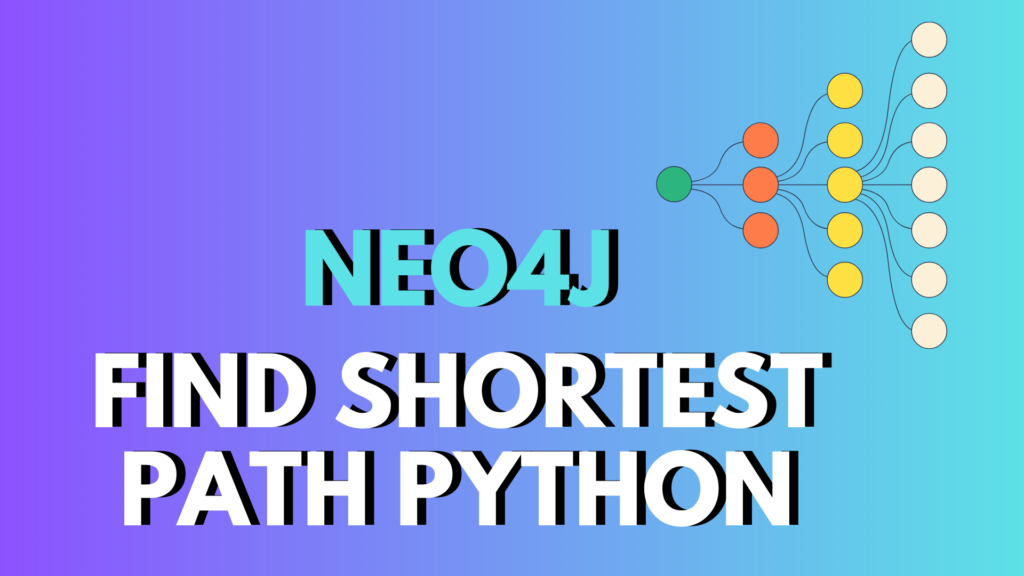
In the realm of graph databases, efficiently navigating relationships between entities is paramount. Neo4j, a leading graph database platform, offers robust tools to determine the shortest path between two nodes, facilitating tasks like route optimization, network analysis, and recommendation systems.
This guide delves into Neo4j find shortest path Python methods, Cypher shortest path queries, Neo4j Dijkstra, and advanced algorithms from the Neo4j GDS (Graph Data Science) library.
Understanding Shortest Paths in Neo4j
A shortest path algorithm determines the minimum sequence of edges between two nodes. Neo4j’s Cypher query language provides built-in functions for pathfinding, allowing users to extract valuable insights from their graph data.
Graph Representation of Cities and Roads
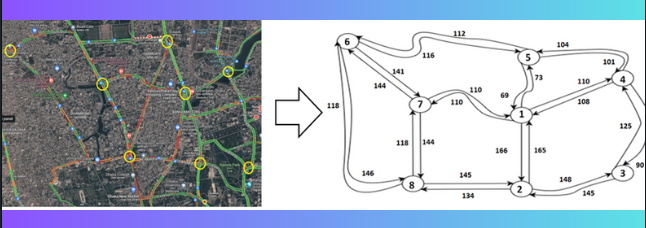
Neo4j’s Built-in Shortest Path Functions
Neo4j offers two primary Cypher shortest path functions:
shortestPath()
: Finds a single shortest path between two nodes.allShortestPaths()
: Retrieves all shortest paths of the same length between two nodes.
Example: Finding the Shortest Path
Imagine a dataset where cities are connected by roads. To find the shortest path between two nodes (‘CityA’ and ‘CityB’):
MATCH (start:City {name: 'CityA'}), (end:City {name: 'CityB'}),
path = shortestPath((start)-[:ROAD*]-(end))
RETURN path
This query identifies the shortest unweighted path between the two cities.
⚠️ Note: These functions are optimal for unweighted graphs. For weighted graphs (e.g., where distances or costs vary), Neo4j Dijkstra or A* algorithms are more suitable.
Weighted Shortest Path: Using Neo4j Dijkstra Algorithm
When relationships have weights (e.g., distance, time, cost), Dijkstra’s algorithm is ideal. Neo4j GDS provides an efficient implementation for finding weighted shortest paths.
Step-by-Step Execution of Dijkstra’s Algorithm
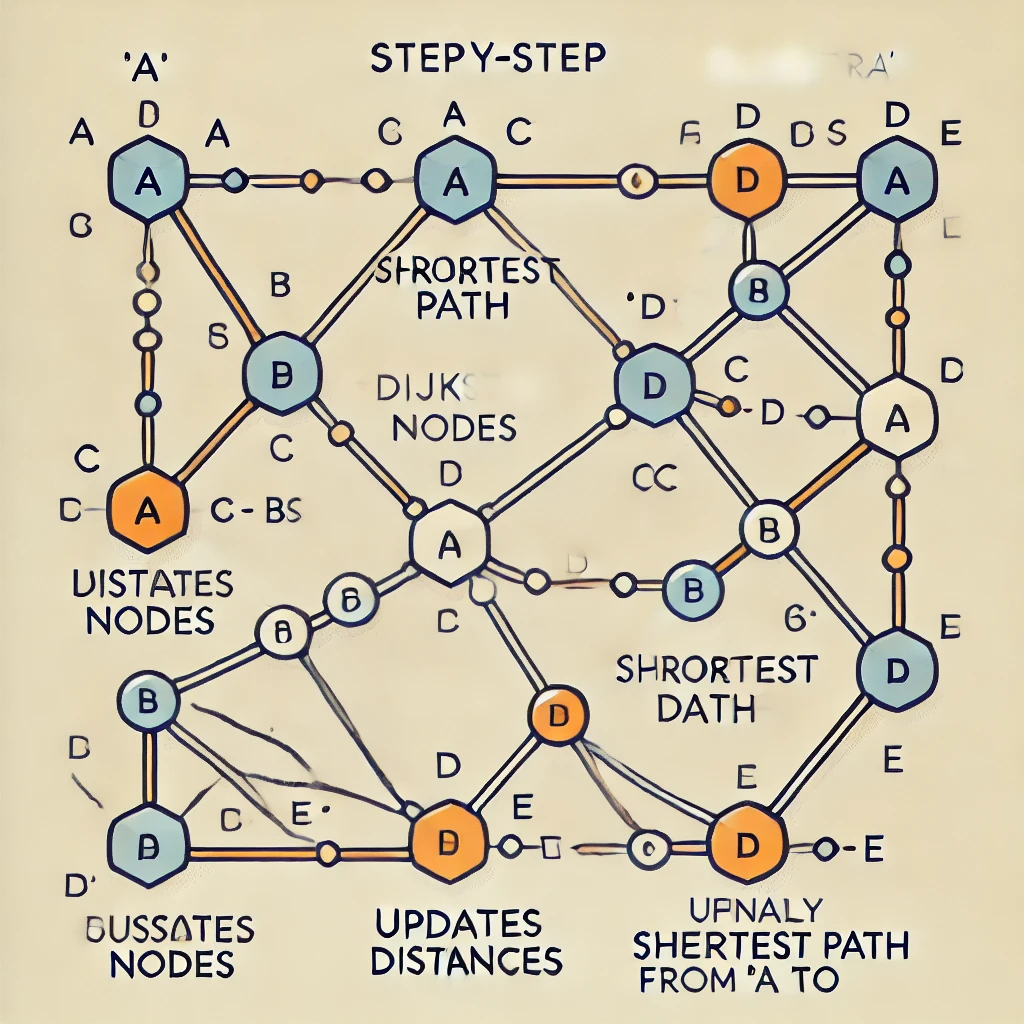
Example: Finding the Shortest Path with Weights
MATCH (source:City {name: 'CityA'}), (target:City {name: 'CityB'})
CALL gds.shortestPath.dijkstra.stream('myGraph', {
sourceNode: source,
targetNode: target,
relationshipWeightProperty: 'distance'
})
YIELD index, sourceNode, targetNode, totalCost, nodeIds, costs, path
RETURN
index,
gds.util.asNode(sourceNode).name AS sourceNodeName,
gds.util.asNode(targetNode).name AS targetNodeName,
totalCost,
[nodeId IN nodeIds | gds.util.asNode(nodeId).name] AS nodeNames,
costs,
nodes(path) as path
This query finds the shortest weighted path based on the ‘distance’ property in the Neo4j GDS graph.
Alternative Approach: Using Neo4j Reduce for Path Computation
The Neo4j reduce function can be used to compute cumulative properties of paths, such as total distance.
Example: Summing Path Weights with Reduce
MATCH (start:City {name: 'CityA'}), (end:City {name: 'CityB'}),
path = shortestPath((start)-[:ROAD*]-(end))
RETURN reduce(total = 0, r IN relationships(path) | total + r.distance) AS totalDistance
This query calculates the total distance along the shortest path.
Neo4j Find Shortest Path Python: Using Python with Neo4j
Neo4j integrates seamlessly with Python via the neo4j Python driver.
Example: Finding the Shortest Path in Python
from neo4j import GraphDatabase
uri = "bolt://localhost:7687"
user = "neo4j"
password = "password"
driver = GraphDatabase.driver(uri, auth=(user, password))
def find_shortest_path(tx, start, end):
query = """
MATCH (a:City {name: $start}), (b:City {name: $end}),
path = shortestPath((a)-[:ROAD*]-(b))
RETURN path
"""
result = tx.run(query, start=start, end=end)
return result.single()
with driver.session() as session:
path = session.read_transaction(find_shortest_path, "CityA", "CityB")
print(path)
driver.close()
This Python script queries Neo4j for the shortest path between two nodes and returns the result.
Best Practices for Efficient Shortest Path Queries
To optimize shortest path computations:
✅ Use Indexing – Ensure nodes have indexes for fast lookups.
✅ Graph Projection – Use in-memory graphs for better performance.
✅ Filter Relationships – Reduce the search space by specifying relationship types.
✅ Consider Parallel Processing – For large datasets, use GDS parallel computation.
🚀 Challenge: Test Your Neo4j Pathfinding Skills
Scenario: You have a graph of cities connected by flights, each with a ‘distance’ property.
Task: Write a Cypher query to find the shortest flight path between ‘CityX’ and ‘CityY’, considering the ‘distance’ property.
Share your solutions in the comments below!
Conclusion
Mastering Neo4j shortest path between two nodes enables powerful analysis of graph-based data. Whether using Cypher shortest path queries, Neo4j find shortest path Python, or advanced Neo4j GDS algorithms, you can efficiently navigate complex networks.
🔗 Learn More
If you found this guide helpful, share it with your network and leave a comment with your thoughts! 🚀
0 Comments