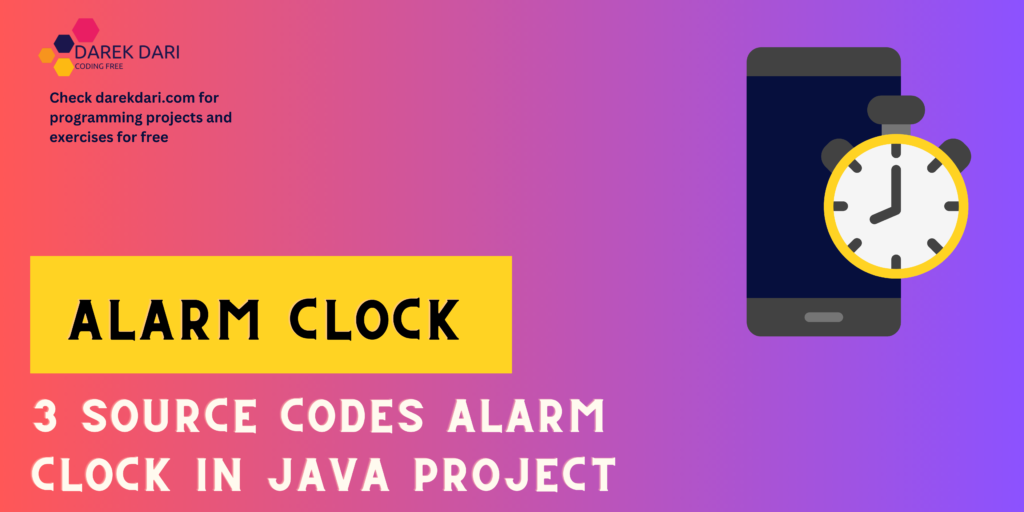
Table of Contents
Introduction for Alarm Clock in Java project
You want Alarm Clock in Java project? Imagine you have an important meeting, and you want to ensure you wake up on time. Fear not; Java has got you covered! In this article, we’ll explore three different methods to create an alarm clock in Java, each with its own approach to timely awakening.
Code 1: Using Timer and TimerTask
import java.util.Timer;
import java.util.TimerTask;
public class AlarmClock1 {
public static void main(String[] args) {
Timer timer = new Timer();
int delayInSeconds = 5; // Adjust this to set the delay for the alarm
timer.schedule(new TimerTask() {
public void run() {
System.out.println("Time's up! Alarm ringing...");
// Add your alarm action here
timer.cancel(); // Terminate the timer thread
}
}, delayInSeconds * 1000); // Convert seconds to milliseconds
}
}
This method employs the Timer
class and TimerTask
to schedule the alarm. The code sets the delay in seconds and triggers the alarm after the specified time. Perfect for a straightforward, no-fuss alarm clock. This is code 1 for alarm clocks in java.
Code 2: Using Thread and sleep
public class AlarmClock2 {
public static void main(String[] args) {
int delayInSeconds = 5; // Adjust this to set the delay for the alarm
try {
Thread.sleep(delayInSeconds * 1000); // Convert seconds to milliseconds
System.out.println("Time's up! Alarm ringing...");
// Add your alarm action here
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
In this code snippet, we utilize the Thread.sleep
method to introduce a pause in the execution, effectively creating a delay. Simple and effective, this method suits situations where a basic alarm suffices. This is code 2 for alarm clocks in java.
Code 3: Using ScheduledExecutorService
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class AlarmClock3 {
public static void main(String[] args) {
ScheduledExecutorService scheduler = Executors.newSingleThreadScheduledExecutor();
int delayInSeconds = 5; // Adjust this to set the delay for the alarm
scheduler.schedule(() -> {
System.out.println("Time's up! Alarm ringing...");
// Add your alarm action here
scheduler.shutdown(); // Shut down the scheduler
}, delayInSeconds, TimeUnit.SECONDS);
}
}
For a more sophisticated approach, Code 3 employs the ScheduledExecutorService
. It offers flexibility and control over scheduled tasks. After the alarm rings, the scheduler gracefully shuts down. This is code 3 for alarm clocks in java.
Feel free to adjust the delayInSeconds
variable in each code to set the time for the alarm to trigger. Each method provides a unique way to implement an alarm clock in Java. Experiment with them and choose the one that fits your waking-up style!
FAQ
How to make an alarm clock in Java?
import java.text.SimpleDateFormat;
import java.util.Date;
public class DigitalClock {
public static void main(String[] args) {
while (true) {
// Get the current time
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
String formattedTime = dateFormat.format(now);
// Print the time
System.out.println("Digital Clock: " + formattedTime);
try {
// Update every second
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
How to write a code for clock in Java?
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class AnalogClock {
public static void main(String[] args) {
JFrame frame = new JFrame("Analog Clock");
frame.setSize(400, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
ClockPanel clockPanel = new ClockPanel();
frame.add(clockPanel);
while (true) {
clockPanel.repaint(); // Update the clock every second
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
class ClockPanel extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Get the current time
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("hh:mm:ss");
String formattedTime = dateFormat.format(now);
// Draw clock hands and components using the Graphics object
// Example: g.drawLine(x1, y1, x2, y2) for drawing lines
// Example: g.drawOval(x, y, width, height) for drawing circles
// Adjust the coordinates and sizes based on your design
}
}
How to make digital clock in Java?
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Scanner;
public class DigitalClockWithAlarm {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Set the alarm time (HH:mm:ss): ");
String alarmTime = scanner.nextLine();
while (true) {
// Get the current time
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
String currentTime = dateFormat.format(now);
// Print the current time
System.out.println("Digital Clock: " + currentTime);
// Check if it's time for the alarm
if (alarmTime.equals(currentTime)) {
System.out.println("ALARM! Wake up!");
break; // Exit the loop after the alarm rings
}
try {
// Update every second
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
How to create an analog clock in Java?
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class AnalogClock extends JFrame {
public AnalogClock() {
setTitle("Analog Clock");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
ClockPanel clockPanel = new ClockPanel();
add(clockPanel);
// Continuously update the clock
while (true) {
clockPanel.repaint();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new AnalogClock());
}
}
class ClockPanel extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Get the current time
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("hh:mm:ss");
String formattedTime = dateFormat.format(now);
// Convert hours, minutes, and seconds to angles
int hours = Integer.parseInt(formattedTime.substring(0, 2)) % 12;
int minutes = Integer.parseInt(formattedTime.substring(3, 5));
int seconds = Integer.parseInt(formattedTime.substring(6, 8));
double angleHours = Math.toRadians((hours * 30) - 90 + (0.5 * minutes));
double angleMinutes = Math.toRadians((minutes * 6) - 90);
double angleSeconds = Math.toRadians((seconds * 6) - 90);
int centerX = getWidth() / 2;
int centerY = getHeight() / 2;
int handLength = Math.min(centerX, centerY) - 20;
// Draw clock circle
g.drawOval(centerX - handLength, centerY - handLength, handLength * 2, handLength * 2);
// Draw hour hand
drawClockHand(g, centerX, centerY, angleHours, handLength / 2, Color.BLACK);
// Draw minute hand
drawClockHand(g, centerX, centerY, angleMinutes, handLength - 10, Color.BLUE);
// Draw second hand
drawClockHand(g, centerX, centerY, angleSeconds, handLength - 10, Color.RED);
}
private void drawClockHand(Graphics g, int centerX, int centerY, double angle, int length, Color color) {
int x = (int) (centerX + length * Math.cos(angle));
int y = (int) (centerY + length * Math.sin(angle));
Graphics2D g2d = (Graphics2D) g;
g2d.setStroke(new BasicStroke(2));
g2d.setColor(color);
g2d.drawLine(centerX, centerY, x, y);
}
}
In this example, the ClockPanel
class extends JPanel
and overrides the paintComponent
method to draw the clock hands and components. The drawClockHand
method is used to draw each clock hand.
To run this program, create an instance of the AnalogClock
class. The code uses the Swing framework to create a simple GUI for the analog clock. The hands of the clock are drawn based on the current time, with different colors for each hand.
How to make a 24-hour clock in Java?
Hey there! So, you know how we usually see those round clocks with the hour, minute, and second hands? Well, I’m going to show you how to make a 24-hour version of that using Java. It’s going to be a bit like a digital clock but with a cool analog twist.
Imagine you have this clock displayed on your computer screen, and it updates every second to show you the current time. Neat, right? Let’s dive into the Java code that makes this happen.
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TwentyFourHourClock extends JFrame {
public TwentyFourHourClock() {
setTitle("24-Hour Clock");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
ClockPanel clockPanel = new ClockPanel();
add(clockPanel);
// Continuously update the clock
while (true) {
clockPanel.repaint();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new TwentyFourHourClock());
}
}
class ClockPanel extends JPanel {
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
// Get the current time in 24-hour format
Date now = new Date();
SimpleDateFormat dateFormat = new SimpleDateFormat("HH:mm:ss");
String formattedTime = dateFormat.format(now);
// Convert hours, minutes, and seconds to angles
int hours = Integer.parseInt(formattedTime.substring(0, 2));
int minutes = Integer.parseInt(formattedTime.substring(3, 5));
int seconds = Integer.parseInt(formattedTime.substring(6, 8));
double angleHours = Math.toRadians((hours % 12 + minutes / 60.0) * 30 - 90);
double angleMinutes = Math.toRadians((minutes * 6) - 90);
double angleSeconds = Math.toRadians((seconds * 6) - 90);
int centerX = getWidth() / 2;
int centerY = getHeight() / 2;
int handLength = Math.min(centerX, centerY) - 20;
// Draw clock circle
g.drawOval(centerX - handLength, centerY - handLength, handLength * 2, handLength * 2);
// Draw hour hand
drawClockHand(g, centerX, centerY, angleHours, handLength / 2, Color.BLACK);
// Draw minute hand
drawClockHand(g, centerX, centerY, angleMinutes, handLength - 10, Color.BLUE);
// Draw second hand
drawClockHand(g, centerX, centerY, angleSeconds, handLength - 10, Color.RED);
}
private void drawClockHand(Graphics g, int centerX, int centerY, double angle, int length, Color color) {
int x = (int) (centerX + length * Math.cos(angle));
int y = (int) (centerY + length * Math.sin(angle));
Graphics2D g2d = (Graphics2D) g;
g2d.setStroke(new BasicStroke(2));
g2d.setColor(color);
g2d.drawLine(centerX, centerY, x, y);
}
}
Now, if you run this Java program, you’ll get this cool 24-hour clock displayed on your screen. The clock hands move in real-time, just like a regular clock, but in a 24-hour format. You can totally play around with the code, customize it, and make it your own.
Conclusion
Feel free to customize and enhance this example based on your preferences and requirements. You can add additional features such as a background image, different hand styles, or interactive elements. Happy coding!
In case you have any questions or need further clarification, don’t hesitate to reach out. Happy coding, and may you wake up to success with these alarm clocks in java!
1 Comment
Best Medium E-commerce Platform Java Source Code 2024 · May 20, 2024 at 10:00 pm
[…] Alarm Clock: Sure thing! Need an Alarm Clock for your Java project? Picture this: you have a crucial meeting coming up, and you want to make sure you wake up on time. No worries; Java has your back! In this post, we will discuss three unique ways to develop an alarm clock in Java, each offering a distinct method to ensure you wake up promptly. […]