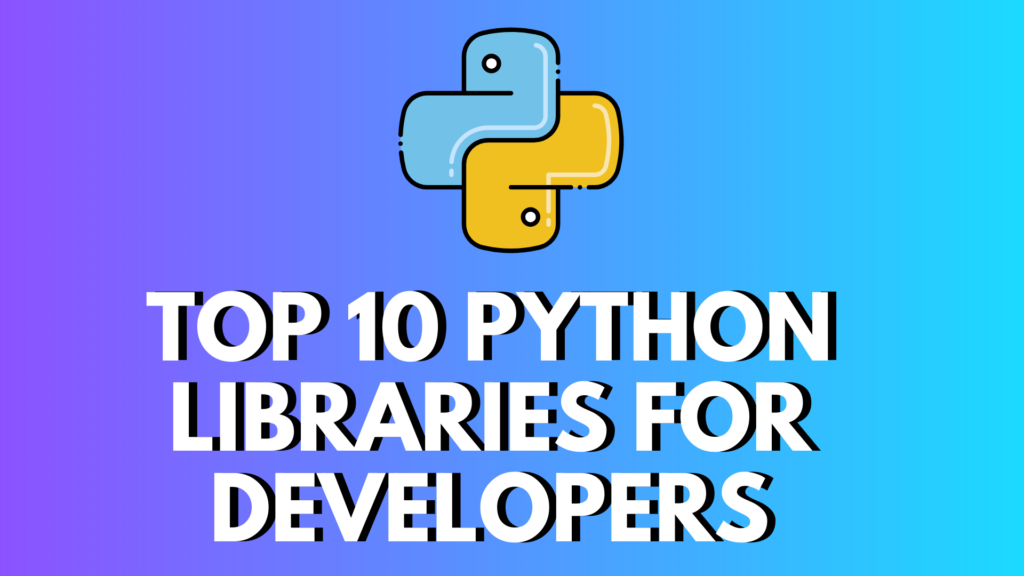
Introductionto Python Libraries
Python is one of the most popular programming languages, thanks to its simplicity, versatility, and vast ecosystem of libraries.
Whether you’re working on data science, machine learning, web development, or automation, Python has powerful libraries that make coding more efficient. In this article, we’ll explore the top 15 Python libraries for developers, their use cases, and code examples to help you get started.
1. NumPy
Use Case: Numerical computing and handling large multi-dimensional arrays.
NumPy is essential for scientific computing in Python. It provides support for large matrices, high-level mathematical functions, and efficient array operations.
Example: Using NumPy for Array Operations
import numpy as np
# Creating an array
arr = np.array([1, 2, 3, 4, 5])
print("Original Array:", arr)
# Performing mathematical operations
print("Squared Array:", np.square(arr))
print("Mean of Array:", np.mean(arr))
2. Pandas
Use Case: Data manipulation and analysis.
Pandas is widely used for working with structured data, such as CSV files and databases. It provides powerful tools for cleaning, analyzing, and visualizing data.
Example: Reading and Analyzing Data with Pandas
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print("DataFrame:")
print(df)
# Reading data from a CSV file
df = pd.read_csv("data.csv")
print("First 5 Rows:")
print(df.head())
3. Matplotlib
Use Case: Data visualization and plotting graphs.
Matplotlib allows developers to create high-quality visualizations, including line plots, bar charts, scatter plots, and histograms.
Example: Creating a Simple Line Plot
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [10, 20, 25, 30, 40]
# Creating a line plot
plt.plot(x, y, marker='o', linestyle='--', color='b')
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.title("Simple Line Plot")
plt.show()
4. Seaborn
Use Case: Advanced data visualization.
Seaborn is built on top of Matplotlib and provides a high-level interface for drawing attractive statistical graphics.
Example: Creating a Scatter Plot
import seaborn as sns
import matplotlib.pyplot as plt
# Sample data
data = sns.load_dataset("iris")
# Creating a scatter plot
sns.scatterplot(x="sepal_length", y="sepal_width", hue="species", data=data)
plt.show()
5. Scikit-Learn
Use Case: Machine learning and predictive modeling.
Scikit-Learn provides tools for building and evaluating machine learning models, including regression, classification, and clustering.
Example: Creating a Simple Linear Regression Model
from sklearn.linear_model import LinearRegression
import numpy as np
# Sample data
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
# Creating and training the model
model = LinearRegression()
model.fit(X, y)
# Making predictions
predictions = model.predict([[6]])
print("Predicted Value for X=6:", predictions[0])
6. TensorFlow
Use Case: Deep learning and neural networks.
TensorFlow is an open-source library developed by Google for building and training deep learning models.
Example: Creating a Basic Neural Network
import tensorflow as tf
# Creating a simple neural network model
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
print("Neural Network Model Summary:")
model.summary()
7. OpenCV
Use Case: Computer vision and image processing.
OpenCV is a powerful library for image processing and computer vision applications.
Example: Detecting Faces in an Image
import cv2
# Load an image
img = cv2.imread("face.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Load the Haar cascade classifier
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml")
faces = face_cascade.detectMultiScale(gray, 1.1, 4)
# Draw rectangles around detected faces
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x + w, y + h), (255, 0, 0), 2)
cv2.imshow("Face Detection", img)
cv2.waitKey(0)
8. Flask
Use Case: Web development and APIs.
Flask is a lightweight web framework that helps developers build web applications and RESTful APIs quickly.
Example: Creating a Simple Flask Web App
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
9. Requests
Use Case: Handling HTTP requests.
Requests is a simple yet powerful library for making HTTP requests in Python.
Example: Fetching Data from a URL
import requests
response = requests.get("https://api.github.com")
print(response.json())
10. BeautifulSoup
Use Case: Web scraping.
BeautifulSoup is used for extracting data from HTML and XML documents.
Example: Extracting Titles from a Webpage
from bs4 import BeautifulSoup
import requests
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
print("Page Title:", soup.title.string)
Conclusion
Python’s extensive library ecosystem makes it a powerful tool for developers across various domains. Whether you’re working on data analysis, machine learning, web development, or automation, these libraries will help you code efficiently and effectively.
Call to Action:
✅ Explore More: Check out our guides on Advanced Python Projects and Python for Data Science.
📢 Which Python library is your favorite? Let us know in the comments below!
1 Comment
21 Python Mini Projects With Code|Best Python For Beginners · March 12, 2025 at 10:16 am
[…] Top Python Libraries for Developers […]