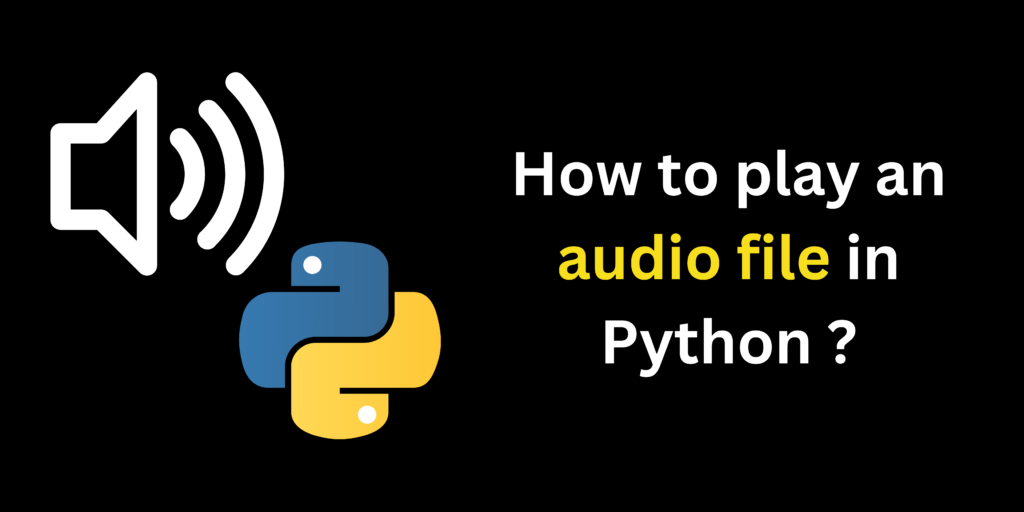
To play sound Python, you can use the Pygame library. Here are the steps to do so:
- Install Pygame library: You can install Pygame library by running the following command in your terminal or command prompt:
pip install pygame
Import the library: Once you have installed the Pygame library, you can import it in your Python script using the following command:
import pygame
Initialize the Pygame mixer: Before playing any audio file, you need to initialize the Pygame mixer using the following command:
pygame.mixer.init()
Load the audio file: You can load the audio file using the following command:
pygame.mixer.music.load('path/to/your/audio/file.mp3')
Play the audio file: Finally, you can play the audio file using the following command:
pygame.mixer.music.play()
Play sound example
Here’s an example code snippet that puts all these steps together:
import pygame
# initialize pygame mixer
pygame.mixer.init()
# load audio file
pygame.mixer.music.load('path/to/your/audio/file.mp3')
# play audio file
pygame.mixer.music.play()
# wait for audio to finish playing
while pygame.mixer.music.get_busy():
continue
Conclusion
Note that you need to replace path/to/your/audio/file.mp3
with the actual path to your audio file. Also, in the example above, the code waits for the audio file to finish playing before the program terminates by using a while
loop that keeps running until the audio finishes playing.
For more python exercises and project: Click here
To join our community:
Telegram: Click here
Facebook: Click here
0 Comments