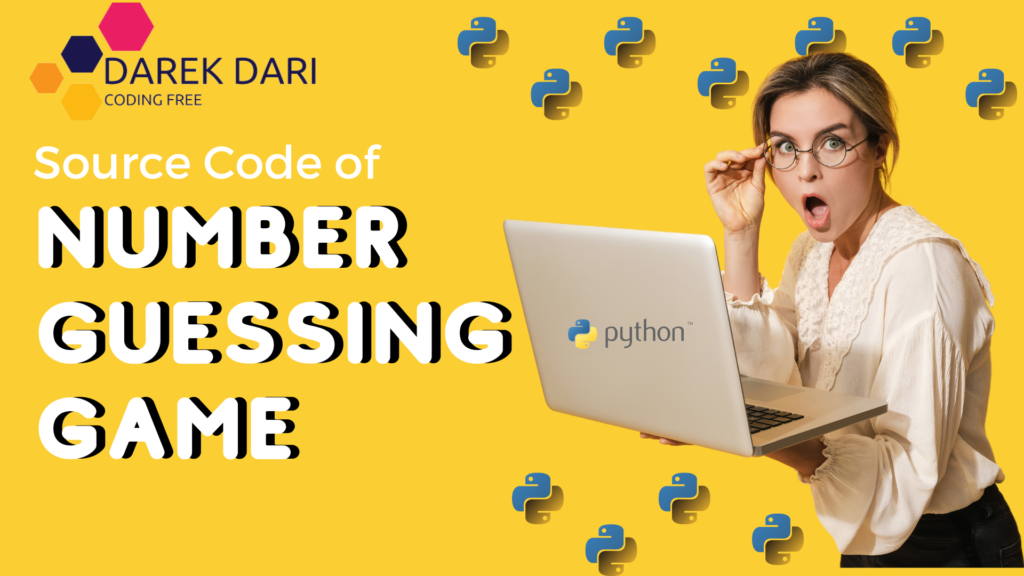
Table of Contents
Introduction To Number Guessing Game
Hello and welcome to our Python programming adventure! In today’s session, we’ll explore creating a traditional number guessing game with Python.
This project is not just enjoyable, but it’s also an excellent opportunity to reinforce essential programming principles such as loops, conditionals, and managing user input.
Ever wondered what your first programming project should be after learning a language like Python? Look no further! In this article, we’ll guide you through creating a fun and foundational project: building a Number Guessing Game.
number guessing game
number guessing
guess the number game
number guessing game python
python guess the number game
python number guessing game
guess the number game python
crisis core number guessing game
Understanding the Game
The game of guessing numbers is simple but fun. A number between 1 and 100 is chosen at random by the computer, and the player has to try and guess this number within a set number of tries.
After each guess, the game gives feedback on whether the guessed number is too high or too low, which helps the player make a better guess next time.
guessing number game python
guess number game python
number guessing tricks
guess number magic trick
guess the number magic trick
guess the number trick
guessing the number trick
Step-by-Step Implementation
Step 1: Setting Up the Game
Let’s start by importing the random
module, which allows us to generate random numbers:
import random
Step 2: Defining the Game Function
Next, we define a function guess_number()
to encapsulate the game logic:
def guess_number():
print("Welcome to the Number Guessing Game!")
print("I'm thinking of a number between 1 and 100.")
secret_number = random.randint(1, 100)
attempts = 0
while attempts < 10:
print("\nAttempt:", attempts + 1)
try:
guess = int(input("Enter your guess (between 1 and 100): "))
except ValueError:
print("Invalid input! Please enter a valid number.")
continue
if guess < 1 or guess > 100:
print("Please enter a number within the range of 1 to 100.")
continue
if guess < secret_number:
print("Too low! Try a higher number.")
elif guess > secret_number:
print("Too high! Try a lower number.")
else:
print(f"Congratulations! You've guessed the number ({secret_number}) correctly in {attempts + 1} attempts!")
return
attempts += 1
print(f"\nGame over! The number I was thinking of was {secret_number}. Better luck next time!")
Step 3: Running the Game
To start the game, simply call the guess_number()
function:
guess_number()
How It Works
Step | Explanation |
---|---|
Random Number Generation | The game uses random.randint(1, 100) to generate a random number between 1 and 100, which the player tries to guess. |
User Interaction | The player inputs their guess, and the game checks whether the guess is correct, too high, or too low. |
Game Loop | The game continues in a loop until the player guesses correctly or exhausts all attempts (up to 10). |
Input Validation | A try-except block ensures the player enters a valid integer within the specified range (1 to 100). |
Outcome | If the player guesses correctly within the allowed attempts, they win; otherwise, the game reveals the correct number. |
guessing game in python
guessing game python
python guessing game
magic number guessing trick
crisis core number guessing game
number guessing game java
guess number game java
Tips for Enhancement
Enhance your number guessing game with these tips:
Tip | Description |
---|---|
User Experience | Improve feedback messages to provide more descriptive hints or visual cues for better player engagement. |
Difficulty Levels | Adjust the range of numbers or the number of attempts to create different difficulty levels for players. |
Scorekeeping | Implement a scoring system to track and display the player’s performance across multiple games. |
Other Solution for Number Guessing Game In Python
You’ll define a range—from A to B (where A and B are integers).
Python will pick a secret number within your chosen range. Your mission? Guess that number in the fewest attempts possible!
Scenario 1: Imagine you set the range from 1 to 100, and Python randomly picks 42:
- Your first guess: 50. Python replies, “Oops, too high!”
- Next, you guess 25. Python says, “Nope, too low!”
- You refine with 37. Still too low!
- Trying 43. “Too high!”
- Nailing it on your sixth attempt with 41. “Congratulations! You got it in 6 tries!”
Scenario 2: Now, narrow it down to 1 to 50, and Python picks 42:
- Starting with 25. “Too low!”
- Advancing with 37. “Still too low!”
- Trying 43. “Too high!”
- Nailing it on your fifth attempt with 41. “Congratulations! You got it in just 5 tries!”
To estimate the minimum attempts needed, use this formula:
Minimum number of guesses=⌈log2(Upper bound−Lower bound+1)⌉\text{Minimum number of guesses} = \lceil \log_2(\text{Upper bound} - \text{Lower bound} + 1) \rceilMinimum number of guesses=⌈log2(Upper bound−Lower bound+1)⌉
Here’s how we’ll make the magic happen:
- Input your preferred lower and upper bounds.
- Python will generate a random number within your specified range.
- Keep guessing until you find the number:
- If your guess is too high, Python kindly nudges you, “Try again! Too high.”
- If your guess is too low, Python encourages, “Try again! Too low.”
- Guess correctly within the minimum attempts, and Python celebrates with, “Congratulations!”
- If not, Python sympathizes with, “Better Luck Next Time!”
java number guessing game
guess the number game java
guess game in java
guessing game java
guess game java
guessing game in java
java guessing game
number guessing game in java
You can also select your preferred language for the game prompts!
import random
import math
def number_guessing_game(language='english'):
print(f"Welcome to the Number Guessing Game! (Language: {language.capitalize()})")
print("Let's see how well you can read Python's mind...\n")
while True:
try:
lower = int(input(f"Enter Lower bound (whole number): "))
upper = int(input(f"Enter Upper bound (whole number): "))
if lower >= upper:
print("Upper bound must be greater than Lower bound. Let's try that again.")
continue
break
except ValueError:
print("Whoops! Please enter whole numbers for the bounds.")
secret_number = random.randint(lower, upper)
min_attempts = math.ceil(math.log2(upper - lower + 1))
attempts = 0
print(f"\nI've picked a number between {lower} and {upper}. You have {min_attempts} tries. Let's go!\n")
while attempts < min_attempts:
attempts += 1
try:
if language == 'english':
guess = int(input(f"Attempt {attempts}: What's your guess? "))
elif language == 'french':
guess = int(input(f"Essai {attempts}: Quel est votre guess? "))
elif language == 'spanish':
guess = int(input(f"Intento {attempts}: ¿Cuál es tu conjetura? "))
else:
print("Language not supported. Using English prompts.")
guess = int(input(f"Attempt {attempts}: What's your guess? "))
if guess < lower or guess > upper:
if language == 'english':
print(f"Hey, I said between {lower} and {upper}. Try again!")
elif language == 'french':
print(f"Eh bien, je dis entre {lower} et {upper}. Réessayez!")
elif language == 'spanish':
print(f"Oye, dije entre {lower} y {upper}. ¡Inténtalo de nuevo!")
else:
print("Invalid input! Please enter a valid whole number.")
continue
if guess < secret_number:
if language == 'english':
print("Hmm... Too low!")
elif language == 'french':
print("Hmm... Trop bas!")
elif language == 'spanish':
print("Hmm... Demasiado bajo!")
elif guess > secret_number:
if language == 'english':
print("Oops... Too high!")
elif language == 'french':
print("Oops... Trop haut!")
elif language == 'spanish':
print("Ups... ¡Demasiado alto!")
else:
if language == 'english':
print(f"Congratulations! You guessed {secret_number} in {attempts} tries!")
elif language == 'french':
print(f"Félicitations! Vous avez deviné {secret_number} en {attempts} tentatives!")
elif language == 'spanish':
print(f"¡Felicidades! Adivinaste {secret_number} en {attempts} intentos!")
break
except ValueError:
if language == 'english':
print("Oops! That's not a number. Please enter a valid whole number.")
elif language == 'french':
print("Oops! Ce n'est pas un nombre. Veuillez entrer un nombre entier valide.")
elif language == 'spanish':
print("¡Ups! Eso no es un número. Por favor, introduce un número entero válido.")
else:
print("Invalid input! Please enter a valid whole number.")
else:
if language == 'english':
print(f"\nSorry, the number was {secret_number}. Maybe next time!")
elif language == 'french':
print(f"\nDésolé, le nombre était {secret_number}. Peut-être la prochaine fois!")
elif language == 'spanish':
print(f"\nLo siento, el número era {secret_number}. ¡Quizás la próxima vez!")
play_again = input("\nWanna play again? (yes/no): ").lower()
if play_again == "yes" or play_again == "y":
language_choice = input("Choose your language (english/french/spanish): ").lower()
number_guessing_game(language_choice)
else:
if language == 'english':
print("\nThanks for playing with Python! See you next time!")
elif language == 'french':
print("\nMerci d'avoir joué avec Python! À la prochaine!")
elif language == 'spanish':
print("\n¡Gracias por jugar con Python! ¡Hasta la próxima!")
language_choice = input("Choose your language (english/french/spanish): ").lower()
number_guessing_game(language_choice)
number guessing game crisis core
number guessing game javascript
javascript number guessing game
number guessing game js
guess the number game javascript
javascript guess the number game
crisis core aerith affection
Output
For English
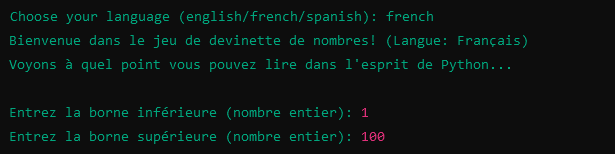
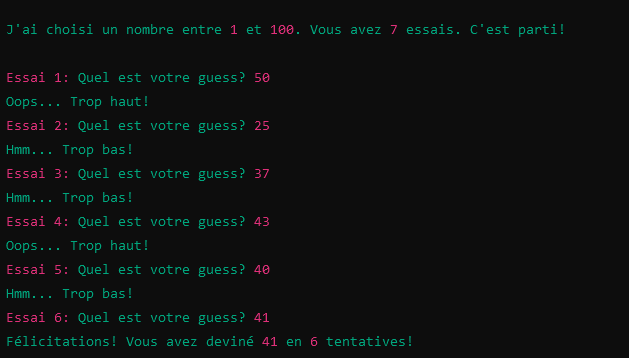
For Spanish
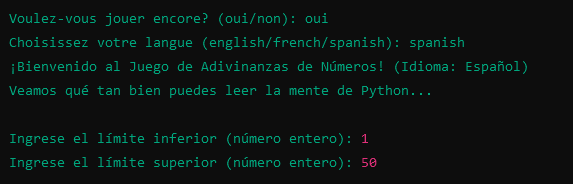
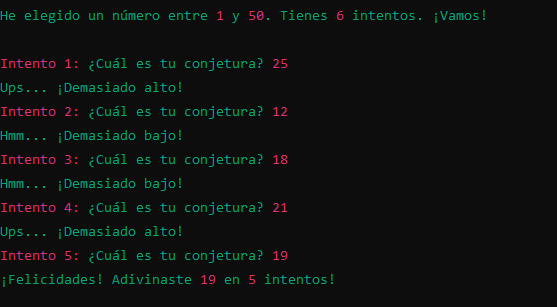
The game runs on a time complexity of O(log(n)), where n represents the difference between the upper and lower bounds of your selected range. This code has a space complexity of O(1), utilizing a fixed amount of memory regardless of the input size.
Are you ready to start your Python programming journey? Mastering AI and ChatGPT opens the door to the future of technology.
random number guessing game python
guessing game python code
crisis core perfume
number guessing game algorithm
guess the number python code
random number guessing game
guess a number between 1 and 100 python
guessing game javascript
guessing game js
guess game javascript
Ideal for tech enthusiasts, this course will guide you through using AI and ChatGPT with practical, hands-on lessons. Enhance your skills and develop innovative applications that make an impact.
What is a number guessing game?
Version 1: Picture yourself in a game where you become a detective with numbers! In this number guessing game, you’ll be provided with a range of numbers, let’s say from 1 to 100. The computer selects a number secretly within this range, and your task, if you decide to accept it, is to determine what that number is!
You begin by making a guess. If your guess is too high, the computer will suggest you to aim lower. If it’s too low, it will encourage you to aim higher. With each guess, you edge closer and closer to solving the mystery!
guess random number
python guessing game 1 100
python guessing game 1-100
number guessing game trick
guess the number game trick
pick any number trick
javascript guessing game 1 100
The game keeps a record of how many guesses it takes you to hit the mark. The real excitement kicks in when you attempt to surpass your own record for the fewest guesses!
It’s not just about guessing numbers—it’s a glimpse into the world of programming. You’ll discover interesting concepts like how computers think, using if-else statements to guide your guesses, and even how randomness influences games and simulations.
So, the next time you’re seeking a fun way to exercise your brain and gain some insights into coding, immerse yourself in a number guessing game. Who knows, you might uncover a talent for cracking codes!
How do I play Guess My number?
javascript guessing game 1-100
number guessing game python code
code guessing game
crisis core godlike speed
crisis core materia mini game
godlike speed crisis core
number guessing game code
magic trick guess number someone thinking
gg
math trick to guess number
guessing game python example
how to code a guessing game in python
python code guess the number
crisis core mini games
aerith affection crisis core
crisis core shell mini game
Conclusion
Developing a Number Guessing Game in Python is more than just coding—it’s an adventure of exploration and skill development.
Whether you’re new to programming or aiming to enhance your knowledge, this endeavor provides both difficulty and fun. Therefore, jump in, explore, and relish the experience of learning through play!
javascript guessing game
random number game python
number guesser python
number guessing python
random number guessing game python using functions
how to make a guessing game in python
guess the number java
crisis core guessing game
Have fun enhancing your Python skills by creating the number guessing game! This project is a great way to learn programming concepts while enjoying the interactive nature of coding.
Whether you’re new to programming or want to strengthen your abilities, this game provides a fun and practical way to dive into Python.
So, get creative, make it your own, and keep coding!
1 Comment
100 Best Python Projects With Source Code: Beginner To Pro · August 23, 2024 at 11:04 pm
[…] 🔹 Number Guessing Game […]