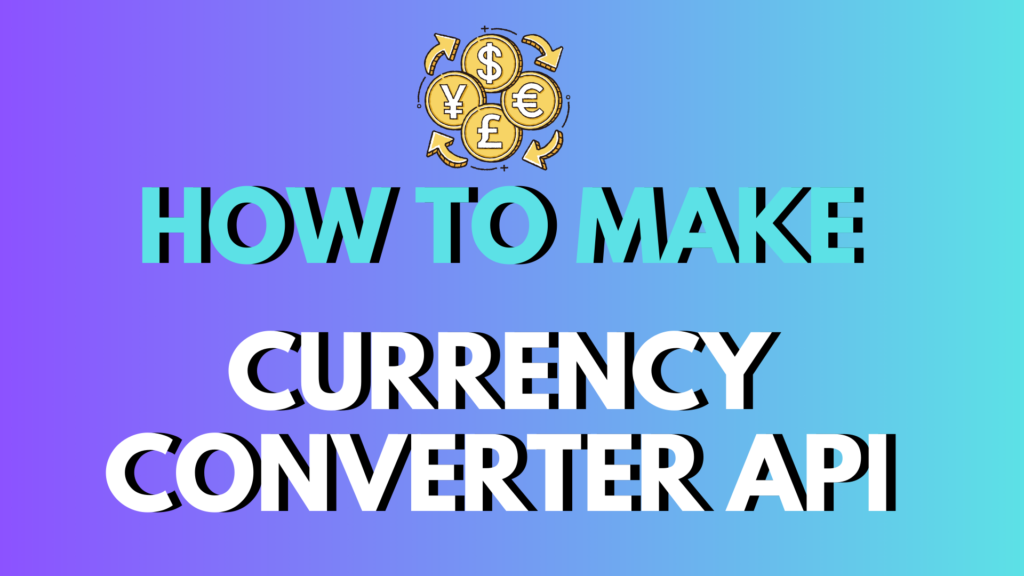
Is there an API for currency conversion?
Yes, there are several APIs available for currency conversion, such as ExchangeRate-API, Fixer.io, CurrencyLayer, Open Exchange Rates, and Exchangerate.host. These APIs allow you to fetch real-time and historical exchange rates.
How do I make a currency converter app?
You can create a currency converter app using programming languages like Python or JavaScript. Here’s a basic approach:
- Use an API like ExchangeRate-API to get live exchange rates.
- Create a front-end interface (using HTML, CSS, and JavaScript).
- Use JavaScript’s Fetch API or Python’s
requests
module to get data from the API. - Display the converted amount based on user input.
Is ExchangeRate API free?
Yes, ExchangeRate-API offers a free tier with limited requests per month. However, for higher request limits and more features, they provide paid plans.
Does Google have a currency converter API?
No, Google does not provide a dedicated public API for currency conversion. While you can see currency conversion in Google search or Google Finance, there is no official API for developers.
How to create a currency converter API using Python?
To create your own currency converter API in Python:
- Use
Flask
to create a simple web API. - Fetch exchange rates from a reliable source (e.g., ExchangeRate-API).
- Create an endpoint that accepts parameters (e.g.,
amount
,from_currency
,to_currency
). - Return the converted value in JSON format.
Example code snippet in Python (using Flask):
from flask import Flask, request, jsonify
import requests
app = Flask(__name__)
API_URL = "https://api.exchangerate-api.com/v4/latest/USD"
@app.route('/convert', methods=['GET'])
def convert_currency():
amount = float(request.args.get('amount'))
from_currency = request.args.get('from')
to_currency = request.args.get('to')
response = requests.get(API_URL).json()
rates = response["rates"]
if from_currency in rates and to_currency in rates:
converted_amount = (amount / rates[from_currency]) * rates[to_currency]
return jsonify({"converted_amount": converted_amount})
return jsonify({"error": "Invalid currency code"}), 400
if __name__ == '__main__':
app.run(debug=True)
How to create a currency converter API in JavaScript?
You can use Node.js and Express to create a simple currency conversion API. Here’s a basic example:
const express = require('express');
const fetch = require('node-fetch');
const app = express();
const API_URL = "https://api.exchangerate-api.com/v4/latest/USD";
app.get('/convert', async (req, res) => {
const { amount, from, to } = req.query;
const response = await fetch(API_URL);
const data = await response.json();
if (data.rates[from] && data.rates[to]) {
const convertedAmount = (amount / data.rates[from]) * data.rates[to];
res.json({ converted_amount: convertedAmount });
} else {
res.status(400).json({ error: "Invalid currency code" });
}
});
app.listen(3000, () => console.log('Server running on port 3000'));
This API listens on port 3000 and converts currency using exchange rates.
What is a Forex API?
A Forex API provides real-time foreign exchange rate data. It allows developers to access forex market rates for use in financial applications, trading platforms, and currency conversion services.
How do I get a conversion API token?
To get an API token for a currency conversion service:
- Register on a provider’s website (e.g., ExchangeRate-API, Fixer.io).
- Verify your email if required.
- Obtain your unique API key (token) from the dashboard.
- Use the API key in your requests to authenticate.
Example API request:
https://v6.exchangerate-api.com/v6/YOUR-API-KEY/latest/USD
How to check if a conversion API is working?
You can check if your currency conversion API is working using:
- Postman: Send a test request to your API and check the response.
- cURL command: Run in the terminal:
curl -X GET "https://api.exchangerate-api.com/v4/latest/USD"
- Web browser: Enter the API URL in the address bar and verify the JSON response.
Is there a free exchange rate API?
Yes, there are free exchange rate APIs with limited requests, such as:
- ExchangeRate-API (free for up to 1,000 requests/month)
- Exchangerate.host (free & open-source)
- Open Exchange Rates (free plan available)
For higher limits and additional features, most services offer paid plans.
0 Comments