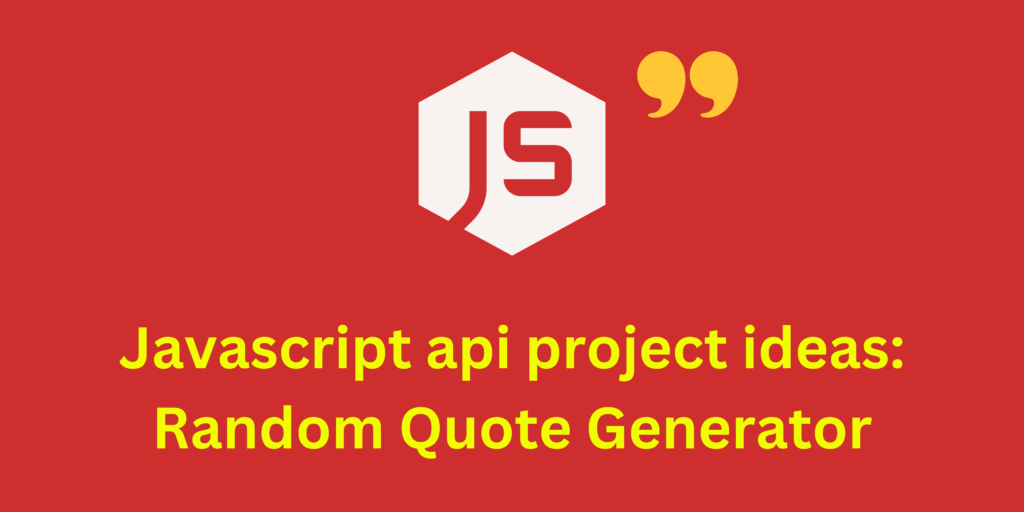
Javascript api project ideas. Build a web app that displays a random quote each time the user clicks a button. Use an API to fetch the quotes and display them on the screen.
Here is an example code for a Random Quote Generator web app using the Quote Garden API:
HTML for Javascript api project ideas: Random Quote Generator
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Random Quote Generator</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<div class="quote-container">
<div class="quote-text"></div>
<div class="quote-author"></div>
</div>
<button class="new-quote-btn">New Quote</button>
</div>
<script src="script.js"></script>
</body>
</html>
CSS for Javascript api project ideas: Random Quote Generator
.container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #f5f5f5;
}
.quote-container {
width: 400px;
height: 200px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
text-align: center;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.3);
}
.quote-text {
font-size: 1.5rem;
margin-bottom: 10px;
}
.quote-author {
font-size: 1rem;
font-style: italic;
}
.new-quote-btn {
padding: 10px 20px;
font-size: 1rem;
border: none;
border-radius: 5px;
background-color: #008CBA;
color: #fff;
cursor: pointer;
}
JavaScript for Javascript api project ideas: Random Quote Generator
const quoteText = document.querySelector('.quote-text');
const quoteAuthor = document.querySelector('.quote-author');
const newQuoteBtn = document.querySelector('.new-quote-btn');
function getQuote() {
fetch('https://quote-garden.herokuapp.com/api/v3/quotes/random')
.then(response => response.json())
.then(data => {
quoteText.textContent = data.data[0].quoteText;
quoteAuthor.textContent = `- ${data.data[0].quoteAuthor}`;
})
.catch(error => console.error(error));
}
newQuoteBtn.addEventListener('click', getQuote);
getQuote();
Explanation
In this example, we are using the Quote Garden API to fetch a random quote and display it on the screen.
We have a container div that contains the quote text and author, and a button that triggers the API call to fetch a new quote.
The getQuote() function makes a fetch request to the Quote Garden API and updates the DOM with the returned quote.
The event listener for the button calls the getQuote() function to fetch a new quote each time the button is clicked.
The initial quote is loaded by calling getQuote() when the page is loaded.
0 Comments