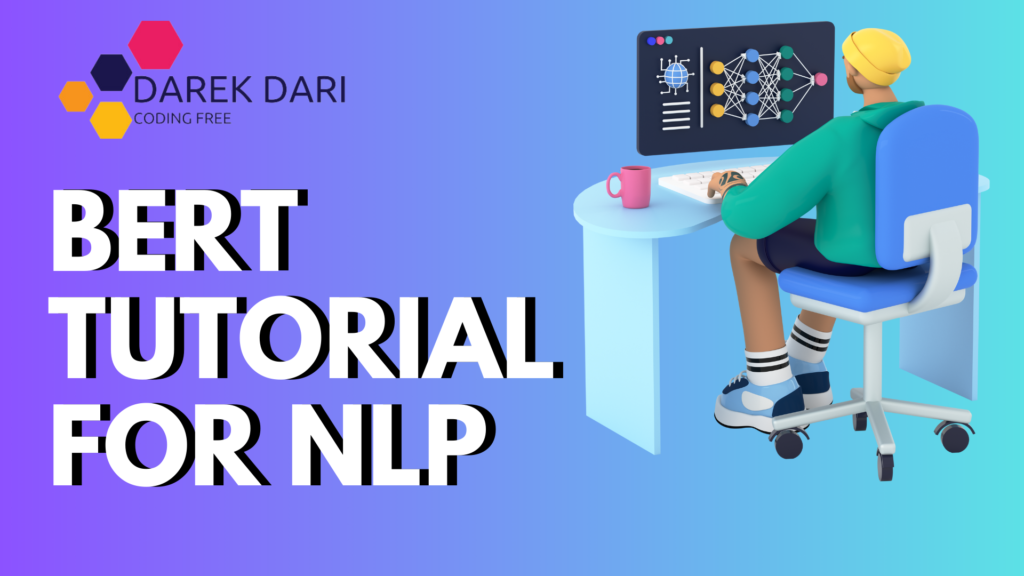
Table of Contents
Introduction to Fine-Tuning BERT for Text Classification
Welcome to the Fine-Tuning BERT for Text Classification guide!
- BERT (Bidirectional Encoder Representations from Transformers) has completely transformed Natural Language Processing (NLP) by its ability to comprehend the context of words in a sentence. In this tutorial, we will fine-tune a pre-trained BERT model for text classification, specifically for sentiment analysis using the IMDB movie reviews dataset. We’ll cover:
- Getting Started: Installing the necessary libraries.
- Model Loading: Utilizing a pre-trained BERT model and tokenizer.
- Tokenization: Preparing text data for BERT.
- Prediction: Obtaining predictions from the pre-trained model.
- Fine-Tuning: Customizing BERT for text classification.
- Evaluation: Assessing model performance.
- Prediction on New Data: Classifying new text data.
By the end, you’ll have the ability to fine-tune BERT for various text classification tasks, harnessing its powerful contextual understanding for high-accuracy predictions.
What is BERT and how to use it?
BERT, a highly intelligent language model created by Google, has the ability to comprehend text in both directions simultaneously—left-to-right and right-to-left. This unique feature allows BERT to grasp the true meaning of words within their context, making it exceptionally proficient in various language-related tasks.
To utilize BERT effectively, you must initially install essential libraries such as transformers
and torch
. These tools enable you to access BERT’s pre-trained models and convert text into a suitable format for the model.
Once the setup is complete, you can load BERT along with its tokenizer, which converts your text into tokens that BERT can interpret.
Subsequently, you can input this tokenized text into BERT to obtain predictions or embeddings for your specific task, whether it involves text classification, sentiment analysis, or any other objective.
For tasks requiring additional customization, you have the option to fine-tune BERT using a specific dataset to enhance its performance according to your requirements.
Once fine-tuned, BERT is well-equipped to handle new text data efficiently, providing you with precise and insightful outcomes due to its profound understanding of language.
Steps to Start Coding
Step 1: Install the Necessary Libraries
First, you need to install the transformers
and torch
libraries. The transformers
library by Hugging Face provides pre-trained models, and torch
(PyTorch) is a popular deep learning library.
pip install transformers torch
bert tutorial
bert tutorial nlp
bert tutorial
bert models
how to use bert
bert python
Step 2: Loading a Pre-trained BERT Model
In this step, we load the pre-trained BERT model and its tokenizer. The tokenizer converts text into the format the BERT model expects (token IDs, attention masks, etc.).
from transformers import BertTokenizer, BertModel
# Load pre-trained model tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
# Load pre-trained model
model = BertModel.from_pretrained('bert-base-uncased')
We use bert-base-uncased
, a version of BERT that is case-insensitive (it treats “apple” and “Apple” the same way).
Step 3: Tokenizing Input Text
Tokenization is the process of converting a string of text into tokens (smaller pieces, like words or subwords) that the model can process.
import torch
# Define a sample sentence
sentence = "Hello, BERT is amazing!"
# Tokenize the sentence
tokens = tokenizer(sentence, return_tensors='pt')
# Print the tokens
print(tokens)
In the code above, sentence
is the input text we want to process. The function tokenizer(sentence, return_tensors='pt')
tokenizes the input text and returns the result as PyTorch tensors.
The tokens
dictionary contains:
input_ids
: The token IDs for the input text.attention_mask
: Indicates which tokens should be attended to (1 for real tokens, 0 for padding tokens).
python bert
bert example
bert examples
use bert
bert model python
bert nlp tutorial
Step 4: Getting the Model’s Predictions
Now, we pass the tokenized input to the BERT model to get predictions.
# Get the hidden states from the model
with torch.no_grad():
outputs = model(**tokens)
# The last hidden state
last_hidden_state = outputs.last_hidden_state
# Print the shape of the last hidden state
print(last_hidden_state.shape)
Here, model(**tokens)
passes the tokenized input to the model, and outputs
is a dictionary containing the model’s output. The last_hidden_state
is a tensor representing the hidden states for each token in the input.
Step 5: Fine-Tuning BERT for Text Classification
Fine-tuning adapts a pre-trained model to a specific task. We’ll fine-tune BERT for text classification using the IMDB movie reviews dataset.
Step 5.1: Load the Dataset
We use the datasets
library to load the IMDB dataset.
pip install datasets
from datasets import load_dataset
# Load the IMDB dataset
dataset = load_dataset('imdb')
Step 5.2: Preprocess the Dataset
We need to tokenize the dataset and split it into training and testing sets.
# Tokenize the dataset
def tokenize_function(examples):
return tokenizer(examples['text'], padding='max_length', truncation=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
# Split the dataset into train and test sets
train_dataset = tokenized_datasets['train']
test_dataset = tokenized_datasets['test']
In this section, tokenize_function
tokenizes each example in the dataset. The function dataset.map(tokenize_function, batched=True)
applies the tokenization function to the entire dataset. We then split the tokenized dataset into training and testing sets.
Step 5.3: Define the Model
We use BertForSequenceClassification
, which is a BERT model with a classification head on top.
from transformers import BertForSequenceClassification
# Define the model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
BertForSequenceClassification
is used for classification tasks. The parameter num_labels=2
specifies that we have two classes (positive and negative sentiment).
Step 5.4: Training the Model
We use the Trainer
API to train the model.
from transformers import Trainer, TrainingArguments
# Define training arguments
training_args = TrainingArguments(
output_dir='./results',
evaluation_strategy='epoch',
learning_rate=2e-5,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
num_train_epochs=3,
weight_decay=0.01,
)
# Define the Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset,
)
# Train the model
trainer.train()
TrainingArguments
defines the training configuration, including output_dir
to specify where to save the model, evaluation_strategy='epoch'
to evaluate the model at the end of each epoch, and other hyperparameters like learning_rate
, batch_size
, num_train_epochs
, and weight_decay
. Trainer
is responsible for training the model, and trainer.train()
starts the training process.
Step 6: Evaluate the Model
We evaluate the trained model on the test set.
# Evaluate the model
results = trainer.evaluate()
print(results)
trainer.evaluate()
evaluates the model on the test set and returns the results.
Step 7: Making Predictions
We use the fine-tuned model to make predictions on new text data.
# Define a sample sentence
sample_sentence = "This movie was absolutely fantastic!"
# Tokenize the sentence
tokens = tokenizer(sample_sentence, return_tensors='pt')
# Get predictions from the model
with torch.no_grad():
outputs = model(**tokens)
# Get the predicted class
predictions = torch.argmax(outputs.logits, dim=-1)
# Print the predicted class
print(f'Predicted class: {predictions.item()}')
First, we tokenize the new text. Then, model(**tokens)
gets the model’s predictions, and torch.argmax(outputs.logits, dim=-1)
finds the class with the highest score. Finally, predictions.item()
gets the predicted class label.
Complete Code
Here’s the complete code again, incorporating all the steps:
from transformers import BertTokenizer, BertModel, BertForSequenceClassification, Trainer, TrainingArguments
from datasets import load_dataset
import torch
# Load pre-trained model tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
# Load the IMDB dataset
dataset = load_dataset('imdb')
# Tokenize the dataset
def tokenize_function(examples):
return tokenizer(examples['text'], padding='max_length', truncation=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
# Split the dataset into train and test sets
train_dataset = tokenized_datasets['train']
test_dataset = tokenized_datasets['test']
# Define the model
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
# Define training arguments
training_args = TrainingArguments(
output_dir='./results',
evaluation_strategy='epoch',
learning_rate=2e-5,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
num_train_epochs=3,
weight_decay=0.01,
)
# Define the Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_dataset,
eval_dataset=test_dataset,
)
# Train the model
trainer.train()
# Evaluate the model
results = trainer.evaluate()
print(results)
# Define a sample sentence
sample_sentence = "This movie was absolutely fantastic!"
# Tokenize the sentence
tokens = tokenizer(sample_sentence, return_tensors='pt')
# Get predictions from the model
with torch.no_grad():
outputs = model(**tokens)
# Get the predicted class
predictions = torch.argmax(outputs.logits, dim=-1)
# Print the predicted class
print(f'Predicted class: {predictions.item()}')
This expanded explanation provides a more detailed walkthrough of each step, from loading and tokenizing data to training, evaluating, and making predictions with a fine-tuned BERT model.
Is Google BERT Free to Use?
Yes google’s BERT model is available for free use. The pre-trained BERT models and their tokenizers can be accessed through the Hugging Face transformers
library.
These models are suitable for various natural language processing tasks, whether for research, personal projects, or commercial applications, all at no cost.
It’s important to note that while the models themselves are free, there may be additional expenses depending on how you utilize them.
For instance, running extensive inference or training tasks on cloud services could result in charges from those platforms.
Moreover, fine-tuning BERT on a large dataset or using it extensively may require significant computational resources, potentially leading to expenses related to cloud computing or hardware usage.
Is BERT better than LSTM?
BERT and LSTM models are commonly used in natural language processing, each with its own set of advantages and disadvantages.
Let’s take a look at how they compare to see when BERT might outperform LSTM, and vice versa.
Feature | BERT | LSTM |
---|---|---|
Context Understanding | Reads text bidirectionally, capturing context from both directions | Reads text sequentially, which can limit context understanding |
Pre-training | Pre-trained on large corpora, providing a strong starting point for fine-tuning | Typically requires training from scratch on specific tasks |
Performance | Often achieves higher accuracy on NLP tasks like sentiment analysis, question answering, and named entity recognition | May achieve lower accuracy compared to BERT, especially on complex tasks |
Computational Efficiency | Can be computationally expensive due to large model size and pre-training | Generally less computationally expensive and can be more efficient for smaller datasets |
Handling Long-Term Dependencies | Effective at capturing long-term dependencies through bidirectional context | Handles long-term dependencies, though may struggle with very long sequences without careful tuning |
Training Requirements | Requires substantial resources for pre-training but less for fine-tuning | Can be resource-intensive and requires careful management of gradients and training data |
Task Suitability | Suitable for a wide range of NLP tasks with high performance | Useful for sequential tasks where bidirectional context is less critical |
BERT typically delivers better results and comprehension of context thanks to its bidirectional processing and pre-training, which enhances its effectiveness across various NLP tasks.
While LSTMs can still be useful, particularly in scenarios with resource constraints or when sequential processing suffices.
Is BERT still used?
Yes, BERT is still very much in use and remains a key player in the world of Natural Language Processing (NLP). Since its release, BERT has set a high bar for understanding language thanks to its clever bidirectional approach. It’s still a go-to model for many tasks, like sentiment analysis, text classification, and question answering.
Even with the rise of newer models, BERT’s strengths continue to shine. It’s like a trusty tool in the NLP toolbox—its pre-trained models make it easy for developers to get started without needing massive computing power. Plus, many new models, like RoBERTa and DistilBERT, build on the concepts BERT introduced.
BERT has also been integrated into popular libraries and frameworks, making it accessible for a wide range of projects.
While the field is buzzing with innovations and newer models, BERT’s foundational role and ongoing relevance keep it in the spotlight.
So, whether you’re working on a fresh NLP project or exploring new techniques, BERT still holds its place as a valuable tool.
Is BERT better than GPT?
Yes, BERT and GPT are both amazing language models, but they shine in different ways. Here’s a friendly rundown of how they stack up against each other:
BERT
- Bidirectional Brilliance: BERT is like having a super-powered pair of reading glasses. It looks at text from both directions at once, which helps it really get the meaning of words in context. This makes BERT fantastic for tasks where understanding the full context is key, like figuring out sentiment or answering questions.
- Pre-trained and Fine-tuned: BERT comes pre-trained on a huge amount of text and then can be fine-tuned for specific jobs. It’s like having a knowledgeable friend who’s already read a lot of books and can help with your particular questions.
- Best For: It excels in tasks where deep understanding of text is crucial, such as text classification, named entity recognition, and question answering.
GPT
- Unidirectional Charm: GPT, on the other hand, reads text in one direction—left to right. This makes it really good at generating text that flows naturally. Think of it as a talented storyteller who can keep the narrative going smoothly.
- Text Generation Wizard: GPT’s strong suit is generating text. Whether you need it to finish a sentence, write a story, or have a conversation, GPT’s got you covered with coherent and contextually relevant text.
- Best For: It’s perfect for tasks that involve creating or completing text, like writing essays, engaging in dialogue, or generating creative content.
Summary
- BERT: Think of it as your go-to for understanding and classifying text where context is everything.
- GPT: It’s your text generator extraordinaire, ideal for crafting and continuing text with flair.
So, depending on what you need—understanding text deeply or generating it creatively—both BERT and GPT have got their own special powers!
Is BERT API free?
The BERT model is available for free through open-source libraries such as Hugging Face’s transformers, allowing you to download and use it at no cost on your own hardware or cloud services.
However, using BERT through APIs may come with varying costs depending on the provider. For example, Hugging Face offers a cloud-based API with a free tier for limited usage, but additional features or higher usage may require a paid plan.
Similarly, Google Cloud provides access to BERT through its AI and Machine Learning services, but extensive use could result in costs based on the resources utilized. Other providers may also offer BERT through their APIs, each with its own pricing structure.
Therefore, while BERT itself is free, accessing it via APIs may involve costs depending on your usage and the service you choose.
Is BERT better than Elmo?
BERT and ELMo are both powerful models for understanding language, but they have different strengths and approaches. Here’s a friendly comparison to highlight how they differ:
Here’s a friendly comparison of BERT and ELMo in table format:
Feature | BERT | ELMo |
---|---|---|
Context Understanding | Reads text bidirectionally (left-to-right and right-to-left) simultaneously, capturing rich contextual meaning | Uses bidirectional context during training but processes text unidirectionally during inference |
Pre-training | Pre-trained on a large corpus and fine-tuned for specific tasks | Pre-trained to generate contextualized word embeddings but not typically fine-tuned for specific tasks |
Performance | Achieves state-of-the-art results on many NLP benchmarks and tasks due to its comprehensive context understanding | Improved many NLP tasks by providing contextual embeddings but generally less effective on its own compared to BERT |
Use Cases | Excellent for tasks requiring deep comprehension and fine-tuning, such as question answering and text classification | Useful for enhancing other models with better word representations through contextual embeddings |
Training and Inference | Fully bidirectional approach in both training and inference, making it highly effective for understanding context | Bidirectional during training but unidirectional during inference, limiting real-time context understanding |
BERT is known for its strength and flexibility in various NLP tasks thanks to its bidirectional processing and fine-tuning abilities, whereas ELMo remains useful for creating contextual embeddings and improving different models.
Conclusion
In this guide, we covered the process of fine-tuning BERT for text classification. We started with installing the necessary libraries, then moved on to loading and tokenizing text data, getting model predictions, and ultimately fine-tuning BERT on the IMDB movie reviews dataset for sentiment analysis. We also assessed our model’s performance and showed how to make predictions on new text data.
By utilizing BERT and the Hugging Face transformers
library, we managed to create a strong text classification model with just a few steps.
This method can be adjusted for various other text classification tasks, like spam detection or topic categorization, making it a versatile tool in your NLP toolkit.
Now that you have this knowledge, you’re ready to use BERT’s capabilities for your own text classification projects, achieving high accuracy and performance in understanding and categorizing text data.
0 Comments