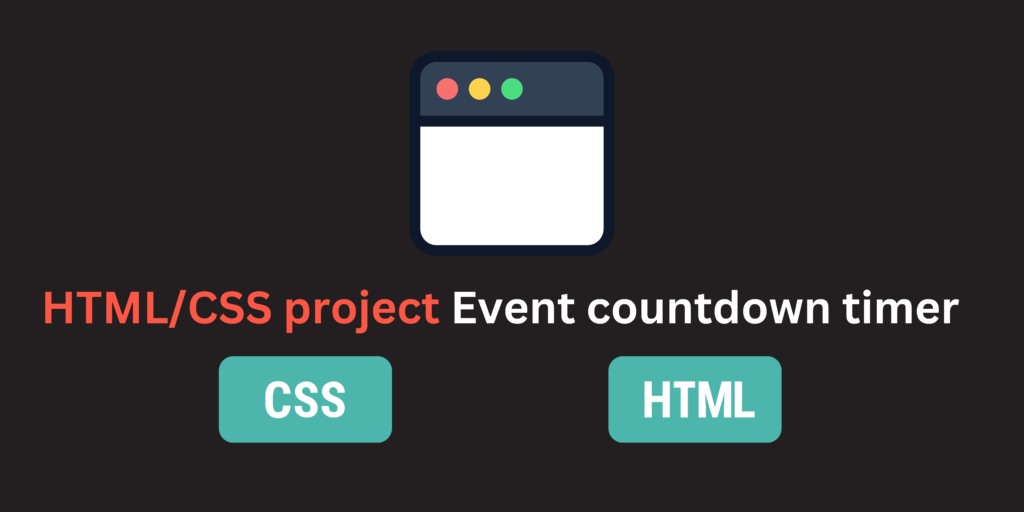
Three files must be created in this example: script.js, styles.css, and index.html. Save the JavaScript code in script.js, the CSS code in styles.css, and the HTML code in index.html. Keep all three files in the same directory, please.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Event Countdown Timer</title>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<div class="countdown-container">
<h1>Event Countdown Timer</h1>
<div id="countdown"></div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
}
.countdown-container {
max-width: 400px;
margin: 100px auto;
text-align: center;
background-color: #fff;
padding: 20px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
font-size: 24px;
margin-bottom: 20px;
}
#countdown {
font-size: 36px;
font-weight: bold;
color: #333;
}
Javascript
// Set the event date and time (year, month, day, hour, minute, second)
var eventDate = new Date('2023-12-31T00:00:00').getTime();
// Update the countdown every second
var countdown = setInterval(function() {
// Get the current date and time
var now = new Date().getTime();
// Calculate the time remaining until the event
var timeRemaining = eventDate - now;
// Calculate days, hours, minutes, and seconds
var days = Math.floor(timeRemaining / (1000 * 60 * 60 * 24));
var hours = Math.floor((timeRemaining % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((timeRemaining % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((timeRemaining % (1000 * 60)) / 1000);
// Display the countdown
document.getElementById('countdown').innerHTML = days + 'd ' + hours + 'h ' + minutes + 'm ' + seconds + 's';
// If the event date has passed, clear the countdown
if (timeRemaining < 0) {
clearInterval(countdown);
document.getElementById('countdown').innerHTML = 'Event has already occurred!';
}
}, 1000);
The date and time of the event you want to countdown to are represented by the JavaScript variable eventDate. Your preferred event date should be updated.
The countdown timer ought to be visible when you open index.html in a web browser. Up until the event date, the timer will update every second.
Learn more
JavaScript ile neler yapılabilir? JavaScript öğrenmek ne kadar sürer? JavaScript hangi program? JavaScript neden popüler?¿Qué proyectos se pueden hacer con JavaScript? ¿Qué es un proyecto en JavaScript? ¿Cómo empezar un proyecto de JavaScript? ¿Qué programas se han hecho con JavaScript? Wird JavaScript noch benötigt? Was kann man alles mit JavaScript machen? Ist JavaScript für Anfänger? Wie schwierig ist JavaScript? مشاريع جافا سكريبت للمبتدئين مشاريع جافا سكريبت جاهزة pdf مشروع جافا سكريبت javascript مشروع جافا سكريبت github تفعيل جافا سكريبت على الهاتف مشاريع جافا للمبتدئين جافا سكريبت تحميل تحميل جافا سكريبت للاندرويد
0 Comments