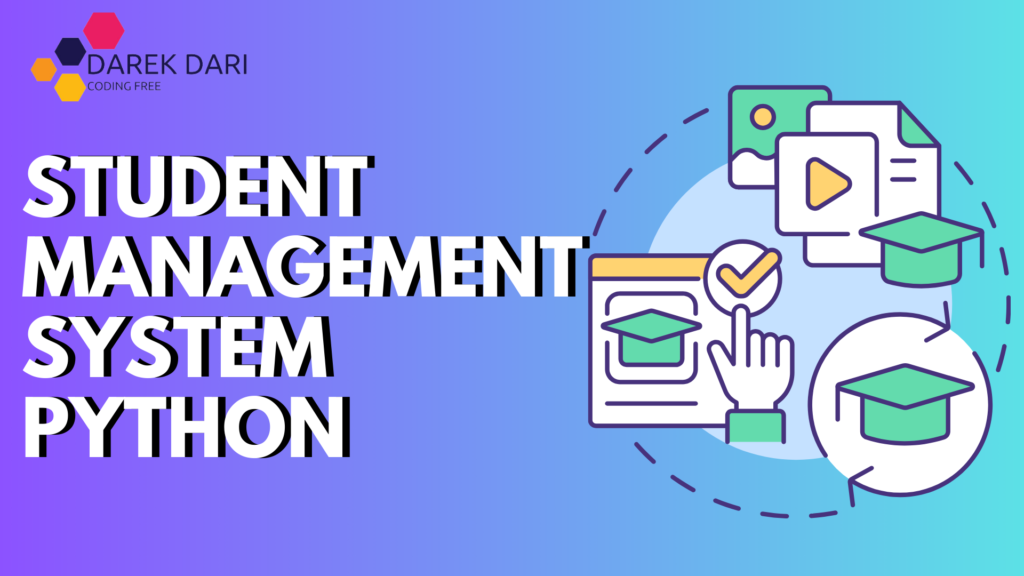
Table of Contents
Managing student records efficiently is a common challenge for educational institutions. Whether you’re running a small tutoring business or overseeing a large school, keeping track of student data like names, grades, and ages can quickly become overwhelming. In this blog, we’ll explore how to create a Student Management System in Python that’s easy to use, scalable, and equipped with cool features like exporting and importing data.
Why Build a Student Management System?
Let’s face it—managing student information manually can be a headache. A student management system is essential for:
- Quick Data Access: View and update student records without shuffling through papers.
- Data Organization: Keep everything neat and secure.
- Ease of Use: Automate repetitive tasks like adding or searching for students.
Python makes it easy to build such systems thanks to its simplicity and vast libraries. Ready to dive in? Let’s go!
Student Management System in Python
Python projects for beginners
managing student data in Python
Features of Our Student Management System
Here’s what our system can do:
- Add Students: Add new student records with unique IDs.
- View Students: See a list of all students in the system.
- Update Students: Modify student details like name, age, or grade.
- Delete Students: Remove students by their ID.
- Search Students: Find a student’s details using their ID.
- Export Data: Save student data to a file for backup or sharing.
- Import Data: Load student data from a file.
These features make it an ideal Student Management System in Python for beginners and professionals alike.
The Code
Below is the complete Python implementation of our Student Management System:
import json
class StudentManagementSystem:
def __init__(self):
self.students = {}
def add_student(self, student_id, name, age, grade):
if student_id in self.students:
print("Student ID already exists.")
return
self.students[student_id] = {
'name': name,
'age': int(age),
'grade': grade.upper()
}
print(f"Student {name} added successfully.")
def view_students(self):
if not self.students:
print("No students in the system.")
return
print("\nStudent List:")
for student_id, details in self.students.items():
print(f"ID: {student_id}, Name: {details['name']}, Age: {details['age']}, Grade: {details['grade']}")
def update_student(self, student_id, name=None, age=None, grade=None):
if student_id not in self.students:
print("Student ID not found.")
return
if name:
self.students[student_id]['name'] = name
if age:
self.students[student_id]['age'] = int(age)
if grade:
self.students[student_id]['grade'] = grade.upper()
print(f"Student {student_id} updated successfully.")
def delete_student(self, student_id):
if student_id not in self.students:
print("Student ID not found.")
return
removed_student = self.students.pop(student_id)
print(f"Student {removed_student['name']} deleted successfully.")
def search_student(self, student_id):
if student_id not in self.students:
print("Student ID not found.")
return
details = self.students[student_id]
print(f"Student Details: ID: {student_id}, Name: {details['name']}, Age: {details['age']}, Grade: {details['grade']}")
def export_students(self, filename):
try:
with open(filename, 'w') as file:
json.dump(self.students, file, indent=4)
print(f"Student data exported to {filename}")
except Exception as e:
print(f"Failed to export data: {e}")
def import_students(self, filename):
try:
with open(filename, 'r') as file:
self.students = json.load(file)
print(f"Student data imported from {filename}")
except Exception as e:
print(f"Failed to import data: {e}")
# Main Program
if __name__ == "__main__":
system = StudentManagementSystem()
# Predefined inputs for testing in non-interactive environments
predefined_inputs = [
'1', '101', 'Alice', '20', 'A',
'1', '102', 'Bob', '22', 'B',
'2',
'3', '101', 'Alicia', '21', '',
'2',
'4', '102',
'2',
'7', 'students.json',
'8', 'students.json',
'2',
'6'
]
input_iterator = iter(predefined_inputs)
def mock_input(prompt):
try:
return next(input_iterator)
except StopIteration:
raise Exception("No more predefined inputs available.")
input = mock_input
while True:
print("\nStudent Management System")
print("1. Add Student")
print("2. View Students")
print("3. Update Student")
print("4. Delete Student")
print("5. Search Student")
print("6. Exit")
print("7. Export Students to File")
print("8. Import Students from File")
choice = input("Enter your choice: ")
if choice == '1':
student_id = input("Enter student ID: ")
name = input("Enter student name: ")
age = input("Enter student age: ")
grade = input("Enter student grade: ")
system.add_student(student_id, name, age, grade)
elif choice == '2':
system.view_students()
elif choice == '3':
student_id = input("Enter student ID to update: ")
name = input("Enter new name (leave blank to keep unchanged): ")
age = input("Enter new age (leave blank to keep unchanged): ")
grade = input("Enter new grade (leave blank to keep unchanged): ")
system.update_student(student_id, name or None, age or None, grade or None)
elif choice == '4':
student_id = input("Enter student ID to delete: ")
system.delete_student(student_id)
elif choice == '5':
student_id = input("Enter student ID to search: ")
system.search_student(student_id)
elif choice == '6':
print("Exiting the program.")
break
elif choice == '7':
filename = input("Enter filename to export data: ")
system.export_students(filename)
elif choice == '8':
filename = input("Enter filename to import data: ")
system.import_students(filename)
else:
print("Invalid choice. Please try again.")
Student management system python github
Student management system python pdf
Student management system python example
Student Management System project in Python with source code
Student management system python geeksforgeeks
Student Management System project with source code
Library Management System project in Python
Student record Management System frontend Python and backend dbms
Example Workflow
Here’s how you can use the system:
- Adding Students:
- Input: ID
101
, NameAlice
, Age20
, GradeA
. - Output: “Student Alice added successfully.”
- Input: ID
- Viewing Students:
- Input: View all students.
- Output: A neat list of student records.
- Updating Students:
- Input: ID
101
, New NameAlicia
, New Age21
. - Output: “Student 101 updated successfully.”
- Input: ID
- Exporting Data:
- Input: Export to
students.json
. - Output: “Student data exported to students.json.”
- Input: Export to
- Importing Data:
- Input: Import from
students.json
. - Output: “Student data imported from students.json.”
- Input: Import from
This practical example showcases the power of Python projects for beginners and demonstrates managing student data in Python effectively.
What’s Next?
This Student Management System in Python is a great starting point, but there’s always room to grow. Here are some ideas:
- Add a Web Interface: Use Flask or Django to make it accessible online.
- Advanced Search: Allow searching by name, age range, or grade.
- Data Visualization: Create graphs to analyze student performance.
- Cloud Storage: Store data in the cloud for better scalability.
Conclusion
Congratulations! You’ve just built a fully functional Student Management System in Python. It’s simple, effective, and can handle the most common student record management tasks. Plus, it’s easy to extend with new features.
Feel free to tweak the code, make it your own, and share your improvements. Happy coding!
0 Comments