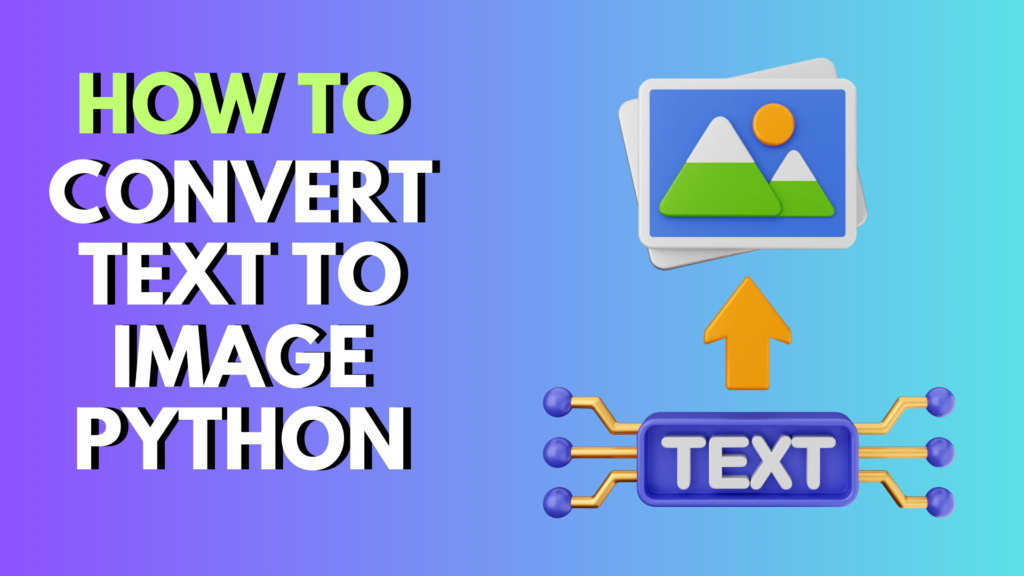
Introduction: Why Convert Text to Image?
In today’s digital landscape, visuals are essential for communication, marketing, and content creation. Whether you’re designing social media graphics, preparing presentations, or sharing quotes online, converting text into an image offers creative and functional advantages.
By turning text into an image, you can:
- Increase engagement on social media platforms that prioritize visual content
- Protect your text from being easily copied or scraped
- Add visual styling, branding, and design elements to plain text
- Share content on platforms that don’t support plain-text formatting
If you’re wondering how to convert text to an image—whether it’s a JPG, PNG, or embedded graphic—this guide covers all the methods you need to know.
Python code to convert text into an image (PNG or JPG):
from PIL import Image, ImageDraw, ImageFont
# --- Settings ---
text = "Hello, this is text converted into an image!"
font_path = "arial.ttf" # Update with the path to a valid .ttf font file
font_size = 40
image_width = 800
image_height = 200
text_color = (0, 0, 0) # Black text
background_color = (255, 255, 255) # White background
output_filename = "output_image.png" # Change to .jpg for JPG
# --- Create image ---
image = Image.new('RGB', (image_width, image_height), color=background_color)
draw = ImageDraw.Draw(image)
# Load font
try:
font = ImageFont.truetype(font_path, font_size)
except IOError:
font = ImageFont.load_default()
print("Custom font not found. Using default font.")
# Calculate text size and position
text_width, text_height = draw.textsize(text, font=font)
x = (image_width - text_width) / 2
y = (image_height - text_height) / 2
# Draw the text onto the image
draw.text((x, y), text, fill=text_color, font=font)
# Save the image
image.save(output_filename)
print(f"Image saved as {output_filename}")
📝 Instructions:
- Install Pillow (if not already installed):
pip install pillow
- Save the code in a
.py
file. - Make sure you have a
.ttf
font file likearial.ttf
in your directory or specify the correct font path (e.g.,"C:/Windows/Fonts/arial.ttf"
on Windows). - Run the script → it will generate an image file like
output_image.png
with your text.
💡 Customizations:
- Change
image_width
andimage_height
to adjust the image size. - Modify
font_size
,text_color
, andbackground_color
as needed. - You can switch the output filename extension from
.png
to.jpg
if you prefer JPG output.
Methods to Convert Text to Image
1. Use Online Text-to-Image Converters
The fastest and most convenient way to turn text into an image is by using free online tools. These platforms allow you to type or paste text, customize fonts and backgrounds, and download your image in various formats such as JPG or PNG.
Popular online converters include:
Tool | Features |
---|---|
SmallSEOTools | Choose font size, color, and background; export as JPG/PNG |
Convertio | Directly converts TXT files to JPG format |
Online-Convert | Advanced options like DPI settings and resizing |
0 Comments