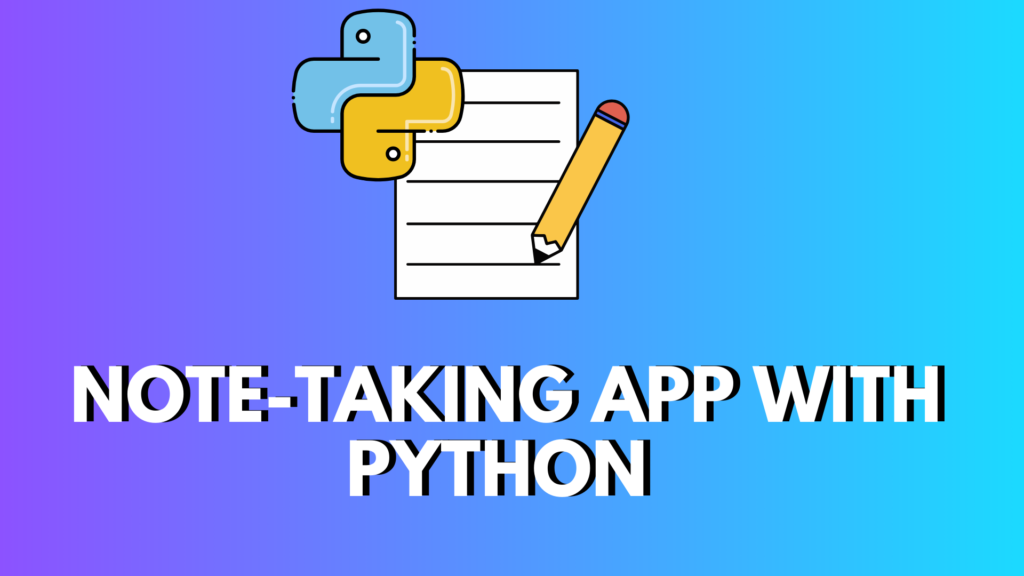
Are you looking to develop your own note-taking app source code? This step-by-step guide will help you build a fully functional note-taking app using Python (Flask) and React in 2024. By the end of this tutorial, you’ll have a working app that allows you to create, edit, and delete notes while gaining valuable experience with Flask and React.
Free Python projects with source code
- Virtual Plant Care Assistant in Python 2025
- Weather Board App in Python 2025
- Best Student Management System Python Project with Source Code 2025
- Best Humanize AI Python Code
- Discover Python Projects with Source Code: Beginner to Advanced
- Automate Login With Python 4 Source Codes
- Best Python Course for Beginners 2024
- Link Prediction in Social Networks with Python 4 Steps
- Building 1 Weather App with Python: A Comprehensive Guide
- Best AI Personal Assistant Using Python 2025
- Movie Recommendation System Using Collaborative Filtering
- Best Content Creation and Podcasting with AI: Step by Step
- Best WhatsApp Bot in Python Code: From Basic to AI-Driven
- E Commerce Customer Satisfaction: E Commerce Data Analysis Project
- Powerful Note Taking App Source Code Python Project 2024
Why Build a Note-Taking App?
A note-taking app is a great beginner-friendly project that incorporates both frontend and backend development. Whether you want to enhance your skills or create a Python project 2024 for your portfolio, this tutorial is the perfect starting point.
Setting Up the Project
Before diving into the code, let’s set up the development environment.
Step 1: Create a React App
Open your terminal and run the following commands to create a new React application:
npx create-react-app note-taking-app
cd note-taking-app
Step 2: Install Flask for Backend
Navigate to the backend directory and install Flask:
pip install Flask
Now, create a new Python file called app.py
and add the following code:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
This will start a Flask server to handle backend requests.
Building the Frontend with React
Step 3: Create Components
Inside your React app, create a src/components
directory and add two components: NoteList.js and AddNoteForm.js.
NoteList.js
import React from 'react';
const NoteList = ({ notes, onDelete }) => {
return (
<div>
<h2>Notes</h2>
<ul>
{notes.map((note) => (
<li key={note.id}>
{note.text}
<button onClick={() => onDelete(note.id)}>Delete</button>
</li>
))}
</ul>
</div>
);
};
export default NoteList;
AddNoteForm.js
import React, { useState } from 'react';
const AddNoteForm = ({ onAdd }) => {
const [newNote, setNewNote] = useState('');
const handleAddNote = () => {
if (newNote.trim() !== '') {
onAdd(newNote);
setNewNote('');
}
};
return (
<div>
<h2>Add Note</h2>
<input
type="text"
value={newNote}
onChange={(e) => setNewNote(e.target.value)}
placeholder="Enter your note"
/>
<button onClick={handleAddNote}>Add</button>
</div>
);
};
export default AddNoteForm;
Bringing It All Together
Step 4: Integrate Components in App.js
Modify App.js
to include state management for notes.
import React, { useState } from 'react';
import NoteList from './components/NoteList';
import AddNoteForm from './components/AddNoteForm';
const App = () => {
const [notes, setNotes] = useState([]);
const addNote = (text) => {
const newNote = {
id: Date.now(),
text: text,
};
setNotes([...notes, newNote]);
};
const deleteNote = (id) => {
const updatedNotes = notes.filter((note) => note.id !== id);
setNotes(updatedNotes);
};
return (
<div>
<h1>Simple Note-Taking Python Project 2024</h1>
<AddNoteForm onAdd={addNote} />
<NoteList notes={notes} onDelete={deleteNote} />
</div>
);
};
export default App;
Running the App
Step 5: Start the Flask Server
Run the following command to start the backend:
python app.py
Your Flask server should now be running at http://127.0.0.1:5000/.
Step 6: Start the React App
Run the React frontend:
npm start
Now, visit http://localhost:3000/ in your browser to see your note-taking app in action!
Expand Your Project
Now that you have a basic note-taking app, consider adding the following features:
- User Authentication
- Database Integration with SQLite or MongoDB
- Rich Text Formatting
- Dark Mode Support
Here are answers to your questions:
How do you take notes in Python?
You can take notes in Python using a simple script that writes and reads from a text file:
def write_note(filename, note):
with open(filename, "a") as file:
file.write(note + "\n")
def read_notes(filename):
with open(filename, "r") as file:
return file.readlines()
# Example usage
write_note("notes.txt", "This is my first note!")
notes = read_notes("notes.txt")
print("Your Notes:", notes)
This saves notes in a text file and retrieves them when needed.
Is there a free note-taking app?
Yes, several free note-taking apps are available, including:
- Joplin – Open-source and supports encryption
- Standard Notes – Offers a free tier with encryption
- Microsoft OneNote – Free with cloud syncing
- Google Keep – Simple and integrates with Google services
How to write Notepad in Python?
You can create a basic Notepad using Python and Tkinter:
import tkinter as tk
from tkinter import filedialog
def save_note():
text = text_area.get("1.0", tk.END)
file_path = filedialog.asksaveasfilename(defaultextension=".txt",
filetypes=[("Text Files", "*.txt")])
if file_path:
with open(file_path, "w") as file:
file.write(text)
app = tk.Tk()
app.title("Python Notepad")
text_area = tk.Text(app, wrap="word")
text_area.pack(expand=1, fill="both")
save_button = tk.Button(app, text="Save", command=save_note)
save_button.pack()
app.mainloop()
This creates a simple Notepad with a save function.
How to create an application using Python?
To create an application in Python, follow these steps:
- Choose a framework – For GUI apps, use Tkinter, PyQt, or Kivy. For web apps, use Flask or Django.
- Plan your app – Define its features and user flow.
- Write the code – Develop the backend (Python logic) and frontend (GUI or web UI).
- Test the app – Debug and refine it.
- Package & deploy – Use PyInstaller for desktop apps or a web server for web apps.
Is Python Good for App Development?
Yes, Python is great for app development, but it depends on the type of app you want to build.
✅ Good for:
- Web applications (Flask, Django)
- Data science & AI apps (TensorFlow, PyTorch)
- Automation scripts & tools
- Desktop applications (Tkinter, PyQt, Kivy)
- Backend development
❌ Not ideal for:
- Mobile apps (You can use Kivy or BeeWare, but native languages like Swift (iOS) or Kotlin (Android) are more efficient.)
- High-performance gaming apps (Python is slower than C++ or C#.)
How to Do Python Projects for Beginners?
Here are some free beginner python projects: https://darekdari.com/category/python/
If you’re a beginner, follow these steps:
1️⃣ Choose a Simple Project Idea – Start small with projects like:
- Calculator app
- To-do list app
- Weather app (using an API)
- Notepad app (Tkinter)
2️⃣ Set Up Your Environment – Install Python and an IDE (PyCharm, VS Code, or Jupyter Notebook).
3️⃣ Learn the Basics – Understand Python syntax, loops, functions, and file handling.
4️⃣ Follow Tutorials – Try small projects from online resources like Python’s official documentation, YouTube, or coding platforms.
5️⃣ Build & Experiment – Modify and add features to your projects to improve your skills.
Final Thoughts
Congratulations! 🎉 You’ve built a complete note-taking app source code using Python and React. This project is an excellent addition to your Python project 2024 portfolio and a great way to practice full-stack development.
0 Comments