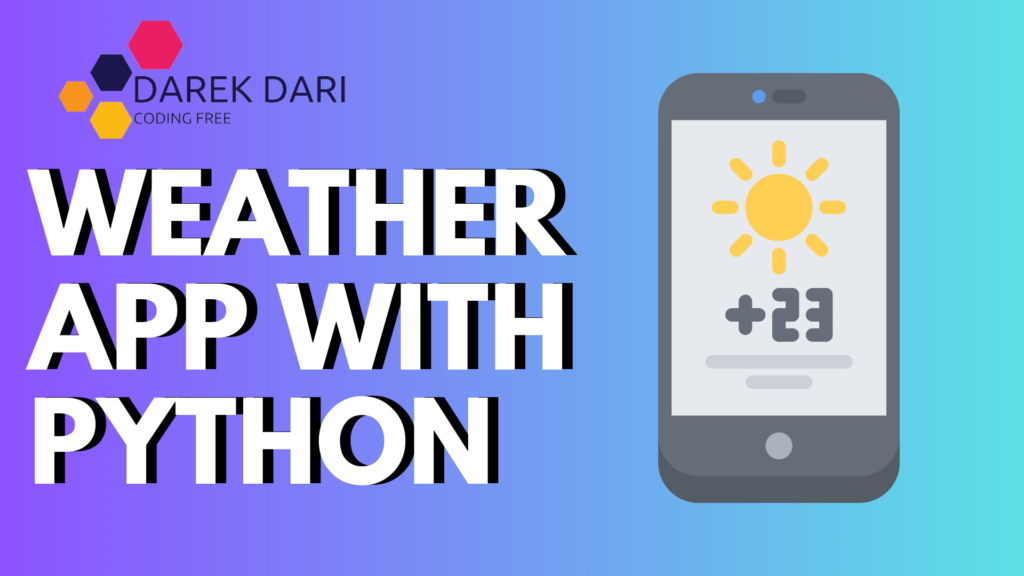
Table of Contents
Introduction
Hey there, coding enthusiasts! 🌞 Have you ever wanted to create a weather app that fetches real-time data? Well, today is your lucky day! We’ll be building a weather application using Python and the OpenWeatherMap API.
Not only will it display the current weather, but it will also provide a 5-day forecast and save the data to files. Ready? Let’s dive in!
weather app
app weather
weather apps
nyc weather today
Prerequisites For the Weather App with Python
Before we start, make sure you have:
- Python 3.x installed on your machine.
- An API key from OpenWeatherMap. You can get it by signing up here.
Project Structure
Here’s how we’ll organize our project:
weather_app/
│
├── main.py
├── forecast.py
├── utils.py
└── README.md
Step-by-Step Guide
Setting Up the Environment
First, create a directory for your project:
mkdir weather_app
cd weather_app
Next, create the necessary files:
touch main.py forecast.py utils.py README.md
Writing the Code
main.py
This will be our main script to run the application.
import utils
from forecast import get_forecast
def main():
api_key = "your_openweathermap_api_key"
city_name = input("Enter city name: ")
# Get current weather
weather_data = utils.get_weather(city_name, api_key)
if weather_data:
utils.display_weather(weather_data)
# Save current weather data to file
utils.save_weather_data(weather_data, f"{city_name}_current_weather.json")
# Get weather forecast
forecast_data = get_forecast(city_name, api_key)
if forecast_data:
utils.display_forecast(forecast_data)
# Save forecast data to file
utils.save_weather_data(forecast_data, f"{city_name}_weather_forecast.json")
else:
print("Failed to retrieve weather data. Please check the city name and try again.")
if __name__ == "__main__":
main()
Explanation:
Code Snippet | Explanation |
---|---|
import utils | Imports the utils module which contains utility functions for fetching, displaying, and saving weather data. |
from forecast import get_forecast | Imports the get_forecast function from the forecast module. |
def main(): | Defines the main function that will execute when the script is run. |
api_key = "your_openweathermap_api_key" | Stores the OpenWeatherMap API key in a variable. |
city_name = input("Enter city name: ") | Prompts the user to enter the name of the city for which they want to fetch the weather data. |
weather_data = utils.get_weather(city_name, api_key) | Calls the get_weather function to fetch the current weather data for the specified city. |
if weather_data: | Checks if the weather data was successfully retrieved. |
utils.display_weather(weather_data) | Calls the display_weather function to print the current weather data. |
utils.save_weather_data(weather_data, f"{city_name}_current_weather.json") | Saves the current weather data to a JSON file. |
forecast_data = get_forecast(city_name, api_key) | Calls the get_forecast function to fetch the 5-day weather forecast. |
if forecast_data: | Checks if the forecast data was successfully retrieved. |
utils.display_forecast(forecast_data) | Calls the display_forecast function to print the weather forecast data. |
utils.save_weather_data(forecast_data, f"{city_name}_weather_forecast.json") | Saves the forecast data to a JSON file. |
else: | Executes if the weather data could not be retrieved, indicating an error with the city name input. |
print("Failed to retrieve weather data. Please check the city name and try again.") | Prints an error message if the weather data could not be retrieved. |
if __name__ == "__main__": | Ensures that the main function is executed only when the script is run directly, not when imported as a module. |
main() | Calls the main function to execute the script. |
apple valley weather
weather-apple valley
new york city current weather
nyc weather news
best app weather iphone
free weather apps
apple valley weather mn
weather forecast apple valley mn
apple valley minnesota weather
best weather app for iphone
utils.py
This file will contain utility functions for fetching, displaying, and saving weather data.
import requests
import json
def get_weather(city_name, api_key):
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = base_url + "q=" + city_name + "&appid=" + api_key + "&units=metric"
response = requests.get(complete_url)
if response.status_code == 200:
return response.json()
else:
print(f"Error {response.status_code}: {response.reason}")
return None
def display_weather(data):
if data["cod"] != "404":
main = data["main"]
weather = data["weather"][0]
wind = data["wind"]
print(f"\nCurrent Weather in {data['name']}:")
print(f"Temperature: {main['temp']}°C")
print(f"Weather: {weather['description']}")
print(f"Humidity: {main['humidity']}%")
print(f"Wind Speed: {wind['speed']} m/s\n")
else:
print("City not found. Please check the name and try again.")
def save_weather_data(data, filename):
with open(filename, 'w') as file:
json.dump(data, file, indent=4)
print(f"Weather data saved to {filename}")
def display_forecast(data):
print("\nWeather Forecast:")
for item in data["list"]:
dt_txt = item["dt_txt"]
main = item["main"]
weather = item["weather"][0]
wind = item["wind"]
print(f"Date and Time: {dt_txt}")
print(f"Temperature: {main['temp']}°C")
print(f"Weather: {weather['description']}")
print(f"Humidity: {main['humidity']}%")
print(f"Wind Speed: {wind['speed']} m/s\n")
Explanation:
Code Snippet | Explanation |
---|---|
import requests | Imports the requests library to handle HTTP requests to the OpenWeatherMap API. |
import json | Imports the json library to handle JSON data serialization and deserialization. |
def get_weather(city_name, api_key): | Defines the get_weather function to fetch current weather data for a given city using the OpenWeatherMap API. |
base_url = "http://api.openweathermap.org/data/2.5/weather?" | Sets the base URL for the OpenWeatherMap weather API. |
complete_url = base_url + "q=" + city_name + "&appid=" + api_key + "&units=metric" | Constructs the complete URL with the city name, API key, and metric units. |
response = requests.get(complete_url) | Sends an HTTP GET request to the complete URL and stores the response. |
if response.status_code == 200: | Checks if the response status code is 200 (OK). |
return response.json() | Returns the response data in JSON format if the status code is 200. |
print(f"Error {response.status_code}: {response.reason}") | Prints an error message if the status code is not 200. |
return None | Returns None if the status code is not 200. |
def display_weather(data): | Defines the display_weather function to print the current weather data in a readable format. |
if data["cod"] != "404": | Checks if the city was found in the weather data. |
main = data["main"] | Extracts the main weather data. |
weather = data["weather"][0] | Extracts the weather description. |
wind = data["wind"] | Extracts the wind data. |
print(f"Current Weather in {data['name']}:") | Prints the city name. |
print(f"Temperature: {main['temp']}°C") | Prints the temperature. |
print(f"Weather: {weather['description']}") | Prints the weather description. |
print(f"Humidity: {main['humidity']}%") | Prints the humidity. |
print(f"Wind Speed: {wind['speed']} m/s") | Prints the wind speed. |
else: | Executes if the city was not found in the weather data. |
print("City not found. Please check the name and try again.") | Prints an error message if the city was not found. |
def save_weather_data(data, filename): | Defines the |
weather app python
weather app
best app weather iphone
best weather app iphone
free weather apps
weather app free
weather apps free
apps weather free
forecast.py
This file will handle fetching the 5-day weather forecast.
import requests
def get_forecast(city_name, api_key):
base_url = "http://api.openweathermap.org/data/2.5/forecast?"
complete_url = base_url + "q=" + city_name + "&appid=" + api_key + "&units=metric"
response = requests.get(complete_url)
if response.status_code == 200:
return response.json()
else:
print(f"Error {response.status_code}: {response.reason}")
return None
Explanation:
Code Snippet | Explanation |
---|---|
import requests | Imports the requests library to handle HTTP requests to the OpenWeatherMap API. |
def get_forecast(city_name, api_key): | Defines the get_forecast function to fetch the 5-day weather forecast for a given city using the OpenWeatherMap API. |
base_url = "http://api.openweathermap.org/data/2.5/forecast?" | Sets the base URL for the OpenWeatherMap forecast API. |
complete_url = base_url + "q=" + city_name + "&appid=" + api_key + "&units=metric" | Constructs the complete URL with the city name, API key, and metric units. |
response = requests.get(complete_url) | Sends an HTTP GET request to the complete URL and stores the response. |
if response.status_code == 200: | Checks if the response status code is 200 (OK). |
return response.json() | Returns the response data in JSON format if the status code is 200. |
print(f"Error {response.status_code}: {response.reason}") | Prints an error message if the status code is not 200. |
return None | Returns None if the status code is not 200. |
best free weather app
best weather app free
weather app android free
free weather app for android phone
best free weather app for iphone
best free weather app iphone
best free weather apps for iphone
free weather apps for iphone
Running the Project
Now that we have all the code ready, let’s run our weather app!
Clone the repository:
git clone https://github.com/yourusername/weather_app.git
cd weather_app
Install the required libraries:
pip install requests
Replace your_openweathermap_api_key
in main.py
with your actual API key.
Run the application:
python main.py
python weather api
python-weather-api
python weather
python-weather
weather api python
weather python
python weather app
what is best free weather app for iphone
is the weather app free
python weather forecast
Enhanced Features
Fetching Current Weather Data
To retrieve the current weather data for a specific city, we utilize the OpenWeatherMap API. The get_weather
function is responsible for constructing the appropriate API request and fetching the data.
It creates a URL using the base endpoint, city name, API key, and unit of measurement (metric for Celsius). After sending a GET request to the API, the function checks if the response status code is 200 (indicating success). If successful, it returns the weather data in JSON format; otherwise, it prints an error message.
Here’s the code for fetching current weather data:
import requests
def get_weather(city_name, api_key):
"""Fetches current weather data for a given city."""
base_url = "http://api.openweathermap.org/data/2.5/weather?"
complete_url = f"{base_url}q={city_name}&appid={api_key}&units=metric"
response = requests.get(complete_url)
if response.status_code == 200:
return response.json()
else:
print(f"Error {response.status_code}: {response.reason}")
return None
def display_weather(data):
"""Displays current weather information in a user-friendly format."""
if data and data.get("cod") != "404":
main = data.get("main", {})
weather = data.get("weather", [{}])[0]
wind = data.get("wind", {})
print(f"\nCurrent Weather in {data['name']}:")
print(f"Temperature: {main.get('temp')}°C")
print(f"Weather: {weather.get('description')}")
print(f"Humidity: {main.get('humidity')}%")
print(f"Wind Speed: {wind.get('speed')} m/s\n")
else:
print("City not found. Please check the name and try again.")
The display_weather
function extracts and prints the relevant weather information such as temperature, weather description, humidity, and wind speed in a user-friendly format. It checks if the data is valid and if the city was found before displaying the details.
Fetching the 5-Day Weather Forecast
The get_forecast
function is designed to fetch a 5-day weather forecast using the OpenWeatherMap API’s forecast endpoint. Similar to the current weather function, it constructs a URL with the city name, API key, and metric units.
After sending a GET request, it checks if the status code is 200. If the request is successful, it returns the forecast data in JSON format. If not, it prints an error message.
Here’s the code for fetching and displaying the 5-day weather forecast:
import requests
def get_forecast(city_name, api_key):
"""Fetches 5-day weather forecast for a given city."""
base_url = "http://api.openweathermap.org/data/2.5/forecast?"
complete_url = f"{base_url}q={city_name}&appid={api_key}&units=metric"
response = requests.get(complete_url)
if response.status_code == 200:
return response.json()
else:
print(f"Error {response.status_code}: {response.reason}")
return None
def display_forecast(data):
"""Displays 5-day weather forecast in a user-friendly format."""
if data and data.get("cod") == "200":
print("\nWeather Forecast:")
for item in data.get("list", []):
dt_txt = item.get("dt_txt")
main = item.get("main", {})
weather = item.get("weather", [{}])[0]
wind = item.get("wind", {})
print(f"Date and Time: {dt_txt}")
print(f"Temperature: {main.get('temp')}°C")
print(f"Weather: {weather.get('description')}")
print(f"Humidity: {main.get('humidity')}%")
print(f"Wind Speed: {wind.get('speed')} m/s\n")
else:
print("Forecast data not available. Please try again later.")
The display_forecast
function processes the forecast data and prints out the date and time, temperature, weather description, humidity, and wind speed for each forecast entry. It provides a clear and organized view of the upcoming weather conditions.
Saving Weather Data to Files
To save the fetched weather data for later use, we utilize the save_weather_data
function. This function takes the weather data and a filename as arguments, writes the data to a JSON file, and prints a confirmation message once the data is saved successfully.
Here’s the code for saving weather data:
import json
def save_weather_data(data, filename):
"""Saves weather data to a JSON file."""
with open(filename, 'w') as file:
json.dump(data, file, indent=4)
print(f"Weather data saved to {filename}")
This function ensures that the weather data can be preserved and reviewed later, providing a reliable way to store and access historical weather information.
Handling Errors Gracefully
In the provided main
function, error handling is integrated to ensure that users receive appropriate feedback if data retrieval fails.
The function prompts the user for a city name, fetches current weather data, displays it, saves it to a file, and then fetches and displays the 5-day weather forecast. If any part of this process encounters an issue, such as an invalid city name or a failed API request, the function prints an informative error message.
Here’s the complete main
function:
from current_weather import get_weather, display_weather
from forecast import get_forecast, display_forecast
from save_data import save_weather_data
def main():
api_key = "your_openweathermap_api_key"
city_name = input("Enter city name: ")
# Get current weather
weather_data = get_weather(city_name, api_key)
if weather_data:
display_weather(weather_data)
save_weather_data(weather_data, f"{city_name}_current_weather.json")
# Get weather forecast
forecast_data = get_forecast(city_name, api_key)
if forecast_data:
display_forecast(forecast_data)
save_weather_data(forecast_data, f"{city_name}_weather_forecast.json")
else:
print("Failed to retrieve weather data. Please check the city name and try again.")
if __name__ == "__main__":
main()
This function serves as the central point of the application, orchestrating the various tasks and ensuring that errors are communicated effectively to the user.
Fetching Weather Data
We use the requests
library to send HTTP requests to the OpenWeatherMap API. The get_weather
function constructs the request URL, sends the request, and returns the response in JSON format. If there’s an error, it prints an error message.
Displaying Weather Data
The display_weather
and display_forecast
functions format and print the weather data to the console. They provide a clear and readable output for the user.
Saving Weather Data
The save_weather_data
function saves the weather data to a JSON file. This way, users can keep a record of the weather data for future reference.
weather forecast python
openweathermap api python
python openweather
weather py
weather api python github
python weather api example
how to get current weather in python
openweathermap api python example
openweathermap python example
python weather script
Conclusion
Congratulations! 🎉 You’ve built a weather application using Python and the OpenWeatherMap API. This project not only helps you understand how to work with APIs but also demonstrates how to structure a Python project, handle errors, and save data to files.
Feel free to enhance this project further by adding features like a graphical user interface (GUI) or integrating more weather details. Happy coding! 😊
If you have any questions or need further assistance, drop a comment below. I’d love to help you out! 👇
application météo
app météo
météo app
météo application
application meteo
télécharger météo
télécharger application météo gratuite
télécharger météo google
télécharger météo gratuitement
FAQ
Q: How can I get an OpenWeatherMap API key?
A: You can get an API key by signing up on the OpenWeatherMap website here.
Q: Can I use this app for other weather-related projects?
A: Absolutely! This app provides a great foundation. You can extend it to include features like severe weather alerts, historical weather data, or even a mobile app version.
Keep coding, keep learning, and keep exploring! 🌟
python weather api
python-weather-api
python weather
python-weather
weather api python
weather python
python weather app
weather app python
python meteorology
python weather forecast
weather forecast python
weather py
0 Comments