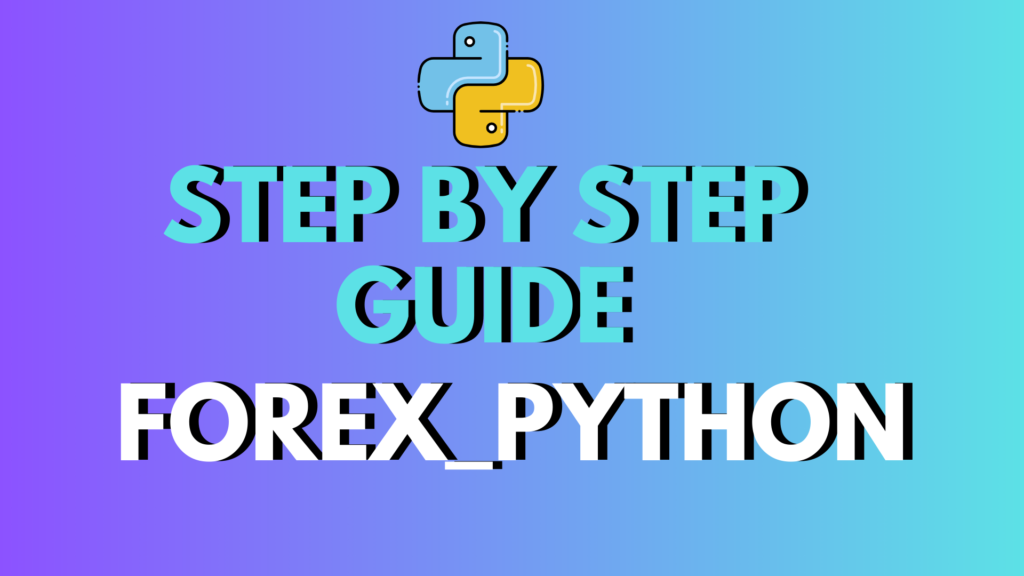
Introduction
If you’re a Python developer looking for an efficient way to access forex exchange rates, cryptocurrency prices, and currency conversion tools, forex_python
might be the perfect solution. This lightweight library provides a simple API for retrieving real-time exchange rates, making it ideal for financial applications, trading bots, and personal projects.
In this guide, we’ll explore how to use forex_python
, its key features, troubleshooting tips, alternatives, and provide practical examples to get you started.
What is forex_python
?
forex_python
is a free and open-source Python library designed to facilitate foreign exchange rate conversions. It allows developers to access live exchange rates, historical forex data, and cryptocurrency prices with minimal effort. The library is available on GitHub and can be installed easily via pip
.
Key Features of forex_python
- Live Exchange Rates: Fetch real-time currency exchange rates.
- Currency Conversion: Convert one currency to another effortlessly.
- Historical Data: Retrieve past exchange rates for analysis.
- Cryptocurrency Support: Get prices for major cryptocurrencies.
- Lightweight & Free: A simple, no-cost solution for forex applications.
- Multi-Currency Support: Works with all major global currencies.
- Simple API: Easy-to-use functions for quick integration into projects.
Forex_python converter
Forex_python github
forex-python documentation
Forex_python exchange rate
Forex_python free
Forex-python not working
Forex-python API
Forex-python example
How to Install and Use forex_python
Before you start using forex_python
, you need to install it in your Python environment:
pip install forex-python
Once installed, you can import it and start fetching exchange rates.
How to Get Currency Exchange Rates in Python
To retrieve the exchange rate between two currencies, use the following Python script:
from forex_python.converter import CurrencyRates
c = CurrencyRates()
usd_to_eur = c.get_rate('USD', 'EUR')
print(f"Exchange rate from USD to EUR: {usd_to_eur}")
How to Convert Currency Using forex_python
Currency conversion is straightforward with forex_python
. You can convert any amount from one currency to another:
amount = 100 # Amount in USD
euros = c.convert('USD', 'EUR', amount)
print(f"{amount} USD is equal to {euros:.2f} EUR")
Retrieving Historical Exchange Rates
You can also fetch historical exchange rates for specific dates:
from datetime import datetime
date = datetime(2023, 1, 1) # Fetch exchange rate from Jan 1, 2023
historical_rate = c.get_rate('USD', 'EUR', date)
print(f"Exchange rate on {date.strftime('%Y-%m-%d')} from USD to EUR: {historical_rate}")
Forex_python converter
Forex_python github
Forex_python free
forex-python documentation
Forex_python exchange rate
Forex-python not working
Forex-python API
pip install forex-python
forex_python
API and Documentation
The forex_python
API provides multiple endpoints to access forex data. You can find the complete documentation on the forex-python GitHub page.
Common Issues and Fixes
forex-python
Not Working?
If you encounter errors while using forex_python
, consider the following fixes:
- Ensure you have an active internet connection.
- Check if the API provider’s services are temporarily down. Some API sources might be unavailable at times.
- Upgrade to the latest version using:
pip install --upgrade forex-python
- Try alternative forex APIs like
exchangeratesapi
orcurrency-api
if the issue persists.
Building a Small Project Using forex_python
Let’s create a simple currency converter application in Python using forex_python
.
from forex_python.converter import CurrencyRates
def currency_converter():
c = CurrencyRates()
from_currency = input("Enter base currency (e.g., USD): ").upper()
to_currency = input("Enter target currency (e.g., EUR): ").upper()
amount = float(input("Enter amount to convert: "))
converted_amount = c.convert(from_currency, to_currency, amount)
print(f"{amount} {from_currency} is equal to {converted_amount:.2f} {to_currency}")
currency_converter()
Challenge: Enhance the Currency Converter
Try adding the following features to the script above:
- Fetch and display historical exchange rates.
- Add cryptocurrency conversion support.
- Build a graphical user interface (GUI) using Tkinter.
Building a small project using forex_python github
Building a small project using forex_python example
Forex-python
Currency converter in Python with source code
forex-python documentation
forex-python not working
Develop an application of currency converter using python
Google currency converter API Python
Best Apps for Currency Conversion
While forex_python
is excellent for coding-based currency conversion, here are some popular mobile and web-based apps for real-time forex conversions:
- XE Currency Converter (Mobile & Web)
- Google Currency Converter (Integrated in Google Search)
- OANDA Currency Converter (Professional trading platform)
- Currency Converter Plus (Mobile app with offline mode)
Alternative APIs for Forex Data
If forex_python
does not meet your needs, here are some alternative APIs:
- ExchangeRatesAPI.io – Provides free and premium exchange rate data.
- CurrencyLayer – Reliable forex data with historical and real-time rates.
- Open Exchange Rates – Offers JSON-based forex API with extensive coverage.
Conclusion
forex_python
is a powerful yet simple Python library for retrieving forex exchange rates and performing currency conversions. Whether you’re building a financial application, a trading bot, or just need quick forex data for personal use, forex_python
provides a convenient and free solution.
Call to Action
🚀 Ready to start using forex_python
? Install it now and explore the possibilities! If you found this guide useful, share it with fellow Python developers and forex enthusiasts. Happy coding! 💻💰
0 Comments