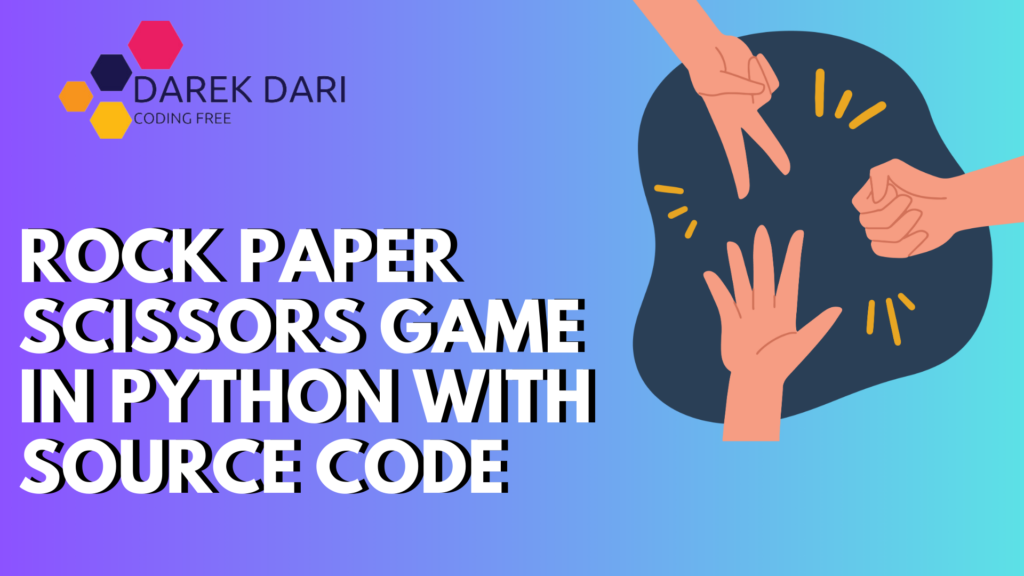
Table of Contents
Have you ever wanted to bring the classic game of Rock Paper Scissors to life with your Python coding skills? Look no further!
Today, we’re going to dive into creating a Rock Paper Scissors game—a simple, yet exciting project that’s perfect for both beginners and seasoned coders. Ready to get started? Let’s jump right in!
In this project, we’ll create a Python script that allows you to play this game against the computer. Along the way, you’ll get to practice essential programming concepts like conditionals, loops, and user input.
What Is Rock Paper Scissors?
Rock Paper Scissors is a timeless game that everyone knows and loves. The rules are simple: Rock crushes Scissors, Scissors cut Paper, and Paper covers Rock.
In the classic game of Rock, Paper, Scissors, two players simultaneously choose one of three symbols: rock, paper, or scissors. Rock beats scissors, scissors beat paper by cutting it, and paper beats rock by covering it.
Setting Up the Game
First, we’ll set up the basic structure of our game. We’ll need to import the random
module to let the computer make random choices, and define a function to get the computer’s move. Here’s a simple way to do it:
import random
def get_computer_choice():
choices = ['rock', 'paper', 'scissors']
return random.choice(choices)
Getting the Player’s Choice
Next, we’ll create a function to get the player’s choice. We’ll use a simple input prompt and make sure to handle invalid inputs gracefully:
def get_player_choice():
choice = input("Enter your choice (rock, paper, or scissors): ").lower()
while choice not in ['rock', 'paper', 'scissors']:
choice = input("Invalid choice. Please enter rock, paper, or scissors: ").lower()
return choice
Determining the Winner
Now that we have both the player’s and the computer’s choices, we need to determine the winner. We’ll create a function that takes both choices as input and returns the result:
def determine_winner(player, computer):
if player == computer:
return "It's a tie!"
elif (player == 'rock' and computer == 'scissors') or \
(player == 'scissors' and computer == 'paper') or \
(player == 'paper' and computer == 'rock'):
return "You win!"
else:
return "You lose!"
Playing the Game
With our functions in place, we can now create the main loop of our game. This loop will continue to run until the player decides to quit:
def play_game():
print("Welcome to Rock Paper Scissors!")
while True:
player_choice = get_player_choice()
computer_choice = get_computer_choice()
print(f"Computer chose: {computer_choice}")
result = determine_winner(player_choice, computer_choice)
print(result)
play_again = input("Do you want to play again? (yes or no): ").lower()
if play_again != 'yes':
break
print("Thanks for playing!")
Running the Game
Finally, we’ll run our game by calling the play_game
function:
if __name__ == "__main__":
play_game()
Enhancing the Game
Want to make your Rock Paper Scissors game even more exciting? Here are a few ideas to spice things up:
Add More Choices
Introduce additional choices like “lizard” and “spock” to create a Rock Paper Scissors Lizard Spock game, which adds more variety and complexity to the gameplay. Here’s an example of how you can expand the choices:
def get_computer_choice():
choices = ['rock', 'paper', 'scissors', 'lizard', 'spock']
return random.choice(choices)
def determine_winner(player, computer):
if player == computer:
return "It's a tie!"
elif (player == 'rock' and computer in ['scissors', 'lizard']) or \
(player == 'scissors' and computer in ['paper', 'lizard']) or \
(player == 'paper' and computer in ['rock', 'spock']) or \
(player == 'lizard' and computer in ['spock', 'paper']) or \
(player == 'spock' and computer in ['scissors', 'rock']):
return "You win!"
else:
return "You lose!"
Implement a Score System
Track the player’s and computer’s scores across multiple rounds to add a competitive edge to the game. Here’s how you can implement a score system:
def play_game():
print("Welcome to Rock Paper Scissors!")
player_score = 0
computer_score = 0
while True:
player_choice = get_player_choice()
computer_choice = get_computer_choice()
print(f"Computer chose: {computer_choice}")
result = determine_winner(player_choice, computer_choice)
print(result)
if "win" in result:
player_score += 1
elif "lose" in result:
computer_score += 1
print(f"Score: Player {player_score} - Computer {computer_score}")
play_again = input("Do you want to play again? (yes or no): ").lower()
if play_again != 'yes':
break
print("Thanks for playing!")
Create a GUI
Use libraries like Tkinter to create a graphical user interface, making the game more visually appealing and user-friendly. Here’s a simple example of how you can start with Tkinter:
import tkinter as tk
from tkinter import messagebox
def get_computer_choice():
choices = ['rock', 'paper', 'scissors']
return random.choice(choices)
def determine_winner(player, computer):
if player == computer:
return "It's a tie!"
elif (player == 'rock' and computer == 'scissors') or \
(player == 'scissors' and computer == 'paper') or \
(player == 'paper' and computer == 'rock'):
return "You win!"
else:
return "You lose!"
def play():
player_choice = player_choice_var.get()
computer_choice = get_computer_choice()
result = determine_winner(player_choice, computer_choice)
messagebox.showinfo("Result", f"Computer chose: {computer_choice}\n{result}")
root = tk.Tk()
root.title("Rock Paper Scissors")
tk.Label(root, text="Choose your move:").pack()
player_choice_var = tk.StringVar()
player_choice_var.set("rock")
choices = ['rock', 'paper', 'scissors']
for choice in choices:
tk.Radiobutton(root, text=choice.capitalize(), variable=player_choice_var, value=choice).pack()
tk.Button(root, text="Play", command=play).pack()
root.mainloop()
How AI is Used in Rock-Paper-Scissors?
Rock-Paper-Scissors (RPS) may seem like a simple game of chance, but when AI enters the scene, it becomes a fascinating playground for algorithms and strategic thinking. Let’s explore how artificial intelligence can be applied to enhance and even master this classic game.
Basic Concept
In Rock-Paper-Scissors, two players simultaneously choose one of three options: rock, paper, or scissors. The outcomes are straightforward: rock crushes scissors, scissors cut paper, and paper covers rock. The simplicity of the game makes it an ideal candidate for studying pattern recognition, prediction, and strategy in AI.
AI Strategies in RPS
Random Strategy
At its core, an AI can start by mimicking a basic strategy: choosing rock, paper, or scissors at random. This ensures a fair game, as each choice has an equal probability of 1/3. Here’s a simple Python implementation of a random strategy:
import random
def get_random_choice():
choices = ['rock', 'paper', 'scissors']
return random.choice(choices)
While this approach is unbiased, it doesn’t leverage the power of AI to anticipate and counter the opponent’s moves.
Pattern Recognition
Human players often fall into patterns, consciously or subconsciously. An AI can exploit these tendencies by analyzing the opponent’s previous moves and predicting future choices. For example, if an opponent tends to choose rock after paper, the AI can counter with paper.
def get_pattern_choice(history):
if len(history) < 2:
return get_random_choice()
if history[-1] == 'rock' and history[-2] == 'paper':
return 'paper'
return get_random_choice()
By keeping track of the opponent’s move history, the AI can start identifying patterns and making informed decisions.
Machine Learning Models
For a more sophisticated approach, machine learning algorithms can be employed. By training a model on a large dataset of RPS games, the AI can learn to predict the opponent’s next move with higher accuracy. Techniques such as neural networks or reinforcement learning can be applied here.
from sklearn.neural_network import MLPClassifier
import numpy as np
# Example training data (features: opponent's previous move, outcome; labels: AI's move)
X = np.array([[0, 1], [1, 2], [2, 0]]) # 0: rock, 1: paper, 2: scissors
y = np.array([1, 2, 0]) # AI's moves
model = MLPClassifier(max_iter=1000)
model.fit(X, y)
def get_ml_choice(previous_move, outcome):
if previous_move is None or outcome is None:
return get_random_choice()
input_data = np.array([[previous_move, outcome]])
predicted_move = model.predict(input_data)[0]
return ['rock', 'paper', 'scissors'][predicted_move]
This approach leverages the power of data and learning algorithms to adapt and improve over time.
Game Theory and Nash Equilibrium
Game theory offers another interesting perspective on RPS. The Nash Equilibrium for RPS dictates that each player should randomize their choices to prevent being exploited. An AI can use this theory to adjust its strategy dynamically, ensuring it remains unpredictable and competitive.
def get_game_theory_choice():
choices = ['rock', 'paper', 'scissors']
probabilities = [1/3, 1/3, 1/3]
return np.random.choice(choices, p=probabilities)
By understanding and applying game theory principles, the AI can achieve a balanced and optimal strategy.
Incorporating AI into Rock-Paper-Scissors transforms a simple game into a complex and intriguing challenge. From basic random strategies to advanced machine learning models, AI can analyze patterns, predict moves, and even apply game theory to outsmart human opponents. Whether for educational purposes or sheer fun, exploring AI in RPS is a fantastic way to delve into the world of artificial intelligence and strategy.
So, next time you play Rock-Paper-Scissors, remember: you might just be facing an AI opponent that’s analyzing your every move!
Conclusion
Creating a Rock Paper Scissors game in Python is a fantastic way to practice coding and have fun at the same time.
Whether you’re a beginner or an experienced coder, this project helps you hone your skills in handling user input, implementing logic with conditionals, and using loops effectively.
Plus, with a few enhancements, you can take the game to the next level and create an even more engaging experience. So, what are you waiting for? Start coding and enjoy the game!
0 Comments