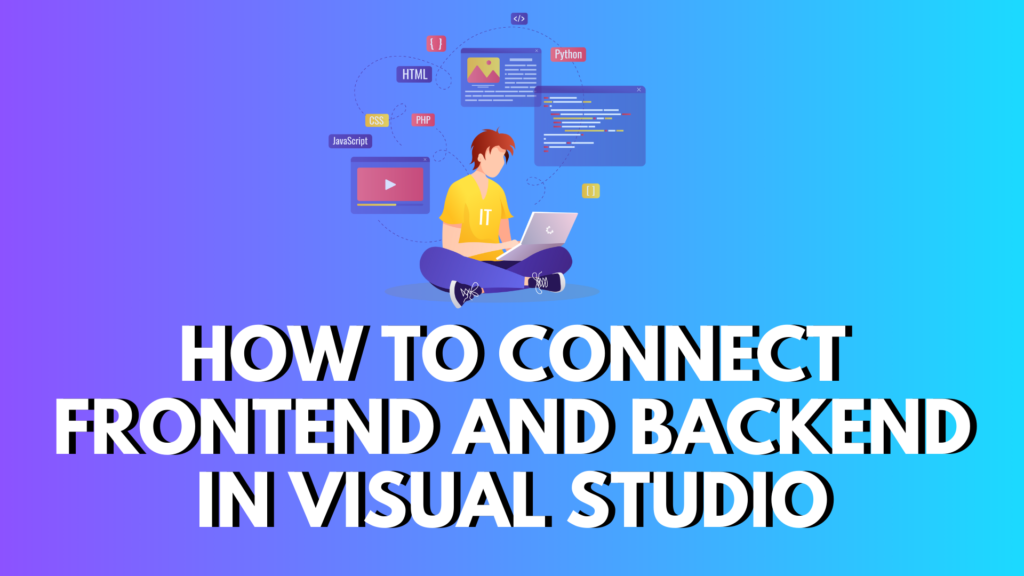
Connecting the frontend and backend of your application is one of the most critical steps in web development.
Whether you’re working on a project in React, Node.js, or Python, ensuring seamless communication between these layers can make or break your app’s functionality.
In this blog post, we’ll dive deep into how to connect the frontend and backend in Visual Studio (VS) and Visual Studio Code (VS Code), step-by-step, while exploring different technologies like React, Node.js, JavaScript, and more.
How to connect frontend and backend in visual studio 2022
How to connect frontend and backend in Visual Studio Code
How to connect frontend and backend in visual studio without
How to connect frontend and backend in visual studio using
How to connect frontend and backend in React JS
How to connect frontend and backend in Node JS
How to connect frontend and backend in JavaScript
How to connect frontend and backend in Python
Introduction: Why Connecting Frontend and Backend Matters
When developing web applications, the frontend refers to the user interface—the part that users interact with. On the other hand, the backend handles the logic, databases, and data management behind the scenes. To create fully functional applications, it’s essential to connect the two effectively.
But how do you link them together? How does data flow from the backend to the frontend seamlessly? And more importantly, how can you do this efficiently within an IDE like Visual Studio or Visual Studio Code?
In this article, we’ll guide you through the process, ensuring that you not only learn the basics but also master the key steps for syncing your frontend and backend across various technologies. Let’s get started!
How to Connect Frontend and Backend in Visual Studio Code
Visual Studio Code (VS Code) is a powerful, lightweight IDE that is popular for both frontend and backend development. Let’s assume you are working on a full-stack project where the frontend is built with React and the backend with Node.js using Express.
Step 1: Set up the Backend (Node.js with Express)
Start by creating the backend with Node.js and Express.js. Here’s how to do it:
1.1. Install Node.js and Express
If you haven’t already installed Node.js, download and install it from here. After installing, open your terminal and run the following commands to set up the backend:
# Initialize a new Node.js project
mkdir my-fullstack-app
cd my-fullstack-app
npm init -y
# Install Express.js to create the backend server
npm install express
1.2. Create server.js
to Handle API Requests
Inside your project folder, create a server.js
file. This will set up an Express server that listens for incoming HTTP requests.
// server.js (Backend Code)
const express = require('express');
const app = express();
const port = 5000;
// Middleware for handling JSON data
app.use(express.json());
// Example of a simple GET route
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello from the backend!' });
});
// POST route to receive data
app.post('/api/data', (req, res) => {
const receivedData = req.body;
console.log(receivedData);
res.json({ status: 'Data received', data: receivedData });
});
// Start the server
app.listen(port, () => {
console.log(`Backend running on http://localhost:${port}`);
});
1.3. Run the Backend
Now, run your backend server with the following command:
node server.js
Your backend should be running on http://localhost:5000
. You can test this by visiting http://localhost:5000/api/data
in your browser. You should see the response { "message": "Hello from the backend!" }
.
Step 2: Set up the Frontend (React)
Now, let’s set up the frontend with React.
2.1. Create a React App
If you don’t have React set up yet, you can create a new React app by running:
npx create-react-app frontend
cd frontend
npm start
This will create a new React app and run it on http://localhost:3000
.
2.2. Install Axios for HTTP Requests
We’ll use Axios to make HTTP requests from the frontend to the backend. Install Axios by running:
npm install axios
2.3. Create a React Component that Calls the Backend
Create a component, App.js
, to fetch data from the backend API:
// App.js (Frontend Code)
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const App = () => {
const [data, setData] = useState(null);
const [postData, setPostData] = useState({ name: 'John Doe', age: 30 });
// Fetch data from the backend when the component mounts
useEffect(() => {
axios.get('http://localhost:5000/api/data')
.then(response => {
console.log('Data from backend:', response.data);
setData(response.data.message);
})
.catch(error => {
console.error('Error fetching data:', error);
});
}, []);
// Send data to the backend when the form is submitted
const handleSubmit = (e) => {
e.preventDefault();
axios.post('http://localhost:5000/api/data', postData)
.then(response => {
console.log('Data sent to backend:', response.data);
})
.catch(error => {
console.error('Error sending data:', error);
});
};
return (
<div>
<h1>Frontend-Backend Integration</h1>
<p>Data from Backend: {data}</p>
<form onSubmit={handleSubmit}>
<input
type="text"
value={postData.name}
onChange={e => setPostData({ ...postData, name: e.target.value })}
/>
<button type="submit">Send Data to Backend</button>
</form>
</div>
);
};
export default App;
2.4. Run the React App
Ensure your React app is running on http://localhost:3000
. Now, when the React app loads, it will make a GET request to http://localhost:5000/api/data
to retrieve data from the backend.
When the form is submitted, it will make a POST request to send data to the backend.
How to connect frontend and backend in VS Code?
How do I connect both frontend and backend?
How to sync frontend and backend?
How do I call the backend from the frontend?
How frontend and backend communicate with each other?
How do I switch from front end to backend?
How to Call the Backend from the Frontend
In our example, we used Axios to make both GET and POST requests.
GET Request Example
axios.get('http://localhost:5000/api/data')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data from backend:', error);
});
POST Request Example
axios.post('http://localhost:5000/api/data', { name: 'Jane Doe', age: 25 })
.then(response => {
console.log('Response from backend:', response.data);
})
.catch(error => {
console.error('Error sending data to backend:', error);
});
These are the basic ways to call the backend from the frontend using Axios.
Syncing Frontend and Backend in React
In React, we can sync the frontend and backend by fetching data and rendering it dynamically. Here’s an advanced example:
Dynamic Data Fetching Example:
useEffect(() => {
const fetchData = async () => {
try {
const result = await axios.get('http://localhost:5000/api/data');
setData(result.data.message);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
This example uses async
/await
to fetch data from the backend asynchronously and update the state with the response.
How to Connect Frontend and Backend in Node.js (Example with Express)
Here’s how you set up a Node.js backend using Express.js to serve data to the frontend. We already did this in the previous steps, but here’s a detailed breakdown of routing.
// server.js (Backend Code)
const express = require('express');
const app = express();
// Set up a simple GET route
app.get('/api/data', (req, res) => {
res.json({ message: 'Hello, this is the backend responding!' });
});
// Start the server
app.listen(5000, () => {
console.log('Server is running on http://localhost:5000');
});
Here, when the frontend makes a GET request to http://localhost:5000/api/data
, the backend responds with a JSON message.
Using APIs to Connect Frontend and Backend
APIs (specifically REST APIs) are the most common way to connect the frontend and backend.
Example with Fetch API:
fetch('http://localhost:5000/api/data')
.then(response => response.json())
.then(data => console.log('Backend Data:', data))
.catch(error => console.error('Error:', error));
This is a basic example using the **Fetch API** to send a **GET** request.
Conclusion & Call to Action
Connecting the frontend and backend is a key skill for full-stack development. By using technologies like React, Node.js, Express, and Axios, you can build robust applications that communicate seamlessly between the client and server.
Best Practices:
- Use environment variables for sensitive data.
- Implement error handling on both frontend and backend.
- Secure your API endpoints with authentication (e.g., JWT).
- Test endpoints with Postman before integrating them with the frontend.
Now that you have a clear understanding of how to connect frontend and backend in Visual Studio Code, it’s time to put this knowledge into practice. Start building your full-stack projects and integrate them smoothly.
If you have any questions or want to share your experiences, feel free to leave a comment below!
0 Comments