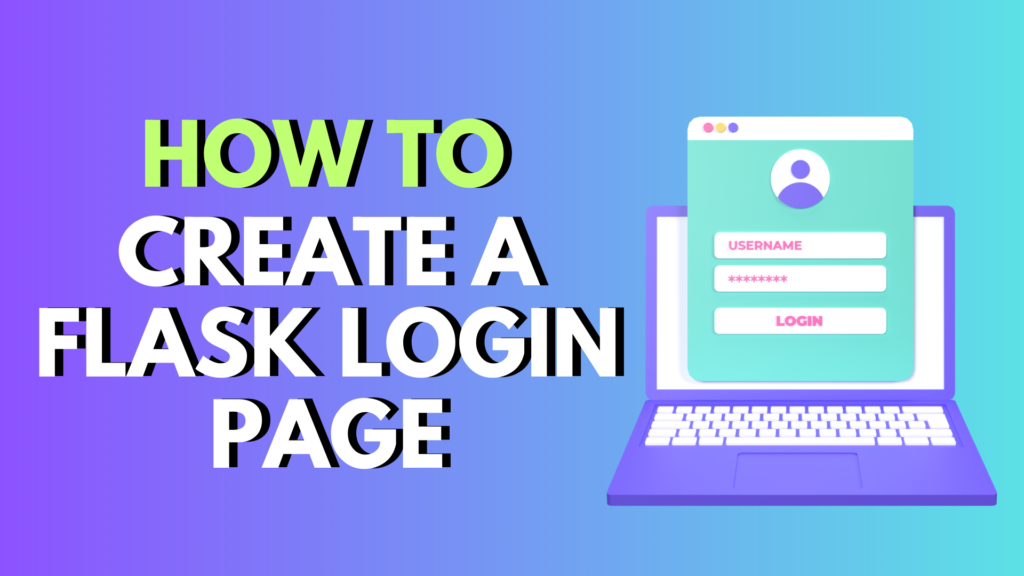
Introduction: Why Your Flask App Needs a Secure Login Page
In today’s digital age, user authentication is not just an option—it’s a necessity. Whether you’re building a personal project or launching a startup, implementing a secure and user-friendly Flask login page is key to protecting user data and managing sessions effectively.
In this comprehensive guide, we’ll walk you through everything you need to know about building a robust login system in Flask using Flask-Login, complete with real examples, GitHub resources, and best practices.
Table of Contents
- What Is Flask-Login?
- Setting Up Your Flask Login Page
- Flask-Login Example Project (GitHub)
- Creating a Flask Login Page Template
- Managing Sessions and
current_user
- Exploring the Flask-Login Documentation
- Interactive Coding Challenge
- Conclusion and Next Steps
What Is Flask-Login?
Flask-Login is a powerful user session management extension for Flask. It handles:
- User login and logout
- Session persistence
- Access control via decorators like
@login_required
- Easy access to the
current_user
object
Key Features:
- Simple integration with existing Flask apps
- Secure cookie-based session management
- Works with custom or SQLAlchemy user models
According to the Flask-Login documentation, over 10,000 GitHub projects use Flask-Login for secure user authentication.
📘 External Resource: Flask-Login Official Docs
Setting Up Your Flask Login Page
Let’s go through the steps to implement a login system using Flask and Flask-Login.
Step 1: Install Flask-Login
pip install flask flask-login
Step 2: Initialize Flask App and Flask-Login
from flask import Flask, render_template, redirect, url_for, request
from flask_login import LoginManager, login_user, login_required, logout_user, current_user, UserMixin
app = Flask(__name__)
app.secret_key = 'your_secret_key_here'
login_manager = LoginManager()
login_manager.init_app(app)
Step 3: Define a User Class
class User(UserMixin):
def __init__(self, id):
self.id = id
self.name = f"User{id}"
self.password = "password"
Step 4: User Loader
users = {'user1': User('user1')}
@login_manager.user_loader
def load_user(user_id):
return users.get(user_id)
Step 5: Login Route
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
user = users.get(username)
if user and user.password == password:
login_user(user)
return redirect(url_for('protected'))
return render_template('login.html')
Step 6: Protected Route
@app.route('/protected')
@login_required
def protected():
return f"Logged in as: {current_user.name}"
Step 7: Logout Route
@app.route('/logout')
@login_required
def logout():
logout_user()
return redirect(url_for('login'))
Flask-Login Example Project (GitHub)
Want to see the full example? Here’s a working login project on GitHub you can clone and explore:
🔗 GitHub Repo: Flask Login Example
Features in the GitHub Project:
- Complete user authentication flow
- Secure password storage with hashing
- Session handling and
current_user
usage
Creating a Flask Login Page Template
Here’s a basic Flask login page template (HTML) to get you started:
<!-- templates/login.html -->
<form method="post">
<label>Username:</label>
<input type="text" name="username" required><br>
<label>Password:</label>
<input type="password" name="password" required><br>
<button type="submit">Login</button>
</form>
You can customize this using Bootstrap or any CSS framework for a better user experience.
Managing Sessions and current_user
How Sessions Work in Flask-Login
Flask-Login stores session data in secure cookies. When a user logs in, Flask-Login:
- Generates a session ID
- Stores user ID in the session
- Allows access to the
current_user
proxy object
Accessing current_user
from flask_login import current_user
@app.route('/profile')
@login_required
def profile():
return f"Welcome, {current_user.name}!"
Pro Tip: Always use @login_required
to protect routes.
Exploring the Flask-Login Documentation
To dive deeper into advanced features like remember me cookies, anonymous users, and custom user classes, check out the official documentation:
Interactive Coding Challenge
🧠 Challenge: Add a “Remember Me” checkbox to your login form and enable session persistence in Flask-Login.
Hints:
- Add
remember=True
inlogin_user(user, remember=True)
- Update your HTML form with:
<input type="checkbox" name="remember"> Remember Me
Share your solution in the comments or fork the GitHub repo and submit a pull request!
Conclusion and Next Steps
Creating a secure and functional Flask login page is essential for any web app. By using Flask-Login, you simplify session management and user authentication while keeping your app secure and maintainable.
✅ Key Takeaways:
- Use Flask-Login to manage sessions and
current_user
- Protect routes with
@login_required
- Customize your login page with HTML/CSS
- Explore real-world examples and GitHub projects
📢 Call to Action: Ready to build your own login system? Start coding now or explore our Flask tutorials and GitHub resources to level up your Python web development skills!
Don’t forget to share this guide with your fellow devs or bookmark it for future reference!
- Flask CRUD App Tutorial
- How to Use SQLAlchemy with Flask
- User Authentication in Django vs Flask
- Flask Documentation
- Flask-Login GitHub
- Miguel Grinberg’s Flask Tutorial
0 Comments