTML Template index.html
Here’s a simple HTML template for your Todo List application:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
<title>Todo List</title>
</head>
<body>
<div class="container">
<h1>Todo List</h1>
<form action="/add" method="post">
<input type="text" name="task" placeholder="New Task">
<button type="submit">Add</button>
</form>
<ul>
{% for task in tasks %}
<li>
{{ task[1] }}
<a href="/delete/{{ task[0] }}">Delete</a>
</li>
{% endfor %}
</ul>
</div>
</body>
</html>
mvc structure
model view architecture
nj dmv appointment
Creating CSS style.css
Add some basic styling with this CSS file:
body {
font-family: Arial, sans-serif;
background-color: #f8f9fa;
}
.container {
width: 300px;
margin: 50px auto;
padding: 20px;
background-color: white;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
font-size: 24px;
text-align: center;
}
form {
display: flex;
justify-content: space-between;
margin-bottom: 20px;
}
input[type="text"] {
width: 70%;
padding: 5px;
}
button {
padding: 5px 10px;
}
ul {
list-style-type: none;
padding: 0;
}
li {
padding: 10px;
display: flex;
justify-content: space-between;
}
li a {
color: red;
text-decoration: none;
}
Initializing the Database
Run this script once to create the database and the todos
table:
from models import init_db
init_db()
Running the Application
Make sure to import views
and controllers
in app.py
:
from flask import Flask
app = Flask(__name__)
import views
import controllers
if __name__ == "__main__":
app.run(debug=True)
Now, run your application:
python app.py
Open your browser and navigate to http://127.0.0.1:5000
to see your Todo List application in action!
Feel free to reach out if you have any questions or need further assistance. Enjoy building your Todo List app!
mvc
mvc nj
new jersey department of motor vehicles
nj dmv
nj motor vehicle commission
Creating controllers.py
This file will handle user inputs and interactions:
from flask import request, redirect, url_for
from app import app
from models import add_task, delete_task
@app.route("/add", methods=["POST"])
def add():
task = request.form.get("task")
if task:
add_task(task)
return redirect(url_for("index"))
@app.route("/delete/<int:task_id>")
def delete(task_id):
delete_task(task_id)
return redirect(url_for("index"))
Conclusion
We have successfully established the core components of our Todo List application using the MVC (Model-View-Controller) architecture with Python, Flask, and SQLite.
Initially, we organized our project directory to maintain file structure. Subsequently, we developed app.py
, the central component of our application, which initializes the Flask app and configures the primary entry point.
Moving forward, we progressed to models.py
, where we defined our data model utilizing SQLite. This file contains functions for database initialization, task addition, retrieval, and deletion.
Lastly, in views.py
, we specified how information is presented to users. By creating a route and rendering an HTML template, we showcased our task list in a user-friendly manner.
With these elements in position, we have a functional framework for our Todo List application. Upon launching our Flask server, we can view the tasks on the home page.
In the upcoming stages, we will enhance functionality by managing user interactions (such as task addition and deletion) and refining the application’s appearance.
mvc model
model view controller
model-view-controller
mvc
If you have any queries or require further support, please don’t hesitate to contact us. Enjoy the process of constructing your Todo List app, and happy coding!
0 Comments