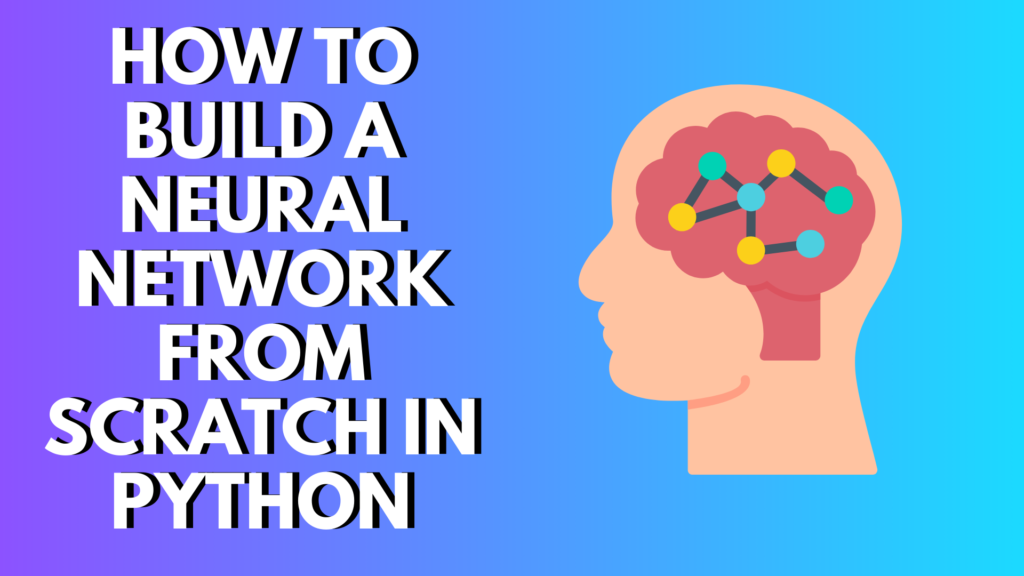
Introduction
Neural networks are the backbone of modern artificial intelligence, powering everything from voice recognition to self-driving cars.
While deep learning frameworks like TensorFlow and PyTorch make building neural networks easier, understanding how they work at a fundamental level is crucial for any AI enthusiast. In this guide, we will walk you through the process of building a neural network from scratch in Python, ensuring you grasp the core concepts without relying on high-level libraries.
Why Build a Neural Network from Scratch?
- Deep Understanding: Helps you comprehend how forward and backward propagation work.
- Customization: Enables you to tweak every component as per your needs.
- Skill Enhancement: Improves your proficiency in Python and machine learning.
Prerequisites
Before diving in, make sure you have a basic understanding of:
- Python programming
- Linear algebra (matrices, vectors, dot product)
- Calculus (derivatives, chain rule)
- Fundamentals of machine learning
You’ll also need Python installed with NumPy and Matplotlib:
pip install numpy matplotlib
Step 1: Importing Necessary Libraries
We’ll use NumPy for matrix operations and Matplotlib for visualization.
import numpy as np
import matplotlib.pyplot as plt
Step 2: Initializing the Neural Network
We’ll create a simple neural network with one hidden layer.
def initialize_parameters(input_size, hidden_size, output_size):
np.random.seed(42)
W1 = np.random.randn(hidden_size, input_size) * 0.01
b1 = np.zeros((hidden_size, 1))
W2 = np.random.randn(output_size, hidden_size) * 0.01
b2 = np.zeros((output_size, 1))
parameters = {"W1": W1, "b1": b1, "W2": W2, "b2": b2}
return parameters
Step 3: Implementing the Activation Function
We use the sigmoid function for activation.
def sigmoid(Z):
return 1 / (1 + np.exp(-Z))
Step 4: Forward Propagation
Calculate the output of each layer:
def forward_propagation(X, parameters):
W1, b1, W2, b2 = parameters["W1"], parameters["b1"], parameters["W2"], parameters["b2"]
Z1 = np.dot(W1, X) + b1
A1 = sigmoid(Z1)
Z2 = np.dot(W2, A1) + b2
A2 = sigmoid(Z2)
cache = {"Z1": Z1, "A1": A1, "Z2": Z2, "A2": A2}
return A2, cache
Step 5: Computing the Loss Function
We’ll use the binary cross-entropy loss function.
def compute_loss(Y, A2):
m = Y.shape[1]
loss = -np.sum(Y * np.log(A2) + (1 - Y) * np.log(1 - A2)) / m
return loss
Step 6: Backpropagation
Calculate gradients to update weights:
def backward_propagation(X, Y, parameters, cache):
m = X.shape[1]
W2 = parameters["W2"]
dZ2 = cache["A2"] - Y
dW2 = np.dot(dZ2, cache["A1"].T) / m
db2 = np.sum(dZ2, axis=1, keepdims=True) / m
dZ1 = np.dot(W2.T, dZ2) * (cache["A1"] * (1 - cache["A1"]))
dW1 = np.dot(dZ1, X.T) / m
db1 = np.sum(dZ1, axis=1, keepdims=True) / m
grads = {"dW1": dW1, "db1": db1, "dW2": dW2, "db2": db2}
return grads
Step 7: Updating Parameters
Using gradient descent to update weights:
def update_parameters(parameters, grads, learning_rate):
parameters["W1"] -= learning_rate * grads["dW1"]
parameters["b1"] -= learning_rate * grads["db1"]
parameters["W2"] -= learning_rate * grads["dW2"]
parameters["b2"] -= learning_rate * grads["db2"]
return parameters
Step 8: Training the Neural Network
We bring everything together in a loop.
def train(X, Y, input_size, hidden_size, output_size, epochs, learning_rate):
parameters = initialize_parameters(input_size, hidden_size, output_size)
for i in range(epochs):
A2, cache = forward_propagation(X, parameters)
loss = compute_loss(Y, A2)
grads = backward_propagation(X, Y, parameters, cache)
parameters = update_parameters(parameters, grads, learning_rate)
if i % 1000 == 0:
print(f"Epoch {i}, Loss: {loss}")
return parameters
Step 9: Making Predictions
def predict(X, parameters):
A2, _ = forward_propagation(X, parameters)
return A2 > 0.5
Conclusion
Congratulations! You’ve built a neural network from scratch in Python. This foundational understanding is crucial before diving into deep learning frameworks. If you found this guide helpful, share it with others and explore more advanced topics like multi-layer networks, dropout regularization, and optimizers like Adam.
Frequently Asked Questions (FAQ)
Is Python good for neural networks? Yes, Python is widely used in AI and deep learning due to its simplicity and powerful libraries like TensorFlow and PyTorch.
Is ChatGPT a neural network? Yes, ChatGPT is based on a large neural network architecture called a transformer, specifically GPT (Generative Pre-trained Transformer).
How to make AI in Python from scratch? You can create AI using Python by implementing machine learning models, neural networks, and deep learning techniques from scratch using NumPy, SciPy, and other libraries.
What is better, TensorFlow or PyTorch? Both are excellent deep learning frameworks. TensorFlow is more production-friendly, while PyTorch is preferred for research and experimentation.
Which Python is best for AI? The latest stable version of Python (3.x) is recommended for AI development.
How to build a neural network in Python? Follow the steps in this guide to implement a simple neural network from scratch using NumPy.
Which coding language is best for neural networks? Python is the most popular, but other languages like R, Julia, and C++ are also used.
How are neural networks built? Neural networks are built using layers of interconnected neurons with weighted connections trained using backpropagation.
How to make and train an AI? AI is trained using large datasets and optimized using machine learning algorithms like gradient descent.
How do you build a neural pathway? A neural pathway in AI refers to layers of neurons connected by weights that adjust during training.
How to make a neuron in Python? A simple neuron can be created using a weighted sum and activation function in Python.
Call to Action
Did you try implementing this neural network? Share your experience or questions in the comments below!
0 Comments