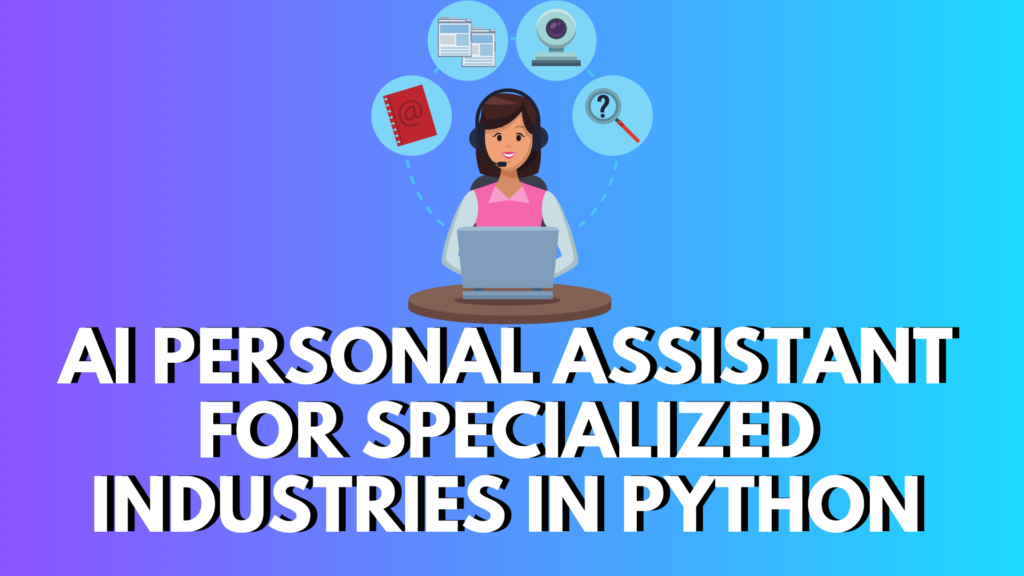
Introduction
Artificial Intelligence (AI) is revolutionizing industries by automating tasks, streamlining workflows, and enhancing user experiences. One of the most impactful applications of AI is personal assistants, which can be tailored for specialized industries such as healthcare, finance, legal services, and customer support. These AI-powered assistants can process natural language, recognize speech, answer questions, and perform specific tasks to increase efficiency and accessibility.
In this blog, we will explore how to develop an AI-powered personal assistant, focusing on the healthcare industry as an example. We will utilize Python and various AI libraries to create an intelligent assistant capable of handling speech recognition, text-to-speech responses, medical information retrieval, and appointment scheduling.
Setting Up the Development Environment
Before we start coding, we need to set up our environment with the necessary tools and libraries. Using a virtual environment is recommended to manage dependencies efficiently.
Step 1: Install Python and Set Up a Virtual Environment
Ensure you have Python installed, then create and activate a virtual environment:
python -m venv health_assistant_env
source health_assistant_env/bin/activate # On Windows: health_assistant_env\Scripts\activate
Step 2: Install Required Libraries
We will use various libraries for speech recognition, natural language processing (NLP), and web development:
pip install numpy pandas nltk transformers torch speechrecognition pyttsx3 flask
Implementing Speech Recognition and Text-to-Speech
To make the AI assistant interactive, we need to integrate voice-based input and output capabilities.
Speech Recognition
Using the speechrecognition
library, we can capture and process user voice commands:
import speech_recognition as sr
def recognize_speech():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
try:
command = recognizer.recognize_google(audio)
print(f"User said: {command}")
return command
except sr.UnknownValueError:
print("Sorry, I did not understand that.")
return None
except sr.RequestError:
print("Could not request results; check your network connection.")
return None
Text-to-Speech
Using pyttsx3
, we can enable the assistant to respond using voice:
import pyttsx3
def speak(text):
engine = pyttsx3.init()
engine.say(text)
engine.runAndWait()
Enhancing the Assistant with NLP
NLP allows the assistant to understand and process user queries effectively. We will use the transformers
library for this purpose.
from transformers import pipeline
qa_pipeline = pipeline("question-answering", model="distilbert-base-uncased-distilled-squad")
def answer_question(question, context):
response = qa_pipeline({'question': question, 'context': context})
return response['answer']
Healthcare-Specific Features
Retrieving Medical Information
To provide relevant healthcare advice, we can define a function that returns medical information based on symptoms:
def get_medical_info(symptom):
medical_database = {
"headache": "Headaches can be caused by stress, dehydration, or other factors. Stay hydrated and rest. Consult a doctor if symptoms persist.",
"fever": "A fever may indicate an infection. Stay hydrated, rest, and seek medical attention if the fever is high."
}
return medical_database.get(symptom.lower(), "I'm sorry, I don't have information on that symptom.")
Appointment Scheduling
Users should be able to schedule appointments easily:
import datetime
def schedule_appointment(date_str):
try:
appointment_date = datetime.datetime.strptime(date_str, "%Y-%m-%d")
return f"Appointment scheduled for {appointment_date.strftime('%A, %B %d, %Y')}."
except ValueError:
return "Invalid date format. Please use YYYY-MM-DD."
Developing a Web Interface with Flask
To make the assistant accessible, we will develop a web-based interface using Flask:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/ask', methods=['POST'])
def ask():
data = request.get_json()
user_input = data.get('query', '')
context = """
Headaches can be caused by stress, dehydration, or lack of sleep.
Fevers indicate infection and should be monitored closely.
Consult a healthcare professional for persistent symptoms.
"""
if 'schedule' in user_input.lower():
date_str = "2025-03-15" # Placeholder
response = schedule_appointment(date_str)
else:
response = answer_question(user_input, context)
return jsonify({'response': response})
if __name__ == '__main__':
app.run(debug=True)
Enhancements and Future Improvements
To make the assistant more robust, consider implementing:
- Sentiment Analysis: Adjust responses based on user emotions.
- Personalization: Store user preferences for a tailored experience.
- Integration with Medical Databases: Use APIs for real-time medical information.
- Security and Compliance: Implement encryption and follow HIPAA guidelines.
Conclusion
AI-powered personal assistants are transforming industries by automating interactions and improving efficiency. By integrating speech recognition, NLP, and domain-specific features, we created a healthcare-focused assistant capable of answering medical queries and scheduling appointments. Future enhancements can further improve its accuracy, usability, and security, making it an indispensable tool in specialized industries.
By following the steps outlined in this guide, you can develop and deploy a powerful AI assistant tailored to your specific industry needs.
FAQ
- How to make a personal AI assistant in Python?
You can create a personal AI assistant in Python by using libraries such asspeechrecognition
for voice input,pyttsx3
for text-to-speech, andtransformers
for natural language processing. Additionally, frameworks like Flask can be used to develop a web-based interface for your assistant. - Can I create my own AI using Python?
Yes, Python is one of the best languages for AI development. You can use machine learning libraries like TensorFlow, PyTorch, and Scikit-learn to build your own AI model for tasks such as voice recognition, chatbot interactions, and decision-making. - Can AI be a personal assistant?
Yes, AI can function as a personal assistant by automating tasks such as setting reminders, answering questions, managing schedules, and even providing recommendations based on user preferences. - Can I make my own personal AI assistant?
Absolutely! With the right programming skills and tools, you can develop your own personal AI assistant tailored to your needs. You can integrate voice recognition, natural language processing, and automation features to make it intelligent and useful. - Can I create my own AI voice?
Yes, you can create your own AI voice using Python libraries such aspyttsx3
for text-to-speech or more advanced models like OpenAI’s TTS, Google’s Text-to-Speech, or ElevenLabs. If you want to train your own AI voice, you can use deep learning frameworks like TensorFlow or Tacotron 2 for high-quality voice synthesis. - Can I make my own AI chatbot?
Absolutely! You can create an AI chatbot using Python with libraries such astransformers
(for natural language processing),ChatterBot
(for conversational AI), or by integrating OpenAI’s GPT models through APIs. A chatbot can be deployed on a website, mobile app, or even messaging platforms like WhatsApp or Telegram. - Can you create a self-learning AI?
Yes, a self-learning AI can be built using machine learning techniques, particularly reinforcement learning or unsupervised learning. You can use libraries like TensorFlow, PyTorch, or Scikit-learn to develop an AI model that learns from data and improves over time without explicit programming. - How to make a personal assistant with ChatGPT?
You can create a personal assistant using ChatGPT by integrating OpenAI’s API with a Python-based application. Using frameworks like Flask or FastAPI, you can create a web or voice assistant that takes user input, sends it to ChatGPT for processing, and returns a response. Additional features like speech recognition (speechrecognition
library) and text-to-speech (pyttsx3
) can make it more interactive.
1 Comment
How Do I Create A CMS System In Python 2025 Powerful · February 27, 2025 at 10:45 am
[…] Best AI Personal Assistant Using Python 2025 […]