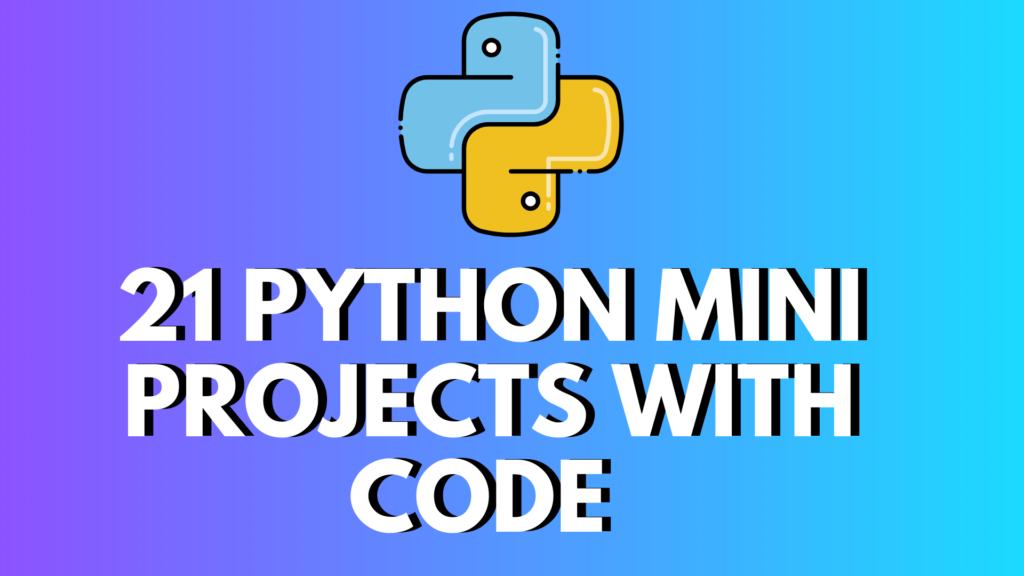
Introduction
Looking to sharpen your Python skills? Whether you’re a beginner or an experienced developer, hands-on practice is the best way to master Python.
In this article, we present 21 Python mini projects with code that you can use for learning, portfolio building, or even final-year projects. Each project includes source code and explanations to help you understand key concepts. Plus, we’ll guide you on where to find Python mini projects with source code (PDF & GitHub links) to accelerate your learning journey.
21 python mini projects with code
advanced python projects with source code
21 python mini projects with code github
Python mini projects with source code
21 python mini projects with code pdf
Python projects with source code for final year
21 python mini projects with code pdf download
Python projects with source code PDF
Python projects with source code GitHub
Python projects for students
Why Work on Python Mini Projects?
Mini projects offer a practical way to:
- Reinforce Python fundamentals.
- Develop problem-solving skills.
- Build an impressive portfolio for job applications.
- Prepare for coding interviews.
- Work on real-world applications with manageable complexity.
21 Python Mini Projects with Code
Below are 21 exciting Python mini projects. Each project includes a brief description and its source code.
1. To-Do List App
tasks = []
def add_task(task):
tasks.append(task)
print("Task added!")
def show_tasks():
for i, task in enumerate(tasks, 1):
print(f"{i}. {task}")
add_task("Learn Python")
show_tasks()
2. Random Password Generator
import random
import string
def generate_password(length=12):
characters = string.ascii_letters + string.digits + string.punctuation
return ''.join(random.choice(characters) for _ in range(length))
print("Generated Password:", generate_password())
3. Weather App Using API
import requests
API_KEY = "your_api_key_here"
city = "New York"
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={API_KEY}&units=metric"
response = requests.get(url)
weather_data = response.json()
print(f"Temperature in {city}: {weather_data['main']['temp']}°C")
4. Hangman Game
import random
words = ['python', 'java', 'kotlin', 'javascript']
word = random.choice(words)
guessed = ['_'] * len(word)
attempts = 6
while attempts > 0 and '_' in guessed:
guess = input("Guess a letter: ")
if guess in word:
for i, letter in enumerate(word):
if letter == guess:
guessed[i] = guess
else:
attempts -= 1
print(' '.join(guessed))
print("You won!" if '_' not in guessed else "You lost!")
5. Simple Calculator
def calculator():
try:
num1 = float(input("Enter first number: "))
operator = input("Enter operator (+, -, *, /): ")
num2 = float(input("Enter second number: "))
if operator == '+':
print(f"Result: {num1 + num2}")
elif operator == '-':
print(f"Result: {num1 - num2}")
elif operator == '*':
print(f"Result: {num1 * num2}")
elif operator == '/':
print(f"Result: {num1 / num2}")
else:
print("Invalid operator!")
except ValueError:
print("Invalid input! Please enter numbers.")
calculator()
6. Number Guessing Game
import random
number = random.randint(1, 100)
guess = None
while guess != number:
guess = int(input("Guess the number (1-100): "))
if guess > number:
print("Too high!")
elif guess < number:
print("Too low!")
else:
print("You guessed it!")
best beginner python projects
best python projects for resume
cool python projects with source code
download python projects with source code
100 python projects
100 python projects for beginners
100 python projects for final year
7. Rock Paper Scissors Game
import random
choices = ['rock', 'paper', 'scissors']
user = input("Enter rock, paper, or scissors: ")
computer = random.choice(choices)
print(f"Computer chose: {computer}")
if user == computer:
print("It's a tie!")
elif (user == 'rock' and computer == 'scissors') or (user == 'paper' and computer == 'rock') or (user == 'scissors' and computer == 'paper'):
print("You win!")
else:
print("You lose!")
8. Tic-Tac-Toe Game
import random
def print_board(board):
for row in board:
print(" | ".join(row))
print("
")
def check_winner(board, player):
for row in board:
if all(s == player for s in row):
return True
for col in range(3):
if all(board[row][col] == player for row in range(3)):
return True
if all(board[i][i] == player for i in range(3)) or all(board[i][2-i] == player for i in range(3)):
return True
return False
def tic_tac_toe():
board = [[" " for _ in range(3)] for _ in range(3)]
players = ["X", "O"]
turn = 0
for _ in range(9):
print_board(board)
row, col = map(int, input(f"Player {players[turn]} enter row and col (0-2): ").split())
if board[row][col] == " ":
board[row][col] = players[turn]
if check_winner(board, players[turn]):
print_board(board)
print(f"Player {players[turn]} wins!")
return
turn = 1 - turn
else:
print("Invalid move! Try again.")
print("It's a tie!")
tic_tac_toe()
9. BMI Calculator
def bmi_calculator():
weight = float(input("Enter weight (kg): "))
height = float(input("Enter height (m): "))
bmi = weight / (height ** 2)
print(f"Your BMI is {bmi:.2f}")
if bmi < 18.5:
print("Underweight")
elif bmi < 24.9:
print("Normal weight")
elif bmi < 29.9:
print("Overweight")
else:
print("Obesity")
bmi_calculator()
10. Digital Clock
from tkinter import *
import time
def update_time():
current_time = time.strftime('%H:%M:%S')
label.config(text=current_time)
label.after(1000, update_time)
root = Tk()
root.title("Digital Clock")
label = Label(root, font=('Helvetica', 48), fg='red')
label.pack()
update_time()
root.mainloop()
11. Email Slicer
email = input("Enter your email: ")
username, domain = email.split("@")
print(f"Username: {username}, Domain: {domain}")
12. QR Code Generator
import qrcode
data = input("Enter text to generate QR code: ")
qr = qrcode.make(data)
qr.show()
13. Markdown to HTML Converter
import markdown
def convert_md_to_html():
md_text = """# Hello World
This is a markdown file!"""
html = markdown.markdown(md_text)
print(html)
convert_md_to_html()
14. Face Detection
import cv2
def detect_faces():
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml")
img = cv2.imread("face.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow("Face Detection", img)
cv2.waitKey(0)
detect_faces()
15. Text-to-Speech Converter
import pyttsx3
def text_to_speech():
text = input("Enter text to speak: ")
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
text_to_speech()
16. Currency Converter
from forex_python.converter import CurrencyRates
def convert_currency():
c = CurrencyRates()
from_currency = input("From currency (USD, EUR, etc.): ")
to_currency = input("To currency: ")
amount = float(input("Amount: "))
result = c.convert(from_currency, to_currency, amount)
print(f"Converted amount: {result:.2f} {to_currency}")
convert_currency()
17. Expense Tracker
expenses = []
def add_expense():
item = input("Enter expense item: ")
amount = float(input("Enter amount: "))
expenses.append((item, amount))
def show_expenses():
for item, amount in expenses:
print(f"{item}: ${amount:.2f}")
add_expense()
show_expenses()
18. Web Scraper
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
print(soup.title.text)
19. Portfolio Website with Flask
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Welcome to my portfolio!"
app.run(debug=True)
20. Chatbot with OpenAI API
import openai
openai.api_key = "your_api_key_here"
prompt = input("Ask me anything: ")
response = openai.Completion.create(engine="text-davinci-003", prompt=prompt, max_tokens=100)
print(response["choices"][0]["text"])
21. AI Image Classifier
from tensorflow.keras.models import load_model
from tensorflow.keras.preprocessing import image
import numpy as np
model = load_model("model.h5")
img = image.load_img("test.jpg", target_size=(224, 224))
img_array = image.img_to_array(img)/255.0
img_array = np.expand_dims(img_array, axis=0)
prediction = model.predict(img_array)
print("Predicted class:", np.argmax(prediction))
Conclusion & Next Steps
These 21 Python mini projects are perfect for learning and building practical coding experience.
Call to Action:
🚀 Start coding now! Download the PDF or visit our GitHub repository.
💬 Have a project idea? Share it in the comments below!
🔗 Further Reading:
Python projects with source code
21 python mini projects with code github
21 python mini projects with code pdf
21 python mini projects with code free download
Python mini projects with source code
21 python mini projects with code pdf download
Python projects with source code for final year
Python projects with source code PDF
0 Comments