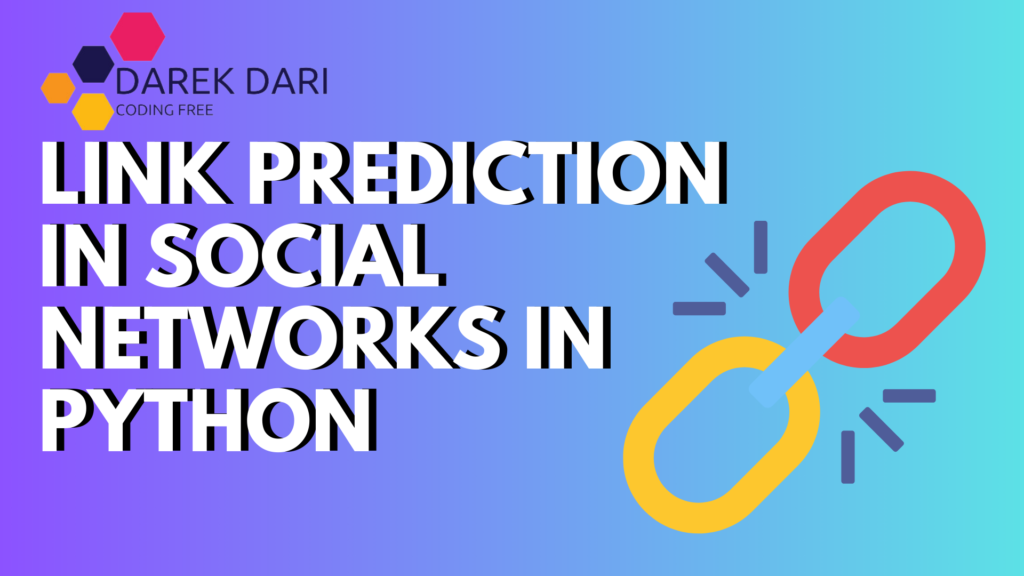
Table of Contents
Introduction to Link Prediction
Link prediction in social networks is a fascinating problem with numerous applications, from recommending new friends to identifying potential collaborations.
This blog post will guide you through a practical approach to link prediction, from data preparation to model evaluation, using Python.
Link prediction aims to estimate the likelihood of a connection forming between two entities in a network. For instance, in a social network like Facebook, it can predict if two users are likely to become friends based on their current interactions and connections. This prediction can enhance user experience by suggesting new connections or uncovering hidden relationships.
What is Link Prediction?
Link prediction involves forecasting whether a connection will form between two entities in a network in the future. It’s widely used in social networks to predict potential friendships, collaborations, or interactions.
Importance
- Enhances User Experience: Recommends new friends or connections.
- Improves Recommendations: Suggests potential partners or collaborators.
- Detects Anomalies: Identifies unusual or fraudulent connections.
Key Techniques
Technique | Description | Example Use Case |
---|---|---|
Common Neighbors | Measures the number of shared neighbors between nodes. | Predicting potential friends who have mutual acquaintances. |
Jaccard Coefficient | Calculates the ratio of shared neighbors to the total number of unique neighbors. | Identifying nodes with similar connectivity patterns. |
Adamic-Adar Index | Weighs less frequent neighbors more heavily, assuming they are more informative. | Recommending users who share rare interests or connections. |
These techniques help in identifying potential links by analyzing existing data and patterns within the network.
Step 1: Data Preparation
Data preparation is the first and crucial step in link prediction. You start by loading your dataset, which should contain information about nodes and links. For this, we use the load_data
function, which reads the data from a CSV file.
Once the data is loaded, it needs to be preprocessed. This involves cleaning the data by removing any missing values and converting categorical labels into binary format. The preprocess_data
function handles this by dropping rows with missing values and mapping link labels to binary values (1 for a link and 0 for no link).
Next, the data is split into training and testing sets using the split_data
function. This is done to evaluate the performance of our model on unseen data. By separating the data, we ensure that our model is tested on examples it hasn’t been trained on, providing a more accurate measure of its performance.
import pandas as pd
from sklearn.model_selection import train_test_split
def load_data(filepath):
"""Loads the dataset from a CSV file."""
data = pd.read_csv(filepath)
return data
def preprocess_data(data):
"""Preprocesses the data for link prediction."""
data = data.dropna() # Drop rows with missing values
data['label'] = data['label'].apply(lambda x: 1 if x == 'link' else 0)
return data
def split_data(data):
"""Splits the data into training and testing sets."""
X = data.drop('label', axis=1)
y = data['label']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
return X_train, X_test, y_train, y_test
Step 2: Feature Engineering
Feature engineering involves creating meaningful features from the raw data that can help the model make better predictions. For link prediction, common features include the number of common neighbors, the Jaccard coefficient, and the Adamic-Adar index.
The create_features
function generates these features for each pair of nodes. It calculates how many neighbors two nodes have in common, their Jaccard coefficient, and their Adamic-Adar index. These features capture different aspects of the relationship between nodes, helping the model understand the likelihood of a link forming between them.
import networkx as nx
import numpy as np
def create_features(graph, edges):
"""Generates features for link prediction based on the graph."""
features = []
for u, v in edges:
common_neighbors = len(list(nx.common_neighbors(graph, u, v)))
jaccard_coefficient = list(nx.jaccard_coefficient(graph, [(u, v)]))[0][2]
adamic_adar_index = list(nx.adamic_adar_index(graph, [(u, v)]))[0][2]
features.append([common_neighbors, jaccard_coefficient, adamic_adar_index])
return np.array(features)
Step 3: Model Training and Evaluation
With features prepared, we can train a model to predict links. The train_model
function uses a Random Forest Classifier, which is effective for classification tasks. This model learns from the training data to identify patterns and make predictions about whether a link will form.
After training the model, it’s essential to evaluate its performance using the testing data. The evaluate_model
function measures the model’s accuracy and provides a detailed classification report, including precision, recall, and F1-score. These metrics help us understand how well the model performs and where it may need improvements.
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score, classification_report
def train_model(X_train, y_train):
"""Trains a Random Forest model for link prediction."""
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
return model
def evaluate_model(model, X_test, y_test):
"""Evaluates the model's performance."""
y_pred = model.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
report = classification_report(y_test, y_pred)
return accuracy, report
Integrating All Components
To tie everything together, the main
function orchestrates the entire link prediction process. It starts by loading and preprocessing the data, then generates features from the graph. After that, it trains the model and evaluates its performance. This comprehensive approach ensures that all aspects of link prediction are covered.
def main():
filepath = 'social_network_data.csv'
# Load and preprocess data
data = load_data(filepath)
data = preprocess_data(data)
X_train, X_test, y_train, y_test = split_data(data)
# Create graph and generate features
graph = nx.from_pandas_edgelist(data, 'node1', 'node2', ['label'])
edges = [(row['node1'], row['node2']) for _, row in data.iterrows()]
features = create_features(graph, edges)
# Train and evaluate model
model = train_model(features, y_train)
accuracy, report = evaluate_model(model, X_test, y_test)
print(f"Model Accuracy: {accuracy:.2f}")
print("Classification Report:")
print(report)
if __name__ == "__main__":
main()
Frequently Asked Questions (FAQ)
Q: What is link prediction?
A: Link prediction is a task in social network analysis that involves predicting the likelihood of a connection forming between two entities in a network based on existing data.
Q: Why is feature engineering important in link prediction?
A: Feature engineering helps in creating informative and relevant features from the raw data, which can significantly improve the performance of the predictive model.
Q: What is the Jaccard coefficient?
A: The Jaccard coefficient is a metric used to measure the similarity between two nodes based on the proportion of common neighbors they share relative to the total number of unique neighbors.
Q: How does Random Forest Classifier work?
A: Random Forest is an ensemble learning method that combines multiple decision trees to improve classification accuracy. It works by averaging the predictions of individual trees to make a final decision.
Conclusion
Link prediction in social networks is a powerful technique with numerous applications. By following the steps outlined in this guide—data preparation, feature engineering, model training, and evaluation—you can build an effective link prediction model. Whether you’re recommending friends or exploring hidden connections, this approach provides a solid foundation for understanding and predicting relationships in a network.
Feel free to experiment with different features and models to further enhance your link prediction capabilities. With the right tools and techniques, you can uncover valuable insights and improve network interactions.
0 Comments