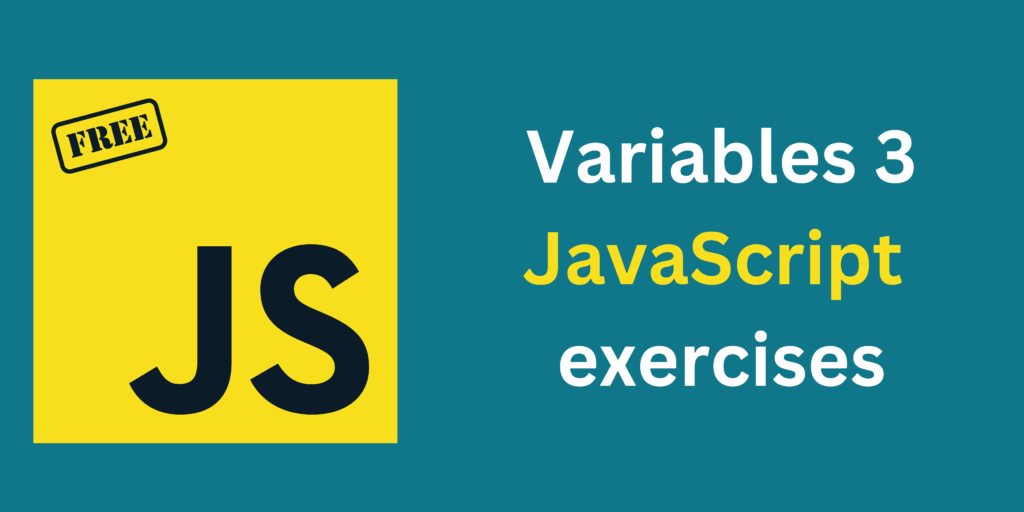
In these javascript exercises, we’ll investigate a few more intricate scenarios involving JavaScript variables. You will have to use your understanding of variables, loops, conditionals, and other programming concepts to solve the problems in these exercises.
The solutions offered will show various methods for resolving these issues, but there may be additional approaches. You’ll gain a deeper comprehension of how to use variables in JavaScript to create more intricate programs by practicing the exercises provided.
javascript exercises
javascript exercises and solutions
javascript exercises for beginners
javascript function exercises
javascript practice exercises with solutions
javascript tasks for practice
javascript variables exercises
js exercises
JavaScript Exercises
- Write a program that takes an array of numbers as input and returns the sum of all even numbers in the array.
let nums = [2, 5, 6, 9, 10, 12, 15, 18];
let sum = 0;
for(let i = 0; i < nums.length; i++) {
if(nums[i] % 2 == 0) {
sum += nums[i];
}
}
console.log("The sum of all even numbers in the array is " + sum);
- Write a program that takes two arrays of numbers as input and returns a new array containing only the numbers that appear in both arrays.
let arr1 = [2, 5, 6, 9, 10, 12, 15, 18];
let arr2 = [3, 5, 7, 9, 12, 15, 18, 21];
let common = [];
for(let i = 0; i < arr1.length; i++) {
for(let j = 0; j < arr2.length; j++) {
if(arr1[i] == arr2[j]) {
common.push(arr1[i]);
break;
}
}
}
console.log("The common numbers in both arrays are " + common);
- Write a program that takes a string as input and returns the most common character in the string.
let str = "the quick brown fox jumps over the lazy dog";
let charMap = {};
let maxChar = "";
let maxCount = 0;
for(let i = 0; i < str.length; i++) {
let char = str[i];
if(charMap[char]) {
charMap[char]++;
}
else {
charMap[char] = 1;
}
if(charMap[char] > maxCount) {
maxChar = char;
maxCount = charMap[char];
}
}
console.log("The most common character in the string is '" + maxChar + "' with a count of " + maxCount);
- Write a program that takes a number as input and returns a string of that many random letters.
let length = 10;
let alphabet = "abcdefghijklmnopqrstuvwxyz";
let randomString = "";
for(let i = 0; i < length; i++) {
let randomIndex = Math.floor(Math.random() * alphabet.length);
let randomChar = alphabet[randomIndex];
randomString += randomChar;
}
console.log("The random string is " + randomString);
5. Write a program that takes a string as input and returns the string with each word capitalized.
let str = "the quick brown fox jumps over the lazy dog";
let words = str.split(" ");
let capitalized = "";
for(let i = 0; i < words.length; i++) {
let word = words[i];
let firstChar = word.charAt(0).toUpperCase();
let restOfWord = word.slice(1);
capitalized += firstChar + restOfWord + " ";
}
console.log("The capitalized string is " + capitalized.trim());
6. Write a program that takes an array of numbers as input and returns the second largest number in the array.
let nums = [2, 5, 6, 9, 10, 12, 15, 18];
let largest = nums[0];
let secondLargest = nums[0];
for(let i = 1; i < nums.length; i++) {
if(nums[i] > largest) {
secondLargest = largest;
largest = nums[i];
}
else if(nums[i] > secondLargest) {
secondLargest = nums[i];
}
}
console.log("The second largest number in the array is " + secondLargest);
javascript exercises
javascript exercises and solutions
javascript exercises for beginners
javascript function exercises
javascript practice exercises with solutions
javascript tasks for practice
javascript variables exercises
js exercises
7. Write a program that takes an array of numbers as input and returns a new array where each number is multiplied by 2.
let nums = [2, 5, 6, 9, 10, 12, 15, 18];
let multiplied = [];
for(let i = 0; i < nums.length; i++) {
multiplied.push(nums[i] * 2);
}
console.log("The multiplied array is " + multiplied);
8. Write a program that takes two strings as input and returns true if they are anagrams of each other, and false otherwise.
let str1 = "listen";
let str2 = "silent";
let sortedStr1 = str1.split("").sort().join("");
let sortedStr2 = str2.split("").sort().join("");
if(sortedStr1 === sortedStr2) {
console.log("The two strings are anagrams.");
}
else {
console.log("The two strings are not anagrams.");
}
9. Write a program that takes an array of strings as input and returns a new array where each string has the first letter removed.
let strings = ["hello", "world", "javascript", "programming"];
let removedFirstLetter = [];
for(let i = 0; i < strings.length; i++) {
removedFirstLetter.push(strings[i].slice(1));
}
console.log("The new array is " + removedFirstLetter);
10. Write a program that takes two arrays as input and returns a new array where each element is the sum of the corresponding elements from the two input arrays.
let nums1 = [2, 5, 6, 9];
let nums2 = [3, 7, 1, 4];
let sum = [];
for(let i = 0; i < nums1.length; i++) {
sum.push(nums1[i] + nums2[i]);
}
console.log("The sum array is " + sum);
javascript exercises
javascript exercises and solutions
javascript exercises for beginners
javascript function exercises
javascript practice exercises with solutions
javascript tasks for practice
javascript variables exercises
js exercises
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
1 Comment
creación de cuenta en Binance · March 29, 2024 at 6:48 am
Your article helped me a lot, is there any more related content? Thanks!