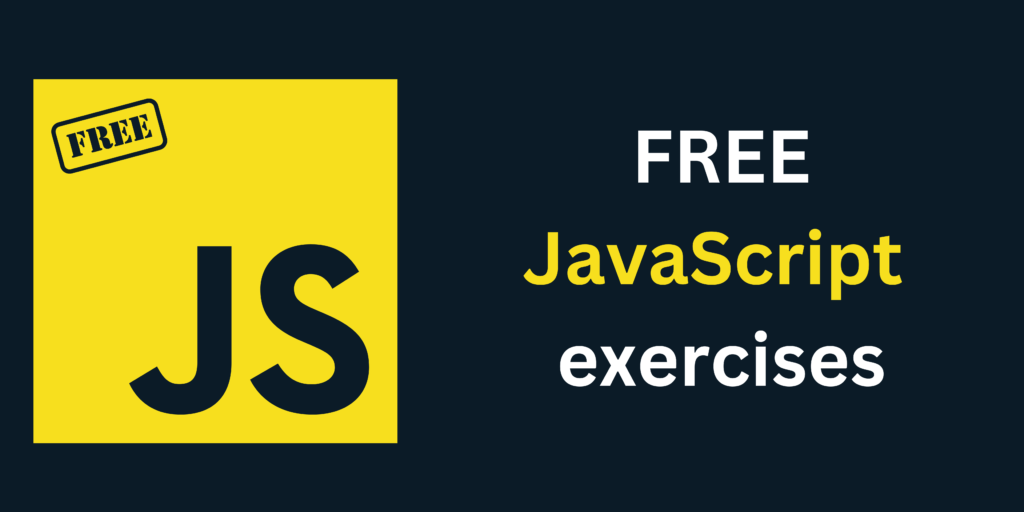
Write a function that takes in an array of numbers and returns the sum of all the numbers that are divisible by 3 or 5.
function sumOfDivisibleNumbers(arr) {
let sum = 0;
for(let i = 0; i < arr.length; i++) {
if(arr[i] % 3 === 0 || arr[i] % 5 === 0) {
sum += arr[i];
}
}
return sum;
}
Write a program that generates a random number between 1 and 100, and then prompts the user to guess the number. If the user’s guess is higher than the random number, the program should output “Too high!” If the user’s guess is lower than the random number, the program should output “Too low!” If the user guesses the number correctly, the program should output “You win!”.
let randomNum = Math.floor(Math.random() * 100) + 1;
let guess = parseInt(prompt("Guess a number between 1 and 100:"));
while(guess !== randomNum) {
if(guess > randomNum) {
guess = parseInt(prompt("Too high! Guess again:"));
} else {
guess = parseInt(prompt("Too low! Guess again:"));
}
}
alert("You win!");
Write a program that prompts the user to enter a string, and then determines whether or not the string is a palindrome (a word or phrase that is spelled the same way forwards and backwards).
function isPalindrome(str) {
str = str.toLowerCase();
for(let i = 0; i < str.length / 2; i++) {
if(str[i] !== str[str.length - i - 1]) {
return false;
}
}
return true;
}
Write a program that prompts the user to enter two strings, and then determines whether or not the two strings are anagrams (words that have the same letters but in a different order).
function isAnagram(str1, str2) {
str1 = str1.toLowerCase().split("").sort().join("");
str2 = str2.toLowerCase().split("").sort().join("");
return str1 === str2;
}
Write a program that prompts the user to enter a sentence, and then determines the most common letter in the sentence.
function mostCommonLetter(str) {
let counts = {};
for(let i = 0; i < str.length; i++) {
let char = str[i];
if(counts[char]) {
counts[char]++;
} else {
counts[char] = 1;
}
}
let maxChar = "";
let maxCount = 0;
for(let char in counts) {
if(counts[char] > maxCount) {
maxChar = char;
maxCount = counts[char];
}
}
return maxChar;
}
Write a program that prompts the user to enter a number, and then determines whether or not the number is a prime number (a number that is only divisible by 1 and itself).
function isPrime(num) {
if(num < 2) {
return false;
}
for(let i = 2; i < num; i++) {
if(num % i === 0) {
return false;
}
}
return true;
}
Write a program that generates a random word from a list of words, and then prompts the user to enter a sentence containing the random word. The program should then determine whether or not the sentence contains the random word.
let words = ["apple", "banana", "cherry", "orange", "pear"];
let randomWord = words[Math.floor(Math.random() * words.length)];
let sentence = prompt("Enter a sentence:");
if(sentence.includes(randomWord)) {
alert("The sentence contains the random word!");
} else {
alert("The sentence does not contain the random word.");
}
Write a program that prompts the user to enter a number, and then outputs the Fibonacci sequence up to that number.
let num = parseInt(prompt("Enter a number:"));
let a = 0;
let b = 1;
let fibonacci = [a, b];
while(b < num) {
let temp = b;
b = a + b;
a = temp;
fibonacci.push(b);
}
alert(fibonacci.join(", "));
Write a program that prompts the user to enter a string, and then determines whether or not the string is a pangram (a sentence that contains every letter of the alphabet).
function isPangram(str) {
str = str.toLowerCase();
for(let char of "abcdefghijklmnopqrstuvwxyz") {
if(!str.includes(char)) {
return false;
}
}
return true;
}
Write a program that prompts the user to enter a string, and then determines whether or not the string is a valid email address.
function isValidEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments