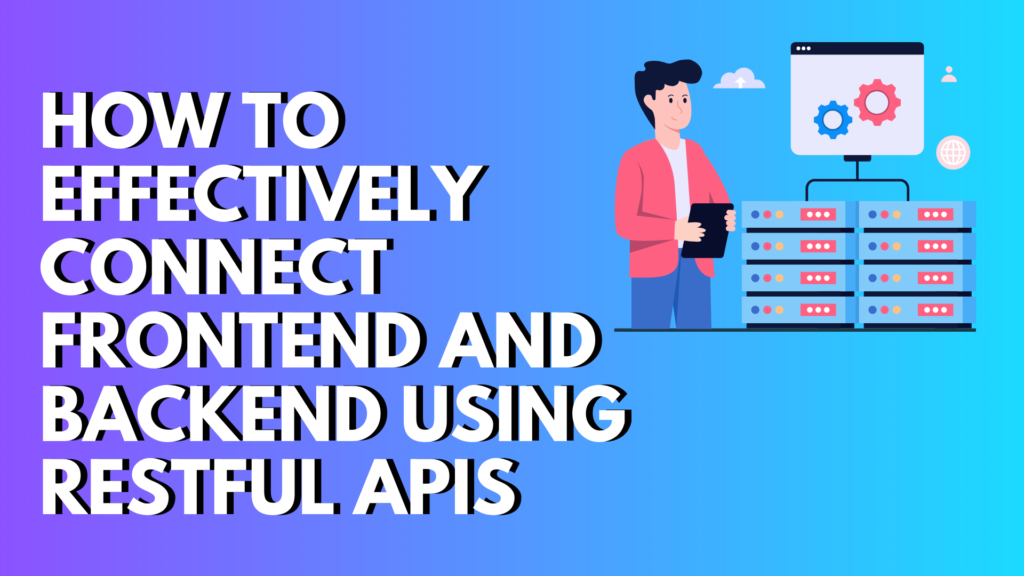
Introduction: Understanding the Importance of Frontend-Backend Integration
In modern web development, creating a seamless interaction between the frontend and backend is essential for building dynamic, responsive applications. One of the most efficient ways to achieve this integration is through RESTful APIs. Whether you’re building a simple app or a complex platform, understanding how to connect your frontend with the backend through APIs is fundamental.
This comprehensive guide will take you through the process of connecting the frontend and backend using RESTful APIs, covering everything from setting up your API to handling responses, errors, and security. By the end of this article, you will have a clear understanding of how to implement API calls, ensure smooth communication, and create robust, scalable web applications.
What are RESTful APIs?
Definition and Key Concepts
A RESTful API (Representational State Transfer) is an architectural style that uses the HTTP protocol to enable communication between a client (frontend) and a server (backend). The primary benefit of RESTful APIs is that they allow frontend and backend systems to interact independently yet cohesively.
Core Features of RESTful APIs:
- Stateless: Each API request is independent, meaning the server doesn’t store information about previous requests.
- Client-Server Architecture: The frontend (client) and backend (server) are separated, allowing independent development and scaling.
- HTTP Methods: RESTful APIs use standard HTTP methods like GET, POST, PUT, and DELETE to manage resources.
- Data Format: Typically, RESTful APIs send and receive data in JSON or XML format, with JSON being the most common.
Why Use RESTful APIs?
- Scalability: REST APIs are designed to handle high volumes of requests and large datasets.
- Independence: The frontend and backend are decoupled, enabling easier updates, maintenance, and scalability.
- Flexibility: You can use RESTful APIs in a variety of languages and frameworks, making them versatile for any project.
How to Connect Frontend and Backend Using RESTful APIs
Now that we understand what RESTful APIs are, let’s explore the steps involved in connecting the frontend and backend.
1. Setting Up the Backend API
The backend is where your API resides. It is responsible for handling requests from the frontend, processing them, and sending back appropriate responses.
Node.js with Express Example:
- Install Node.js and the Express framework to set up the backend API.
- Define routes for your API, like
/users
,/tasks
, or/posts
, depending on the application you’re building. - Use GET, POST, PUT, and DELETE methods to handle requests.
Example (Express.js):
const express = require('express');
const app = express();
app.use(express.json());
// GET request to fetch all tasks
app.get('/api/tasks', (req, res) => {
res.json({ tasks: ['Task 1', 'Task 2', 'Task 3'] });
});
// POST request to add a new task
app.post('/api/tasks', (req, res) => {
const newTask = req.body.task;
res.json({ message: 'Task added', task: newTask });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
2. Setting Up the Frontend
The frontend is responsible for sending requests to the backend API and displaying the data returned by the API. You can use React.js, Vue.js, or plain HTML/CSS/JS for the frontend.
React.js Example:
React is a powerful JavaScript library that simplifies building interactive UIs. In React, you can make API calls using fetch or Axios.
Example (React.js):
import React, { useEffect, useState } from 'react';
function App() {
const [tasks, setTasks] = useState([]);
useEffect(() => {
fetch('http://localhost:3000/api/tasks')
.then(response => response.json())
.then(data => setTasks(data.tasks))
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>Tasks</h1>
<ul>
{tasks.map((task, index) => (
<li key={index}>{task}</li>
))}
</ul>
</div>
);
}
export default App;
3. Handling API Responses
Once the frontend sends a request to the backend, it expects a response, usually in JSON format. Here’s how you can handle the response in the frontend:
- React.js: Use state to store the response and update the UI accordingly.
- Error Handling: Always include error handling in case the API request fails.
Example (Error Handling in React):
fetch('http://localhost:3000/api/tasks')
.then(response => response.json())
.then(data => setTasks(data.tasks))
.catch(error => console.error('Error:', error));
4. Authentication and Security
When working with APIs, it’s crucial to secure your endpoints to prevent unauthorized access. Here’s how you can handle authentication:
- JWT (JSON Web Tokens): Use JWT to authenticate API requests and ensure that only authorized users can access certain endpoints.
- CORS (Cross-Origin Resource Sharing): Ensure the backend allows requests from different domains (especially important during development).
Example (Express.js JWT Authentication):
const jwt = require('jsonwebtoken');
// Middleware to protect routes
function authenticate(req, res, next) {
const token = req.headers['authorization'];
if (!token) return res.status(403).send('No token provided');
jwt.verify(token, 'your-secret-key', (err, decoded) => {
if (err) return res.status(500).send('Failed to authenticate token');
req.userId = decoded.id;
next();
});
}
Best Practices for Connecting Frontend and Backend
- Use HTTPS: Always encrypt data between the frontend and backend by using HTTPS.
- Limit API Requests: Optimize the number of API requests to reduce latency and improve performance.
- API Rate Limiting: Prevent abuse of your API by implementing rate limiting.
- Error Handling: Make sure both frontend and backend have proper error-handling mechanisms.
Common Challenges and How to Overcome Them
- Latency: Network latency can slow down API requests, affecting user experience. Minimize this by optimizing the backend or using caching.
- Versioning: As your API evolves, use versioning (e.g.,
/api/v1/
) to ensure that changes don’t break existing features. - Cross-Origin Requests: If the frontend and backend are hosted on different domains, you’ll need to handle CORS properly.
Conclusion: The Power of RESTful APIs
Connecting the frontend and backend using RESTful APIs is a crucial step in web development. By following best practices, handling security concerns, and optimizing your API, you can create scalable and secure applications that deliver excellent user experiences.
Ready to connect your frontend and backend? Start implementing RESTful APIs today and unlock the full potential of modern web development.
Call to Action:
Start building your own web applications today! Check out our interactive tutorials and join our developer community to learn more about RESTful APIs, authentication strategies, and advanced frontend-backend integration.
Internal/External Linking Recommendations:
- Internal: Link to articles like “How to Build a RESTful API with Node.js”, “Advanced React API Integration”.
- External: Reference official documentation like Express.js, React.js, and MDN Web Docs on HTTP methods.
- Connect frontend and backend
- RESTful API tutorial
- Frontend backend integration
- Node.js API setup
- React.js API requests
0 Comments