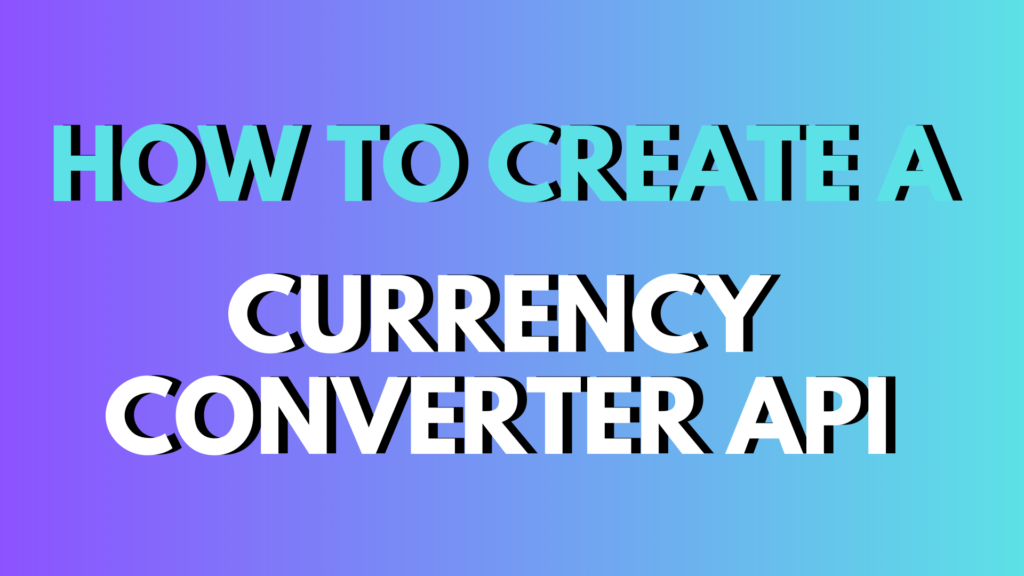
Introduction
With the rise of global transactions, having a reliable currency converter API is essential for businesses, financial apps, and e-commerce platforms. Whether you’re building a real-time exchange rate service or a personal currency conversion tool, creating your own API can provide flexibility and control over your data.
In this guide, we’ll walk you through the process of building a currency converter API, including fetching exchange rates, handling API requests, and deploying your service. By the end, you’ll have a working currency conversion API that can be integrated into web and mobile applications.
What is a Currency Converter API?
A currency converter API is a web service that allows users to retrieve exchange rates and perform currency conversions in real time. These APIs can fetch data from sources like forex markets, central banks, or third-party services to provide up-to-date rates.
Key Features of a Currency Converter API
- Real-time exchange rates
- Historical exchange data
- Support for multiple currencies
- Conversion calculations
- JSON-based responses for easy integration
How to create currency converter api python
How to create currency converter api using python
How to create currency converter api in javascript
Currency converter API free
Free exchange rate API Google
Open API for exchange rates
Exchange rate API documentation
Currency API JSON
Step 1: Choosing the Right Data Source
Before coding your API, you need a reliable forex data provider. Here are some popular options:
- ExchangeRatesAPI.io – Free and paid plans for exchange rate data.
- Open Exchange Rates – Provides accurate, real-time forex data.
- CurrencyLayer – Offers a robust API for currency conversion.
- Fixer.io – Trusted by many developers for forex exchange rates.
- European Central Bank (ECB) – Free and reliable exchange rate provider.
- Free exchange rate API Google – Google’s free exchange rate API may offer useful data.
- Open API for exchange rates – Open-source and publicly accessible APIs for forex data.
Step 2: Setting Up Your Development Environment
To build a currency converter API, we’ll use Python and Flask to handle requests. Ensure you have Python 3.x installed, then install Flask:
pip install flask requests
We’ll also use the requests
library to fetch exchange rate data from an external provider.
Step 3: Writing the API Code
Below is a basic currency converter API using Flask and an external API (ExchangeRatesAPI.io) for forex data. This will help you understand How to create a currency converter API using Python:
from flask import Flask, request, jsonify
import requests
app = Flask(__name__)
API_KEY = "your_api_key" # Replace with your forex API key
BASE_URL = "https://api.exchangeratesapi.io/latest"
@app.route('/convert', methods=['GET'])
def convert_currency():
from_currency = request.args.get('from')
to_currency = request.args.get('to')
amount = float(request.args.get('amount'))
response = requests.get(f"{BASE_URL}?access_key={API_KEY}")
data = response.json()
if from_currency in data['rates'] and to_currency in data['rates']:
rate = data['rates'][to_currency] / data['rates'][from_currency]
converted_amount = amount * rate
return jsonify({
'from_currency': from_currency,
'to_currency': to_currency,
'amount': amount,
'converted_amount': round(converted_amount, 2)
})
else:
return jsonify({'error': 'Invalid currency code'}), 400
if __name__ == '__main__':
app.run(debug=True)
Step 4: How to Create a Currency Converter API in JavaScript
If you prefer to use JavaScript, you can create a simple currency converter API using Node.js and Express:
const express = require('express');
const axios = require('axios');
const app = express();
const API_KEY = 'your_api_key'; // Replace with your forex API key
const BASE_URL = 'https://api.exchangeratesapi.io/latest';
app.get('/convert', async (req, res) => {
try {
const { from, to, amount } = req.query;
const response = await axios.get(`${BASE_URL}?access_key=${API_KEY}`);
const rates = response.data.rates;
if (rates[from] && rates[to]) {
const rate = rates[to] / rates[from];
const convertedAmount = amount * rate;
res.json({ from, to, amount, convertedAmount: convertedAmount.toFixed(2) });
} else {
res.status(400).json({ error: 'Invalid currency code' });
}
} catch (error) {
res.status(500).json({ error: 'API request failed' });
}
});
app.listen(3000, () => console.log('Server running on port 3000'));
Is there an API for currency conversion?
How do I make a currency converter app?
Is ExchangeRate API free?
Does Google have a currency converter API?
Step 5: Deploying the Currency Converter API
To make your API accessible online, deploy it using:
- Flask + Gunicorn + Heroku (Simple and free option)
- FastAPI + Uvicorn + Docker (For scalable deployment)
- AWS Lambda + API Gateway (Serverless option)
Example of deploying with Heroku:
git init
git add .
git commit -m "Initial commit"
heroku create currency-converter-api
git push heroku main
Your API will now be live at:
https://currency-converter-api.herokuapp.com/convert?from=USD&to=EUR&amount=100
Step 6: Enhancing Your API with Additional Features
To make your API more powerful, consider adding:
- Historical Exchange Rates – Fetch past exchange rates for analysis.
- Cryptocurrency Support – Convert between fiat and crypto (e.g., Bitcoin to USD).
- User Authentication – Implement API keys to control access.
- Rate Limits & Caching – Improve performance and reduce API calls.
Best Practices for Building a Currency Converter API
✅ Use a reliable data provider to ensure accurate exchange rates.
✅ Implement error handling to manage invalid inputs and API failures.
✅ Optimize performance by caching frequent requests.
✅ Secure your API using authentication and rate limits.
✅ Monitor API usage to detect potential issues early.
Conclusion
Creating a currency converter API is a valuable skill for developers working with financial applications. By following this guide, you can build, test, and deploy a functional API that provides real-time exchange rates.
Call to Action
🚀 Ready to build your own currency converter API? Follow this guide and start coding today! If you found this article helpful, share it with fellow developers and let us know in the comments what additional features you’d like to see! 💰💻
0 Comments