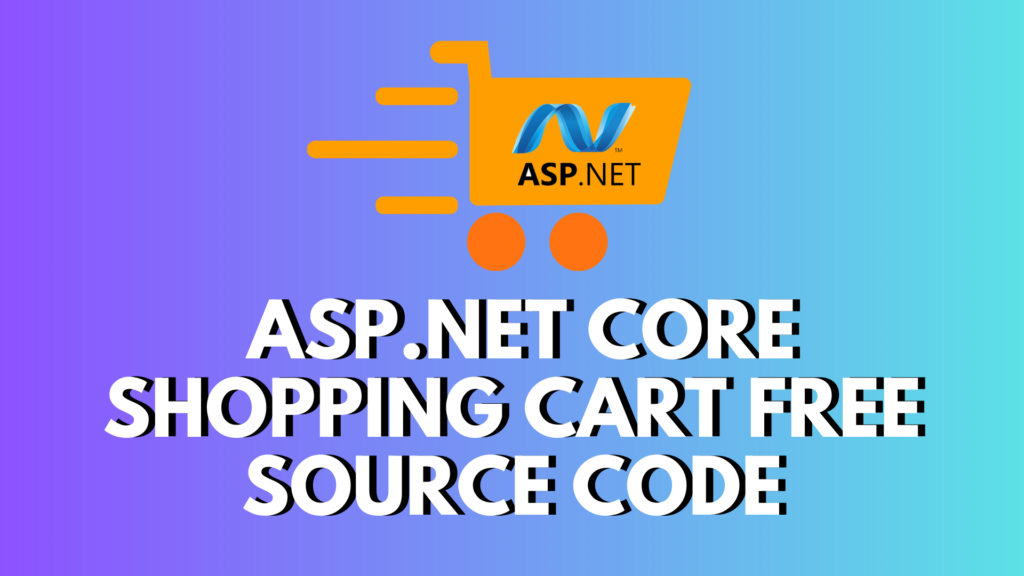
Introduction
E-commerce is a booming industry, and building a shopping cart is a crucial feature for any online store. If you’re looking to create a simple ASP NET Core shopping cart, this guide will walk you through the process. We’ll cover how to:
✅ List products dynamically ✅ Add and remove items from the cart ✅ Store cart data using session storage ✅ Build a basic REST API for cart operations
By the end of this tutorial, you’ll have a working shopping cart implemented in ASP.NET Core MVC.
Why Use ASP NET Core for an E-commerce Cart?
ASP.NET Core is a fast, scalable, and cross-platform framework ideal for building web applications. Some key benefits include:
- Performance: Faster execution with modern .NET optimizations.
- Session Management: Supports in-memory caching for cart storage.
- RESTful API Support: Easily integrates with frontend frameworks like React, Vue, or Angular.
- Security: Provides built-in authentication and authorization features.
Setting Up the ASP.NET Core Shopping Cart
1️⃣ Install Required Dependencies
First, make sure you have the latest .NET SDK installed. You can check your version by running:
dotnet --version
Create a new ASP.NET Core MVC project:
dotnet new mvc -n ShoppingCartApp
cd ShoppingCartApp
Next, install Session support to store the shopping cart data:
dotnet add package Microsoft.AspNetCore.Session
2️⃣ Configure Session in Program.cs
Open Program.cs
and modify the code to enable session support:
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddDistributedMemoryCache();
builder.Services.AddSession();
builder.Services.AddControllersWithViews();
var app = builder.Build();
app.UseSession();
app.UseRouting();
app.MapControllers();
app.Run();
3️⃣ Create the Product and Cart Models
We need a Product model to represent our store’s items:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
For managing items in the shopping cart, create a CartItem model:
public class CartItem
{
public Product Product { get; set; }
public int Quantity { get; set; }
}
4️⃣ Create the Shopping Cart Controller
Now, let’s build a CartController to handle adding and viewing cart items.
[Route("cart")]
public class CartController : Controller
{
private static List<Product> Products = new()
{
new Product { Id = 1, Name = "Laptop", Price = 999.99M },
new Product { Id = 2, Name = "Smartphone", Price = 499.99M },
new Product { Id = 3, Name = "Headphones", Price = 79.99M }
};
[HttpGet("products")]
public IActionResult GetProducts() => Ok(Products);
[HttpPost("add/{id}")]
public IActionResult AddToCart(int id)
{
var cart = HttpContext.Session.GetObjectFromJson<List<CartItem>>("Cart") ?? new List<CartItem>();
var product = Products.FirstOrDefault(p => p.Id == id);
if (product == null) return NotFound();
var cartItem = cart.FirstOrDefault(c => c.Product.Id == id);
if (cartItem != null)
cartItem.Quantity++;
else
cart.Add(new CartItem { Product = product, Quantity = 1 });
HttpContext.Session.SetObjectAsJson("Cart", cart);
return Ok(cart);
}
[HttpGet("view")]
public IActionResult ViewCart() => Ok(HttpContext.Session.GetObjectFromJson<List<CartItem>>("Cart") ?? new List<CartItem>());
}
5️⃣ Implement Session Extensions for Object Storage
Since sessions only support storing strings, we need to serialize and deserialize objects.
public static class SessionExtensions
{
public static void SetObjectAsJson(this ISession session, string key, object value)
{
session.SetString(key, System.Text.Json.JsonSerializer.Serialize(value));
}
public static T GetObjectFromJson<T>(this ISession session, string key)
{
var value = session.GetString(key);
return value == null ? default : System.Text.Json.JsonSerializer.Deserialize<T>(value);
}
}
Running and Testing the Shopping Cart
To test the shopping cart functionality:
1️⃣ Start the application:
dotnet run
2️⃣ Open Postman or your browser and access product listings:
GET http://localhost:5000/cart/products
3️⃣ Add a product to the cart:
POST http://localhost:5000/cart/add/1
4️⃣ View the cart:
GET http://localhost:5000/cart/view
Enhancing Your Shopping Cart
Here are some ideas to expand your cart system:
🚀 Add a Database: Use Entity Framework Core for persistent storage.
🛒 Integrate a Frontend: Build a UI using Razor Pages or a React/Angular frontend.
💳 Payment Gateway: Connect with Stripe or PayPal for checkout.
📦 User Authentication: Implement ASP.NET Identity for user-based carts.
Conclusion
This guide covered the fundamentals of creating a shopping cart in ASP.NET Core. You now have a fully functional cart that can list products, add/remove items, and store data using sessions.
If you found this guide helpful, share it with fellow developers and leave a comment with your thoughts! 🚀
0 Comments