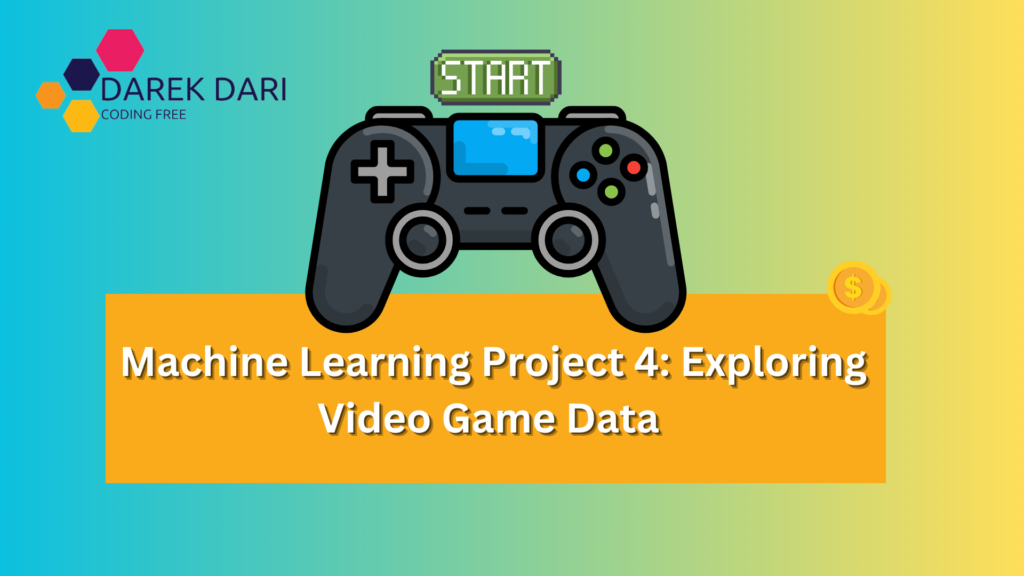
Table of Contents
Introduction
Data science often begins with exploring and understanding data before diving into complex models. In this blog, we’ll take you through an end-to-end journey of analyzing a video game dataset, from initial exploration to building a machine learning model for predicting game genres.
Also, check Machine Learning projects:
- Machine Learning Project 1: Honda Motor Stocks best Prices analysis
- Machine Learning Project 2: Diversity in Tech Companies Best EDA Analysis
- Machine Learning Project 3: Exploring Indian Cuisine Best Analysis
- Machine Learning Project 4: Exploring Video Game Data
- Machine Learning Project 5: Best Students Performance EDA
- Machine Learning Project 6: Obesity type Best EDA and classification
Step 1. Loading and Understanding the Dataset
Dataset Link: https://www.kaggle.com/datasets/dem0nking/video-game-ratings-dataset
machine learning projects github
machine learning projects for final year
machine learning projects for students
First, we import the necessary libraries and load the dataset:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler, OneHotEncoder
from sklearn.linear_model import LinearRegression
from sklearn.ensemble import RandomForestRegressor, RandomForestClassifier
from sklearn.metrics import mean_absolute_error, mean_squared_error, accuracy_score, classification_report, confusion_matrix
from sklearn.model_selection import GridSearchCV
import warnings
warnings.filterwarnings('ignore')
# Load the dataset
file_path = '/content/Video_Game_Information.csv'
df = pd.read_csv(file_path)
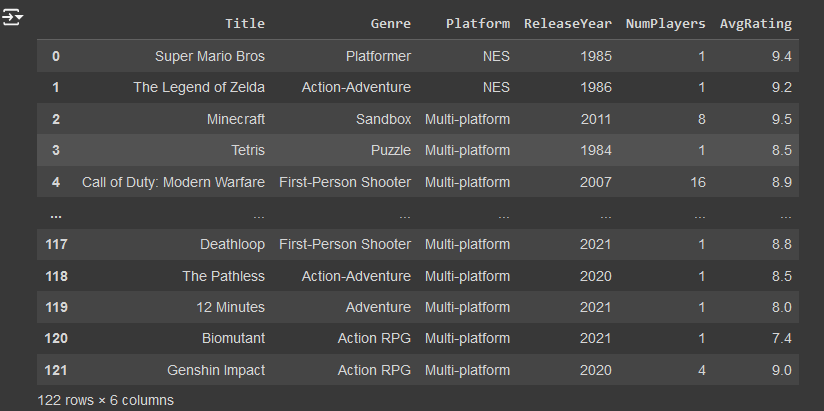
machine learning projects
machine learning projects with source code
machine learning projects github
machine learning projects for final year
machine learning projects for students
Let’s inspect the data to understand its structure:
print(df.info())
print(df.describe())
ml process
kaggle machine learning projects
machine learning project manager
machine learning project management
machine learning projects for masters students
Dataset Information
This step reveals the columns, data types, and the presence of any missing values. Summary statistics provide insights into the distribution and central tendencies of the numeric features.
Link: https://www.kaggle.com/datasets/dem0nking/video-game-ratings-dataset
Step 2. Visualizing the Data
Distribution of Numeric Features
Visualizing the distribution of features helps in understanding their spread and identifying any anomalies.
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
sns.histplot(df['ReleaseYear'], bins=20, kde=True)
plt.title('Distribution of Release Year')
plt.subplot(1, 2, 2)
sns.histplot(df['AvgRating'], bins=20, kde=True)
plt.title('Distribution of Average Rating')
plt.tight_layout()
plt.show()
plt.figure(figsize=(6, 5))
sns.histplot(df['NumPlayers'], bins=20, kde=True)
plt.title('Distribution of Number of Players')
plt.show()
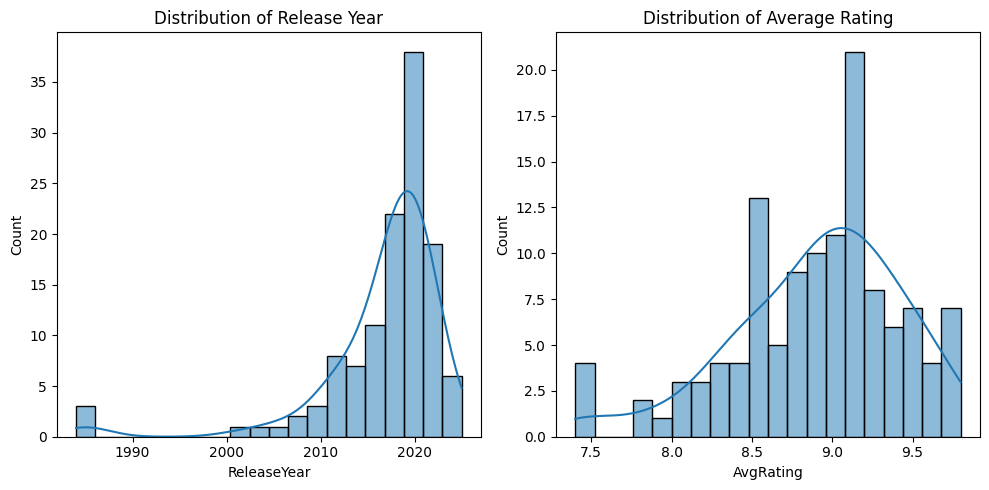
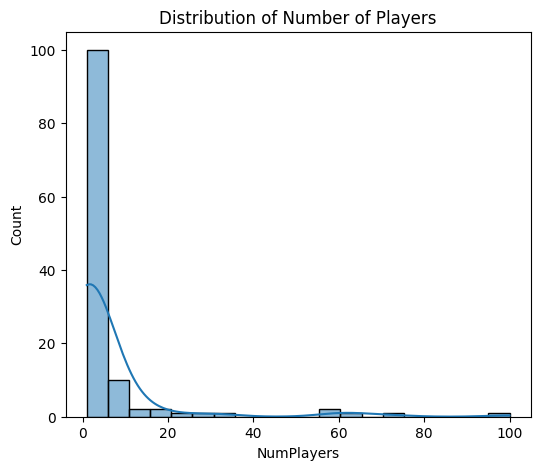
Distribution of Categorical Features
Categorical features such as ‘Genre’ and ‘Platform’ can be visualized using count plots.
plt.figure(figsize=(15, 5))
plt.subplot(1, 2, 1)
sns.countplot(y=df['Genre'], order=df['Genre'].value_counts().index)
plt.title('Distribution of Genres')
plt.subplot(1, 2, 2)
sns.countplot(y=df['Platform'], order=df['Platform'].value_counts().index)
plt.title('Distribution of Platforms')
plt.tight_layout()
plt.show()
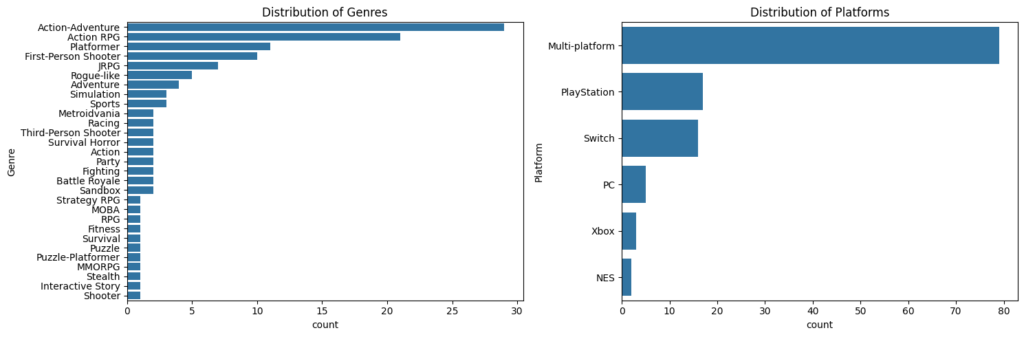
ml process
kaggle machine learning projects
machine learning project manager
machine learning project management
machine learning projects for masters students
Step 3. Exploring Relationships
Relationships Between Features
Understanding how features relate to each other can provide valuable insights, especially for predicting target variables.
plt.figure(figsize=(10, 5))
sns.boxplot(x='Genre', y='AvgRating', data=df)
plt.title('Average Rating by Genre')
plt.xticks(rotation=90)
plt.show()
plt.figure(figsize=(10, 5))
sns.boxplot(x='Platform', y='AvgRating', data=df)
plt.title('Average Rating by Platform')
plt.xticks(rotation=90)
plt.show()
plt.figure(figsize=(10, 5))
sns.scatterplot(x='ReleaseYear', y='AvgRating', hue='Genre', data=df)
plt.title('Average Rating over Years by Genre')
plt.show()
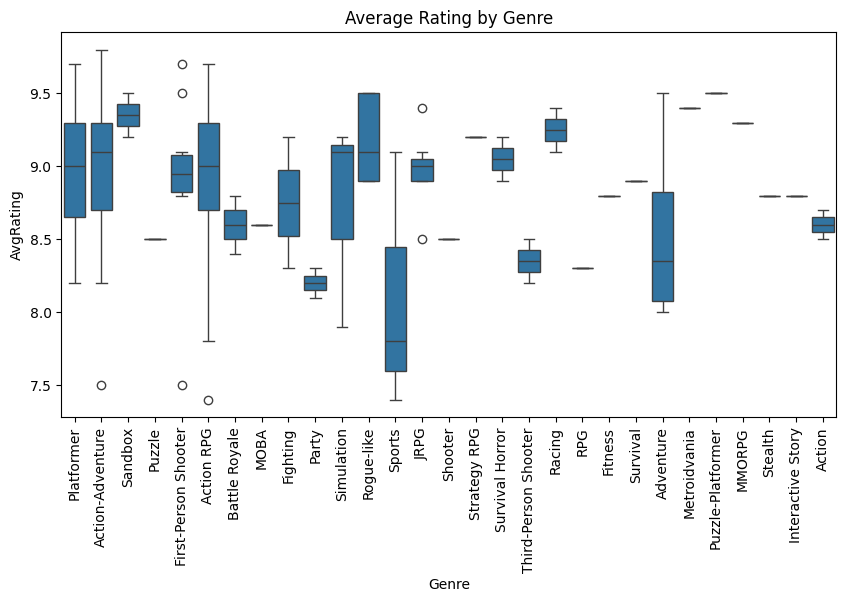
Step 4. Key Insights from the Data
Let’s extract some key insights:
- Oldest Games:
print(df.sort_values('ReleaseYear').head())
- Total Number of Games:
print(f"There are {df.shape[0]} games in the dataset.")
- Most Popular Platforms:
print(df['Platform'].value_counts().head())
- Top Rated Games:
print(df.groupby('Title')['AvgRating'].sum().sort_values(ascending=False).head())
- Most Common Genres:
python print(df['Genre'].value_counts().head())
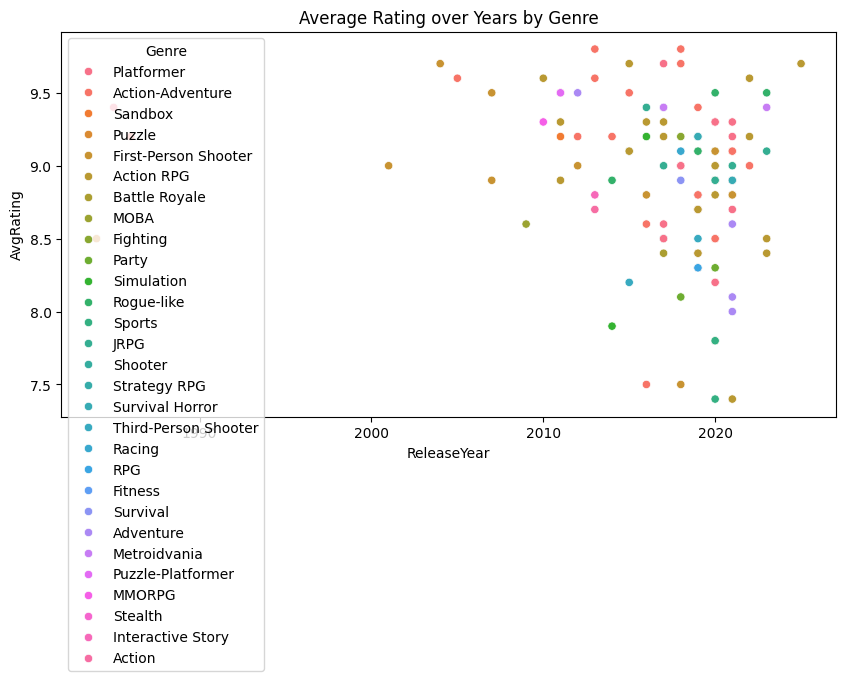
Step 5. Machine Learning for Genre Prediction
Data Preparation
Before building a model, we need to prepare our data by encoding categorical variables and splitting it into training and testing sets.
# Preprocessing
X = df.drop('Genre', axis=1)
y = df['Genre']
# Combine training and future games data
combined_df = pd.concat([df, future_games_df], ignore_index=True)
# Encoding categorical features
label_encoders = {}
for column in combined_df.select_dtypes(include=['object']).columns:
label_encoders[column] = LabelEncoder()
combined_df[column] = label_encoders[column].fit_transform(combined_df[column])
# Separate combined data back into training and future games
X = combined_df.drop('Genre', axis=1)
y = combined_df['Genre']
X_train, future_games = X.iloc[:len(df)], X.iloc[len(df):]
y_train = y.iloc[:len(df)]
# Splitting data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X_train, y_train, test_size=0.2, random_state=42)
ml process
kaggle machine learning projects
machine learning project manager
machine learning project management
machine learning projects for masters students
Model Training and Evaluation
We’ll use a Random Forest Classifier and perform hyperparameter tuning using GridSearchCV.
# Feature scaling
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Model training
model = SVC(kernel='linear', C=1.0, random_state=42)
model.fit(X_train_scaled, y_train)
# Model evaluation
y_pred = model.predict(X_test_scaled)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
print("Classification Report:")
print(classification_report(y_test, y_pred))
print("Confusion Matrix:")
print(confusion_matrix(y_test, y_pred))
Predicting Future Game Genres
Assuming we have data on future games, we can use our model to predict their genres.
# Example prediction for future games
future_games_encoded = future_games.copy()
for column in future_games_encoded.select_dtypes(include=['object']).columns:
future_games_encoded[column] = label_encoders[column].transform(future_games_encoded[column])
future_games_scaled = scaler.transform(future_games_encoded)
predicted_genres = model.predict(future_games_scaled)
# Print the predicted genres
for game, genre in zip(future_games_df['Title'], predicted_genres):
print(f"{game}: {genre}")
Other Machine Learning Model
import pandas as pd
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score, classification_report, confusion_matrix
from sklearn.preprocessing import LabelEncoder, StandardScaler
# Assume df is your complete DataFrame
# Preprocessing
X = df.drop('Genre', axis=1)
y = df['Genre']
# Combine training and future games data
combined_df = pd.concat([df, future_games_df], ignore_index=True)
# Encoding categorical features
label_encoders = {}
for column in combined_df.select_dtypes(include=['object']).columns:
label_encoders[column] = LabelEncoder()
combined_df[column] = label_encoders[column].fit_transform(combined_df[column])
# Separate combined data back into training and future games
X = combined_df.drop('Genre', axis=1)
y = combined_df['Genre']
X_train, future_games = X.iloc[:len(df)], X.iloc[len(df):]
y_train = y.iloc[:len(df)]
# Splitting data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X_train, y_train, test_size=0.2, random_state=42)
# Feature scaling
scaler = StandardScaler()
X_train_scaled = scaler.fit_transform(X_train)
X_test_scaled = scaler.transform(X_test)
# Model training
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train_scaled, y_train)
# Model evaluation
y_pred = model.predict(X_test_scaled)
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy:.2f}")
print("Classification Report:")
print(classification_report(y_test, y_pred))
print("Confusion Matrix:")
print(confusion_matrix(y_test, y_pred))
# Example prediction for future games
future_games_encoded = future_games.copy()
for column in future_games_encoded.select_dtypes(include=['object']).columns:
future_games_encoded[column] = label_encoders[column].transform(future_games_encoded[column])
future_games_scaled = scaler.transform(future_games_encoded)
predicted_genres = model.predict(future_games_scaled)
# Print the predicted genres
for game, genre in zip(future_games_df['Title'], predicted_genres):
print(f"{game}: {genre}")
step machine learning
step of machine learning
ml projects
ml project
machine learning python projects
machine learning projects in python
Is 50GB a lot of data for gaming?
Hey there! Curious if 50GB is a lot of data for gaming? Let’s dive in and have a casual chat, just like hanging out and enjoying a slice of pizza.
Game Download Size
- Big Fancy Games: You know those awesome, high-budget games like “Call of Duty” or “Red Dead Redemption 2”? Well, those bad boys are often much larger than 50GB. They’re like a loaded pizza with all the toppings.
- Smaller or Indie Games: On the flip side, you have indie games or smaller titles. They’re like a classic cheese pizza, simple and delightful. Most of these games are way under 50GB, often just a few gigabytes.
Game Updates and Patches
- Frequent Updates: Some games are like that friend who always needs a little extra attention. They receive updates and patches frequently, adding a few gigabytes here and there. Games like “Fortnite” or “Apex Legends” are always getting new stuff.
Data for Online Gaming
- Playing Online: Just playing games online doesn’t consume too much data. It’s like snacking on some treats – you use about 40MB to 300MB per hour. Not too shabby, right?
- Streaming Games: Now, if you’re streaming games (similar to Netflix for games), that’s a whole different story. Streaming in high definition (1080p or 4K) can devour a few gigabytes per hour. It’s like an all-you-can-eat buffet, goes by quickly!
Storage Considerations
- Storage Space: Modern consoles and PCs are like having a spacious fridge. They usually come with 500GB to a few terabytes of space. So, a single 50GB game is like a nice-sized pizza in your fridge – big but not overwhelming.
- External Storage: Many gamers use extra hard drives to expand their storage. It’s like having a second fridge in the garage, perfect for all those extra goodies.
Internet Data Caps
ISP Data Caps: Some internet providers set limits on how much data you can use each month, similar to strict parents. Downloading large games or streaming movies can quickly eat up your data allowance, especially if you're a heavy internet user.
The Bigger Picture
For Indie and Older Games: 50GB is a significant chunk of data for these types of games.
For Modern AAA Games: Considered standard, like ordering a large pizza for a party.
For Storage: It's manageable, but you need to be mindful of your available space.
For Data Caps: Keep a close watch on your usage to avoid any unexpected surprises.
So, is 50GB a lot? It can be, especially if you’re playing multiple games or have data caps to worry about.
However, for those big, impressive AAA games, it’s becoming more of the norm. Just ensure you have sufficient storage and monitor your data limits. Enjoy your gaming! 🎮.
How is data used in video games?
Here is a data usage in gaming quick reference:
Activity | Data Usage Estimate |
---|---|
Initial Game Download | 50GB+ (for large AAA games) |
Game Updates/Patches | Few MBs to several GBs |
Online Multiplayer | 40MB to 300MB per hour |
Voice Chat | Adds a bit more to online play |
Game Streaming | Several GBs per hour (HD/4K) |
Cloud Saves | Usually small, MBs |
Downloading DLC | Varies, can be several GBs |
Downloading Mods | Varies, small to several GBs |
In-Game Browsing | Similar to regular web browsing |
Social Features | Minimal additional data |
VR/AR Gaming | High data usage for real-time rendering |
DRM Checks | Small, minimal data |
step machine learning
step of machine learning
ml projects
ml project
machine learning python projects
machine learning projects in python
How do video games collect data?
Hey there, curious gamer! Have you ever wondered how video games gather data? Let’s break it down like we’re just having a casual chat over a delicious burger and some crispy fries. 🍔🍟
Player Behavior Tracking
- Gameplay Analytics: Games keep an eye on how you play, such as which levels you spend the most time on, how you interact with different features, and where you might be facing challenges. This valuable information helps developers make the game more enjoyable and captivating. It’s like receiving feedback without having to fill out a survey!
- Heatmaps: Some games use heatmaps to visualize where players frequently go within a level. These maps highlight areas of high activity and assist developers in understanding which parts of the game are the most engaging or need improvement.
In-Game Events and Actions
- Achievements and Progress: Every time you unlock an achievement or reach a new level, that data is recorded. This helps keep track of your progress and can be used to create leaderboards or provide personalized content tailored to your gaming journey.
- Choices and Consequences: In games with multiple storylines, the choices you make are tracked to shape the narrative and determine the outcomes. This data helps in customizing the story according to your decisions and enhancing future game designs.
Social Interactions
- Chat and Voice Communication: If you’re chatting with friends or using voice chat, those interactions might be recorded (though usually not the actual content). This information can assist in moderating conversations and improving social features within the game.
- Friend Lists and Multiplayer Matches: Your interactions with friends and other players are tracked to enhance matchmaking, suggest new friends, or create exciting social events for you to enjoy together.
Technical Data
- Performance Metrics: Games collect data on how they perform on your device, including frame rates, loading times, and crashes. This data helps developers optimize performance and fix any bugs that may arise.
- Device Information: Details about your hardware (such as your graphics card and CPU) and software (like your operating system) are collected to ensure compatibility and optimize game settings for the best gaming experience possible.
github artificial intelligence-projects
machine learning project life cycle
machine learning project python
machine learning projects python
deep learning projects for masters students
User Feedback
Surveys and Feedback Forms: At times, games may directly ask for your thoughts and opinions through in-game surveys or feedback forms. This information is extremely valuable as it helps improve the overall game experience.
Monetization and Purchases
- In-Game Purchases: Developers gain insights into spending habits and can optimize in-game stores by analyzing data on the items or upgrades you purchase. This information also enables them to create targeted offers and promotions.
- Ad Interactions: In free-to-play games, your interactions with ads, such as how often you watch or click on them, are tracked to enhance ad placements and relevance.
Location Data
Geolocation: Some games, especially mobile ones, utilize your location data to customize the gaming experience by offering location-specific content or events. This data also aids in understanding regional popularity and usage patterns.
Player Demographics
Account Information: When you create a game account, you provide details like your age, gender, and email. This information helps personalize the game experience and provides insights into the player base.
Data Sharing and Third-Party Services
Third-Party Integrations: Games often integrate third-party services for analytics, social features, or cloud saves. These services collect data to provide their functionality and may share valuable insights with the game developers.
How Do Video Games Collect Data?
Hey, curious gamer! Ever wondered how video games collect data? Let’s break it down like we’re just chatting over a burger and some fries. 🍔🍟
Player Behavior Tracking
- Gameplay Analytics: Games keep an eye on how you play – like which levels you spend the most time on, how you interact with different features, and where you might be getting stuck. This helps developers tweak the game to make it more fun and engaging. Think of it as getting feedback without having to fill out a survey.
- Heatmaps: Some games use heatmaps to see where players go most often in a level. These maps show hot spots of activity and can help developers understand which areas are most engaging or problematic.
In-Game Events and Actions
- Achievements and Progress: Every time you unlock an achievement or reach a new level, that data gets recorded. This helps keep track of your progress and can be used to create leaderboards or personalized content.
- Choices and Consequences: In games with branching storylines, your choices are tracked to shape the narrative and outcomes. This data helps in tailoring the story to your decisions and improving future game designs.
github artificial intelligence-projects
machine learning project life cycle
machine learning project python
machine learning projects python
deep learning projects for masters students
Social Interactions
- Chat and Voice Communication: If you’re chatting with friends or using voice chat, those interactions might be recorded (but usually just the fact that they happened, not the actual content). This can help with moderation and improving social features.
- Friend Lists and Multiplayer Matches: Your interactions with friends and other players are tracked to enhance matchmaking, suggest friends, or create social events.
Technical Data
- Performance Metrics: Games collect data on how they run on your device – like frame rates, loading times, and crashes. This helps developers optimize performance and fix bugs.
- Device Information: Details about your hardware (e.g., graphics card, CPU) and software (e.g., operating system) are collected to ensure compatibility and optimize game settings.
User Feedback
- Surveys and Feedback Forms: Sometimes, games will directly ask for your opinion through in-game surveys or feedback forms. This data is invaluable for improving the game experience.
Monetization and Purchases
- In-Game Purchases: Data on what items or upgrades you buy helps developers understand spending habits and optimize in-game stores. It also helps in creating targeted offers and promotions.
- Ad Interactions: In free-to-play games, how you interact with ads (like how often you watch them or click on them) is tracked to improve ad placements and relevance.
Location Data
- Geolocation: Some games, especially mobile ones, use your location data to tailor the experience – like offering location-specific content or events. This data can also help in understanding regional popularity and usage patterns.
Player Demographics
- Account Information: When you create a game account, you provide info like your age, gender, and email. This data helps in personalizing the game experience and understanding the player base.
Data Sharing and Third-Party Services
- Third-Party Integrations: Games often use third-party services for analytics, social features, or cloud saves. These services collect data to provide their functionality, and they might share insights with the game developers.
machine learning projects
machine learning projects with source code
machine learning projects github
machine learning projects for final year
machine learning projects for students
Privacy and Control
- Privacy Policies: Games outline what data they collect and how it’s used in their privacy policies. It’s always a good idea to check these out to understand what’s happening with your data.
- User Control: Many games give you options to control data collection, like opting out of certain types of tracking or deleting your account data.
Data Collection in Video Games: Quick Reference
Type of Data | How It’s Collected | Purpose |
---|---|---|
Player Behavior | Gameplay analytics, heatmaps | Improve game design and engagement |
In-Game Events | Achievement tracking, choice recording | Personalize experience, track progress |
Social Interactions | Chat logs, friend lists, multiplayer data | Enhance social features, moderation |
Technical Data | Performance metrics, device info | Optimize performance, fix bugs |
User Feedback | In-game surveys, feedback forms | Direct player feedback |
Monetization Data | Purchase history, ad interactions | Optimize in-game economy, targeted ads |
Location Data | Geolocation tracking | Location-specific content, regional analysis |
Player Demographics | Account info (age, gender) | Personalization, demographic insights |
Third-Party Services | Analytics tools, cloud saves, social integrations | Enhance game features, additional insights |
Privacy Controls | User settings, privacy policies | Allow players to manage data collection |
machine learning projects reddit
reddit ai subreddit
machine learning interesting projects
good machine learning projects
What is gameplay data?
Hey there, gaming enthusiast! Let’s explore the fascinating world of gameplay data and uncover its secrets, just like embarking on a thrilling new level together. 🎮✨
What Exactly is Gameplay Data?
- Player Actions: Gameplay data encompasses all the actions you take within a game – every jump, every shot, every decision. It serves as a digital diary, meticulously recording your gaming adventures and keeping track of every move you make.
- Game Events: Whenever something significant occurs in the game, such as completing a level, unlocking an achievement, or discovering a hidden treasure, it becomes a part of the gameplay data. It’s like a logbook that documents your progress and celebrates your accomplishments.
- Interactions with Characters and Objects: Whether you’re engaging in conversations with non-player characters (NPCs), interacting with objects in the game’s environment, or making choices that shape the storyline, all these interactions are captured within the gameplay data.
- Performance Metrics: Additionally, gameplay data includes information about how the game performs on your device – from frame rates and loading times to any glitches or crashes you may encounter along the way. It’s akin to a report card that evaluates the game’s performance.
deep learning projects github
deep learning project github
github artificial intelligence projects
Why is Gameplay Data Important?
Game developers heavily rely on gameplay data to gain valuable insights into player engagement. They carefully analyze popular levels, player struggles, and feature usage to continuously improve and refine the game, ultimately creating a more immersive and enjoyable experience for everyone.
By closely monitoring gameplay data, games have the ability to personalize the gameplay according to your preferences.
For example, if you find a particular level challenging, the game may offer helpful hints or adjust the difficulty level to assist you in progressing further. It’s like having a game that adapts to your unique style and abilities.
The collection and analysis of gameplay data are essential for crafting captivating challenges and rewarding experiences that keep players hooked.
By understanding how players interact with the game, developers can create engaging quests, hidden surprises, and epic boss battles that entice players to continue playing and exploring the game’s world.
How is Gameplay Data Collected?
Games often come equipped with built-in analytics tools that automatically gather data on your gameplay. These tools keep track of various aspects, such as your movements, decisions, and performance metrics.
Additionally, games may prompt you to share your feedback through surveys or feedback forms. This direct input from players is incredibly valuable as it helps developers understand what aspects of the game are successful and what areas need improvement.
In some cases, games employ advanced techniques like heatmaps and telemetry to gather even more detailed gameplay data. Heatmaps provide insights into where players spend the most time or face the greatest challenges, while telemetry collects real-time data on game performance.
ml projects ideas
project manager artificial intelligence
best machine learning courses reddit
Conclusion
Through this blog, we walked through the process of exploring a video game dataset, deriving insights, and building a machine learning model to predict game genres.
machine learning projects for resume
machine learning project for resume
best machine learning projects
cool machine learning projects
This workflow demonstrates the power of data analysis and machine learning in uncovering patterns and making predictions.
6 Comments
Machine Learning Project 3: Best Explore Indian Cuisine · May 27, 2024 at 12:12 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]
Machine Learning Project 2: Diversity Tech Company Best EDA · May 27, 2024 at 12:14 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]
Machine Learning Project 1: Honda Motor Stocks Best Prices · May 27, 2024 at 12:14 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]
Machine Learning Project 5: Best Students Performance EDA · May 27, 2024 at 1:18 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]
ML Project 6: Obesity Type Best EDA And Classification · May 27, 2024 at 1:36 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]
Best ML Project: Machine Learning Engineer Salary In 2024 · May 28, 2024 at 6:19 pm
[…] Machine Learning Project 4: Exploring Video Game Data […]